Loops (for, while, do-while)
Loops in JavaScript allow you to execute a block of code multiple times, based on a condition. They are essential for iterating over arrays and performing repetitive tasks efficiently.
Lets Go!
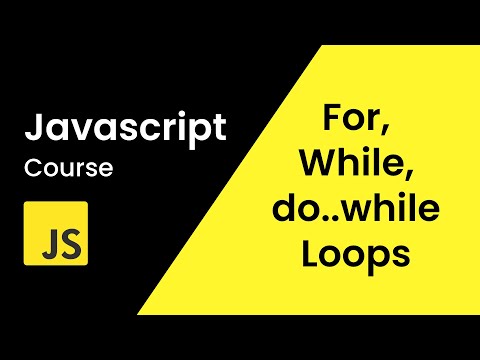
Loops (for, while, do-while)
Level 5
Loops in JavaScript allow you to execute a block of code multiple times, based on a condition. They are essential for iterating over arrays and performing repetitive tasks efficiently.
Get Started 🍁Loops (for, while, do-while)
Loops in JavaScript allow you to execute a block of code multiple times, based on a condition. They are essential for iterating over arrays and performing repetitive tasks efficiently.
Introduction to Loops in JavaScript Programming
Welcome to our course on Loops in JavaScript programming! In this course, we will delve into the fundamental concepts of loops, a crucial component in programming that allows you to repeat a block of code multiple times.
Loops are essential for tasks such as displaying messages, iterating through arrays, and handling repetitive operations efficiently. Whether you are a beginner or looking to deepen your understanding of loops in JavaScript, this course will provide you with a solid foundation.
What You Will Learn
- Syntax and usage of the for loop
- Iterating through arrays using loops
- Creating nested loops for complex operations
- Exploring the while and do while loop structures
- Implementing break and continue statements to control loop execution
By the end of this course, you will feel confident in your ability to leverage loops effectively in JavaScript programming. Are you ready to explore the power of loops in programming? Let's dive in!
Curiosity Question
How can loops streamline the process of printing a message multiple times without repetitive code?
Join us on this exciting journey into the world of loops in JavaScript programming. Let's get started! 🚀
Main Concepts of Loops in JavaScript
-
For Loop
- A for loop is used to repeat a block of code a specified number of times.
- Syntax:
for (initial value of i; condition; increase i) { // code to be executed }
- Example: Printing a message multiple times or iterating through an array.
-
While Loop
- A while loop repeats a block of code while a specified condition is true.
- Syntax:
while (condition) { // code to be repeated }
- Example: Printing numbers from 0 to 10 using a while loop.
-
Do-While Loop
- A do-while loop is similar to a while loop, but it will always execute the block of code at least once before checking the condition.
- Syntax:
do { // code to be executed } while (condition);
- Example: Printing numbers from 1 to 5 using a do-while loop.
-
Break Statement
- The break statement is used to terminate a loop immediately.
- Example: Terminating a loop when a specific condition is met, such as ending the loop when i reaches 3 in a for loop.
-
Continue Statement
- The continue statement is used to skip the current iteration of a loop and move to the next iteration.
- Example: Skipping a specific iteration, like not printing a number when i is equal to 3 in a for loop.
By understanding these concepts, you can effectively control the flow of your JavaScript code and perform repetitive tasks efficiently using loops.
Practical Applications of JavaScript Loops
Step-by-Step Guide:
-
Printing a Message Multiple Times with a
for
Loop:- Start by setting an initial value of
i
to 1. - Use a
for
loop with the syntax:for(let i = 1; i <= 10; i++) { console.log("Great Stack"); }
- Run the code to see "Great Stack" printed 10 times.
- Start by setting an initial value of
-
Printing Numbers from 1 to 10 with a
for
Loop:- Use a
for
loop with the syntax:for(let i = 1; i <= 10; i++) { console.log(i); }
- Execute the code to display numbers from 1 to 10 in the console.
- Use a
-
Iterating Through an Array with a
for
Loop:- Declare an array, for example:
let coding = ["JavaScript", "Python", "C++"];
- Utilize a
for
loop to print each element:for(let i = 0; i < coding.length; i++) { console.log(coding[i]); }
- Run the code to output "JavaScript", "Python", and "C++" separately.
- Declare an array, for example:
-
Nested Loops with
for
Loop:- Create a nested loop scenario:
for(let i = 1; i <= 5; i++) { console.log(i); for(let j = 1; j <= 3; j++) { console.log("Inner Loop " + j); } }
- Execute the code to see the sequence of numbers and inner loop messages.
- Create a nested loop scenario:
-
Repeating Output with a
while
Loop:- Set an initial value of
i
to 0. - Use a
while
loop to print numbers from 0 to 10:let i = 0; while(i <= 10) { console.log(i); i++; }
- Run the code to display numbers from 0 to 10 in the console.
- Set an initial value of
-
Printing Numbers using a
do while
Loop:- Implement a
do while
loop to print numbers from 1 to 5:let i = 1; do { console.log(i); i++; } while(i <= 5);
- Execute the code to output numbers 1 to 5.
- Implement a
Try It Out:
- Copy and paste the provided code snippets into your JavaScript environment.
- Run the code to observe the results in the console.
- Experiment with tweaking the values and logic to further understand JavaScript loops.
Test your Knowledge
Which loop is best used when the number of iterations is known?
Which loop is best used when the number of iterations is known?
Advanced Insights into Loops in JavaScript
In this section, we will delve into advanced aspects of loops in JavaScript to enhance your programming skills.
Nested Loops
Nested loops involve a loop inside another loop, allowing for more complex iterations. By nesting loops, you can create intricate patterns or perform operations on multidimensional arrays efficiently. For example, you can print a matrix or traverse a two-dimensional array comprehensively.
Expert Tip: When using nested loops, be mindful of the order of execution and ensure clarity in your code structure to avoid confusion.
Curiosity Question: How might you optimize nested loops to minimize time complexity in algorithmic problems?
Advanced Loop Control
Understanding the break
and continue
statements is crucial for precise control over loop execution. The break
statement enables you to exit a loop prematurely, while the continue
statement allows you to skip the current iteration and move to the next one.
Recommendation: Employ break
and continue
judiciously to tailor the loop behavior to your specific requirements.
Curiosity Question: Can you think of real-world scenarios where the strategic use of break
or continue
can streamline program logic effectively?
Iterating over Arrays
Loops are indispensable for traversing arrays and performing operations on each element. Whether using a for
loop to iterate over elements sequentially or leveraging for...of
or forEach
for a more concise syntax, mastering array iteration methods is fundamental for manipulating data efficiently.
Tip: Choose the appropriate loop iteration method based on your task requirements and maintain code readability.
Curiosity Question: How can you optimize array traversal using built-in array methods like map
, filter
, or reduce
in JavaScript?
By exploring these advanced insights into loops in JavaScript, you can elevate your programming proficiency and tackle complex tasks with confidence. Experiment with different loop structures and control statements to enhance your problem-solving skills and code efficiency. Happy coding! 🚀
Additional Resources for Loops in JavaScript
- Article: A Comprehensive Guide to JavaScript Loops
- Video Tutorial: Mastering Loops in JavaScript
- Book: "JavaScript for Beginners" by Emily Thomson - Chapter 5 covers in-depth information on loops
- Online Course: JavaScript Loops and Iteration
- GitHub Repository: Examples of JavaScript Loops
Feel free to explore these resources to enhance your understanding of loops in JavaScript. Happy coding! 🚀
Practice
Task
Task: Use a for
loop to sum all values in an array.
Task: Write a program that prints numbers from 1 to 10 using a while
loop.
Task: Create a program that finds the smallest number in an array using a loop.
Task: Write a program that displays the multiplication table of 5 using a loop.
Task: Use a for...of
loop to iterate over an array of strings and log each element.
Task: Implement a do...while
loop that asks the user for input until they provide a valid response.
Task: Write a program that counts the occurrences of a specific value in an array.
Task: Create a program that prints out the odd numbers between 1 and 50.
Task: Use a for...in
loop to iterate through the keys of an object.
Task: Write a program that prints a countdown from 10 to 1.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
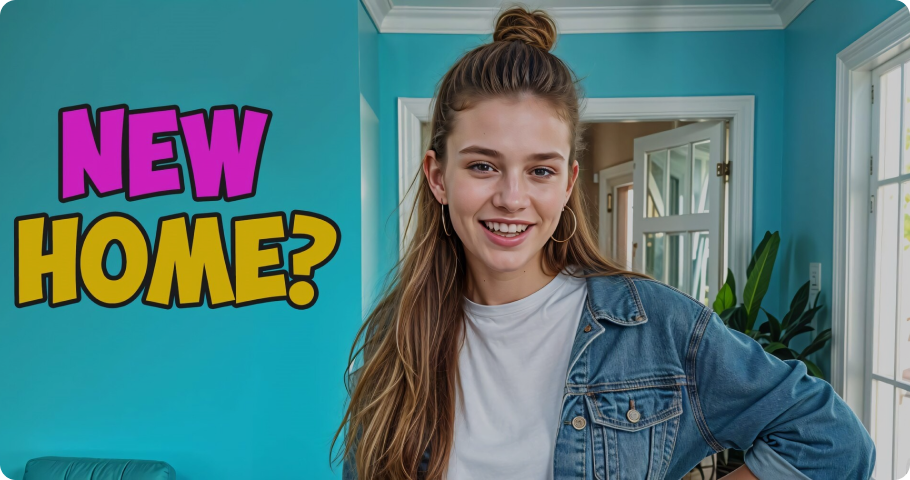
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.