Operators in Javascript
Operators in JavaScript are used to perform operations on variables and values. They are essential for performing tasks like mathematical calculations, comparisons, and logical operations, making them fundamental to control flow and data manipulation.
Lets Go!
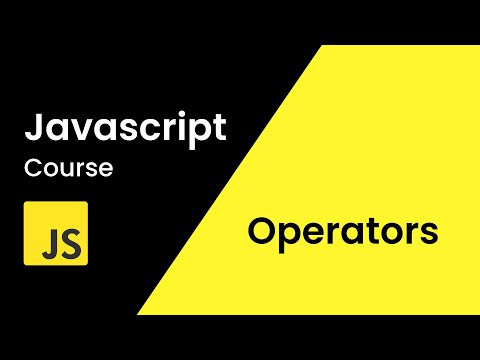
Operators in Javascript
Level 2
Operators in JavaScript are used to perform operations on variables and values. They are essential for performing tasks like mathematical calculations, comparisons, and logical operations, making them fundamental to control flow and data manipulation.
Get Started 🍁Operators in Javascript
Operators in JavaScript are used to perform operations on variables and values. They are essential for performing tasks like mathematical calculations, comparisons, and logical operations, making them fundamental to control flow and data manipulation.
Introduction to JavaScript Operators
Welcome to the course "Introduction to JavaScript Operators"! In this course, we will delve into the world of operators in JavaScript, which are vital symbols used to perform operations on values and variables.
Course Overview:
Throughout this course, we will explore various types of operators, including:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- String operators
We will cover essential concepts such as operator precedence and associativity, preparing you to navigate and utilize operators effectively in JavaScript programming.
Are you ready to uncover the power of JavaScript operators and enhance your coding skills?
Let's embark on this exciting journey together! If you have any questions, feel free to ask in the comment section. Don't forget to like, share, and subscribe to stay updated on more informative content. Thank you for joining us! 🚀🌟
Main Concepts of JavaScript Operators
-
Operators in JavaScript: Operators are symbols used to perform operations on values and variables in JavaScript.
-
Arithmetic Operators: Arithmetic operators are used to perform mathematical operations such as addition, subtraction, multiplication, division, modulus, and exponentiation.
-
Assignment Operators: Assignment operators are used to assign values to variables, with examples including simple assignment, addition assignment, subtraction assignment, multiplication assignment, division assignment, modulus assignment, and exponentiation assignment.
-
Increment and Decrement Operators: Increment (++) and decrement (--) operators are used to increase or decrease the value of a variable by 1, with both prefix and postfix forms.
-
Comparison Operators: Comparison operators compare two values and return a Boolean value (true or false). Examples include less than, greater than, less than or equal to, greater than or equal to, equal to, not equal, strict equality check, and strict inequality check.
-
Logical Operators: Logical operators (AND, OR, NOT) perform logical operations based on the truth values of operands. Logical AND returns true only if all operands are true, logical OR returns true if at least one operand is true, and logical NOT returns the flipped value of the operand.
-
String Operators: The plus (+) operator in JavaScript is used to concatenate or join two or more strings, allowing for the creation of longer strings by combining smaller ones.
-
Operator Precedence: Operator precedence in JavaScript determines the order in which operators are evaluated in mathematical expressions, influencing the outcome of calculations based on the priority of operators.
-
Operator Associativity: Operator associativity in JavaScript defines the order in which operators of the same precedence are evaluated, with examples of left-to-right associativity and right-to-left associativity explained.
Practical Applications of JavaScript Operators
Step-by-Step Guide
-
Arithmetic Operators:
- Addition: Try
let sum = 5 + 3; console.log(sum);
to see the result. - Subtraction: Test
X = 5 - 3; console.log(X);
to see the output. - Multiplication: Use
5 * 3;
to display the result of 5 multiplied by 3. - Division: Experiment with
15 / 3;
to get the division result. - Modulus: Test
17 % 4;
to see the modulus value. - Exponentiation: Calculate
2 ** 4;
to see the exponentiation result.
- Addition: Try
-
Assignment Operators:
- Simple Assignment: Try
let x = 5;
to assign a value to a variable. - Addition Assignment: Use
x += 3; console.log(x);
to increment the existing value ofx
by 3. - Subtraction, Multiplication, Division, Modulus, and Exponentiation Assignment: Experiment with these operators similarly.
- Simple Assignment: Try
-
Increment and Decrement Operators:
- Prefix Increment and Decrement: Test
let a = 10; console.log(++a); console.log(a);
to see the prefix increment in action. - Postfix Increment and Decrement: Experiment with
let a = 10; console.log(a++); console.log(a);
to observe the postfix increment at work.
- Prefix Increment and Decrement: Test
-
Comparison Operators:
- Less Than, Greater Than, Less Than or Equal To, Greater Than or Equal To: Try out various comparison operators with numbers or strings for practice.
- Equality Checks: Experiment with
a == b
anda === b
to see the difference in strict equality checks.
-
Logical Operators:
- Logical AND: Test logical AND operations with
&&
to understand how it works. - Logical OR: Experiment with logical OR operations using
||
to see how it evaluates operands. - Logical NOT: Try
let s = true; let no = false; console.log(!s); console.log(!no);
to see the flipped Boolean values.
- Logical AND: Test logical AND operations with
-
String Operators:
- Concatenation: Use the
+
operator to join strings together, likeconsole.log('Hello' + ' World');
.
- Concatenation: Use the
-
Operator Precedence and Associativity:
- Operator Precedence: Understand the order in which operators are evaluated, such as multiplication before addition.
- Operator Associativity: Learn how operators of the same precedence are evaluated, either left to right or right to left.
Actionable Tasks
- Choose any of the above practical applications.
- Try out the code snippets in your preferred JavaScript environment.
- Experiment with different values and operands to see how the operators behave.
- Share your findings or ask questions in the comments for further clarification.
Test your Knowledge
Which operator is used to perform division in JavaScript?
Which operator is used to perform division in JavaScript?
Advanced Insights into JavaScript Operators
JavaScript operators play a crucial role in performing various operations on operands. Let's delve deeper into some advanced aspects and insights of JavaScript operators to enhance your understanding:
Tips and Recommendations:
- When working with arithmetic operators, ensure you are familiar with their functionality and how they interact with operands.
- Experiment with different assignment operators to understand how they update and modify variable values efficiently.
- Practice using increment and decrement operators in both prefix and postfix forms to grasp their impact on variable values.
Expert Advice:
- Logical operators are vital for decision-making and loop programming in JavaScript. Understanding how && (logical AND), || (logical OR), and ! (logical NOT) operate can enhance your code logic.
- Consider operator precedence while coding to ensure correct operation execution order, especially when combining multiple operators in an expression.
Curiosity Question:
How can you leverage bitwise operators in JavaScript to optimize performance in specific scenarios?
By exploring these advanced insights into JavaScript operators, you can elevate your programming skills and problem-solving approach. Happy coding!
Additional Resources for JavaScript Operators
- MDN Web Docs: Expressions and Operators: A comprehensive guide to JavaScript expressions and operators by MDN Web Docs.
- JavaScript Arithmetic Operators: W3Schools tutorial on arithmetic operators in JavaScript.
- JavaScript Assignment Operators: GeeksforGeeks article on assignment operators in JavaScript.
- JavaScript Comparison Operators: The comparison operator section from JavaScript.info.
- JavaScript Logical Operators: Tutorial on logical operators in JavaScript from Tutorialspoint.
- JavaScript String Operators: String manipulation functions in JavaScript for further exploration.
- Operator Precedence and Associativity in JavaScript: An in-depth article on operator precedence and associativity in JavaScript.
Explore these resources to deepen your understanding of JavaScript operators and enhance your programming skills! 🚀
Practice
Task
Task: Create a program that checks if a number is even or odd using the modulus operator.
Task: Write a function that takes two numbers and returns the result of their multiplication.
Task: Develop a simple comparison program that checks if two strings are equal using ===
.
Task: Use logical operators to create a program that checks if a user is both older than 18 and a registered member.
Task: Write a program to calculate the percentage of a score based on the total and display a message accordingly.
Task: Implement a basic calculator using the four main arithmetic operators.
Task: Write a function that takes three numbers and returns the highest value.
Task: Create a program to check if a person is eligible for a senior citizen discount based on age.
Task: Implement a function that determines whether a given year is a leap year.
Task: Use the !==
operator to create a program that checks if two variables are not equal.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
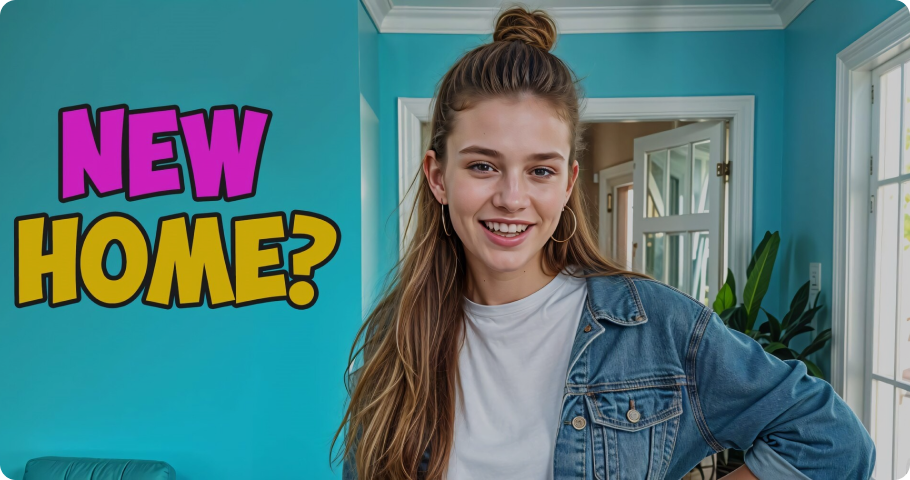
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.