Control Flow (if, else, switch)
Control flow statements allow you to dictate the flow of your program based on certain conditions. They are essential for decision-making, and they include `if`, `else`, and `switch` statements.
Lets Go!
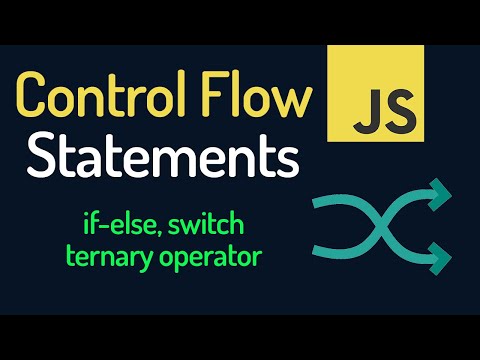
Control Flow (if, else, switch)
Level 3
Control flow statements allow you to dictate the flow of your program based on certain conditions. They are essential for decision-making, and they include `if`, `else`, and `switch` statements.
Get Started 🍁Control Flow (if, else, switch)
Control flow statements allow you to dictate the flow of your program based on certain conditions. They are essential for decision-making, and they include `if`, `else`, and `switch` statements.
Introduction to JavaScript Control Flow Statements
Welcome to the world of JavaScript programming! In this beginner's tutorial course by Coder Fiction, we will dive deep into understanding control flow statements in JavaScript. Control flow statements are essential for any programmer as they allow you to control the flow of program execution based on certain conditions.
Throughout this course, we will cover key topics such as if
statements, if-else
statements, the ternary operator, and the switch statement.
Have you ever wondered how to make your programs execute different blocks of code based on specific conditions? Join us in this course to learn how to make your JavaScript programs more dynamic and flexible by incorporating control flow statements.
Let's embark on this exciting journey of mastering JavaScript's control flow statements together and unlock the potential of your coding skills. Are you ready to take your programming knowledge to the next level? Let's get started!
Main Concepts of JavaScript Control Flow Statements
-
If Statement:
- The
if
statement executes a block of code if a specified condition is true. - It is written as
if (condition) { // code block }
. - If the condition is evaluated to true, the code block inside the curly braces will be executed.
- The
-
If-Else Statement:
- The
if-else
statement provides an alternative execution path if the condition in theif
statement is false. - It is written as
if (condition) { // code block } else { // alternative code block }
. - If the condition in the
if
statement is false, the code block inside theelse
clause will be executed.
- The
-
Else If Statement:
- The
else if
statement allows the program to check multiple conditions sequentially. - It is written as
if (condition1) { // code block } else if (condition2) { // code block }
. - If
condition1
is false, it moves tocondition2
and executes the corresponding code block if true.
- The
-
Ternary Operator:
- The ternary operator is a shorthand syntax for an
if-else
statement. - It is written as
condition ? expression1 : expression2
. - If the condition is true, it returns
expression1
; otherwise, it returnsexpression2
.
- The ternary operator is a shorthand syntax for an
-
Switch Statement:
- The
switch
statement evaluates an expression and executes the corresponding code block based on matching cases. - It is structured as
switch (expression) { case label1: // code block break; case label2: // code block break; default: // code block }
. - If a case matches the expression, the code block associated with that case is executed.
- The
These concepts are essential for controlling the flow of program execution based on specific conditions, allowing for more dynamic and flexible programming in JavaScript.
Practical Applications of Control Flow Statements in JavaScript
Step-by-Step Guide:
-
Checking if a Number is Even or Odd:
- Declare a variable
num
and initialize it with a number. - Use an
if
statement with a modulus operation to check if the number is even. - Print appropriate messages for even and odd numbers.
- Example:
let num = 5; if (num % 2 === 0) { console.log(`${num} is an even number`); } else { console.log(`${num} is an odd number`); }
- Declare a variable
-
Finding the Biggest Number from Three Numbers:
- Declare three variables and initialize them with numbers.
- Use an
if-else if-else
statement to find the biggest number. - Print the result based on the comparisons.
- Example:
let a = 10; let b = 20; let c = 15; if (a >= b && a >= c) { console.log(`a is the biggest number`); } else if (b >= a && b >= c) { console.log(`b is the biggest number`); } else { console.log(`c is the biggest number`); }
-
Using Ternary Operator to Check Student Exam Result:
- Declare a variable with the score.
- Use a ternary operator to check if the student passed.
- Print the result based on the condition.
- Example:
let score = 75; let result = score > 45 ? 'passed' : 'failed'; console.log(`Student ${result} the exam`);
-
Identifying Country Names by Code using Switch Statement:
- Declare a variable with a country code.
- Use a
switch
statement withcase
labels for different countries. - Print the corresponding country name based on the code.
- Example:
let countryCode = 'ind'; switch (countryCode) { case 'usa': console.log('United States of America'); break; case 'ind': console.log('India'); break; case 'sa': console.log('South Africa'); break; case 'ca': console.log('Canada'); break; default: console.log('Country not found'); }
Try these examples in your JavaScript environment to understand the practical applications of control flow statements. Play around with different values to see how the flow changes based on the conditions provided. Happy coding!
Test your Knowledge
Which statement is used to execute code based on multiple conditions?
Which statement is used to execute code based on multiple conditions?
Advanced Insights into JavaScript Control Flow Statements
In JavaScript, control flow statements play a crucial role in managing the flow of program execution based on specific conditions. Let's delve deeper into some advanced aspects of control flow in JavaScript.
Chaining Else-If Statements for Complex Conditions
When dealing with more complex conditions, you can chain multiple else-if statements to cater to various scenarios. Remember that the order of these conditions matters, as they are evaluated sequentially. Starting from the first condition, if it evaluates to false, the next condition in line is checked. This iterative process continues until a true condition is found, or the final else block is executed.
Curiosity Question: How can you optimize the order of else-if conditions to ensure efficient execution when dealing with complex scenarios?
Leveraging Ternary Operator for Concise Statements
The ternary operator in JavaScript offers a concise way to implement conditional statements as shorthand for if-else blocks. It consists of a condition followed by a question mark and two expressions separated by a colon. This operator is particularly useful for short, straightforward conditions where you want to assign a value based on a logical condition.
Tip: Consider using the ternary operator for compact and readable code in scenarios with simple conditional assignments.
Understanding the Behavior of Switch Statements
Switch statements provide a structured way to handle multiple selections based on an expression's value. Each case block contains statements to execute if the expression matches the specified value. It's crucial to include break statements after each case block to prevent fall-through behavior, where subsequent case blocks are executed irrespective of matching conditions.
Expert Advice: Utilize switch statements for scenarios involving multiple distinct cases to enhance code readability and maintainability.
By exploring these advanced insights into JavaScript control flow statements, you can enhance your programming skills and efficiently manage program execution based on different conditions. Experiment with various scenarios to deepen your understanding and proficiency in implementing control flow in JavaScript.
Additional Resources for JavaScript Control Flow
- MDN Web Docs: Control flow and error handling
- JavaScript Control Statements
- Understanding JavaScript Control Flow: Understanding Control Flow
Explore these resources to deepen your understanding of JavaScript control flow statements and enhance your programming skills!
Practice
Task
Task: Create a program that checks if a number is positive, negative, or zero using if/else
.
Task: Write a program that prints 'Even' or 'Odd' based on a given number using the ternary operator.
Task: Create a switch
statement that checks a user's grade and returns a message based on the grade.
Task: Write a program that checks if a person is eligible to vote (age >= 18) using if/else
.
Task: Use a switch
statement to handle different user roles like 'admin', 'moderator', and 'guest'.
Task: Implement a program that checks if a number is divisible by both 3 and 5.
Task: Write a program that prints 'Good Morning' if the time is before noon, and 'Good Evening' otherwise.
Task: Implement a program that checks if a user is logged in and has administrator privileges.
Task: Create a program that checks if a year is a leap year or not using if/else
.
Task: Write a function that returns 'Adult' or 'Minor' based on the user's age.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
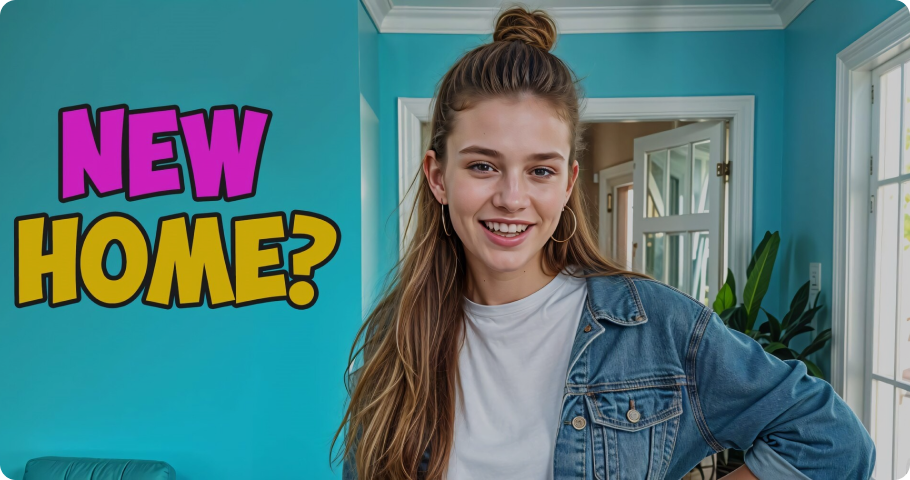
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.