Arrays (Methods, Iteration)
Arrays in JavaScript allow you to store multiple values in a single variable. They are essential for working with lists of data, and JavaScript provides powerful methods to manipulate them.
Lets Go!
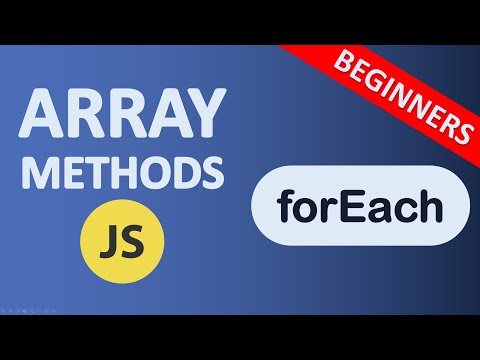
Arrays (Methods, Iteration)
Lesson 6
Learn to manipulate arrays, access elements, and use array methods effectively in JavaScript.
Get Started 🍁Introduction to JavaScript Arrays and Iteration Techniques
Welcome to "Introduction to JavaScript Arrays and Iteration Techniques"! In this course, we will explore one of the most fundamental array methods in JavaScript - the forEach method.
If you are new to JavaScript or looking to enhance your understanding of array manipulation, this course is perfect for you. We will start by diving into the basics of iterating through arrays using a simple for loop, understanding index management, and element traversal.
Have you ever wondered how you can streamline your array iterations without the hassle of manually setting index values and loop conditions? The forEach method is here to revolutionize your approach to array processing!
Throughout this course, we will cover essential concepts like callback functions, inline functions, arrow functions, and the magical "this" keyword in JavaScript. By the end of the course, you will grasp the nuances of forEach and other array methods like every, some, find, and find index.
Join us on this exciting journey through the world of JavaScript arrays and iteration techniques. Let's break free from traditional loops and embrace the efficiency and elegance of forEach! Don't forget to like, comment, share, and subscribe for more enriching content. See you in the course!
Main Concepts of Array Methods in JavaScript
-
Introduction to For Each Method: The
forEach
method is one of the most commonly used array methods in JavaScript. It allows you to iterate over each element in an array without the need for a traditionalfor
loop. -
Iterating an Array using a For Loop: Initially, understanding how a simple
for
loop works can help grasp the concept of iteration. By setting the initial index, defining the stopping condition, and incrementing the index, you can iterate through an array manually. -
Creating a Function for Logging Elements: To simplify the logging process, you can encapsulate the code inside a function. This function can log both the index and the element of the array, making the code cleaner and more maintainable.
-
Using For Each Method: The
forEach
method eliminates the need for manually setting up an iteration process by calling a function for each element in the array. It takes a callback function as an argument, which is executed on each element of the array. -
Understanding Callback Functions: The function passed as a callback in the
forEach
method is known as a callback function. It is essential to follow the order of parameters, starting with the element, followed by the index. -
In-line Functions and Arrow Functions: In addition to passing a separate function, you can use in-line functions or arrow functions directly within the
forEach
method, providing a more concise way to iterate over an array. -
Limitation of Break Statement: Unlike traditional
for
loops, theforEach
method does not support using abreak
statement to exit the loop prematurely. Therefore, if breaking out of a loop is required, a standardfor
loop should be used instead. -
Alternative Array Methods for Loop Breaking: To overcome the limitation of the
forEach
method not permitting the use of abreak
statement, alternative array methods likeevery
,some
,find
, andfindIndex
can be utilized to achieve similar functionalities. -
Additional Parameters in For Each Method: Apart from the callback function,
forEach
can accept a third parameter representing the array itself. Additionally, a second parameter calledthisArg
can be included to specify the context for thethis
keyword within the callback function. -
Manipulating the
this
Keyword: Understanding how thethis
keyword works within functions is crucial. By providing an object as the second argument (thisArg
) in theforEach
method, you can control the context in whichthis
refers, allowing flexibility in accessing different objects within the iterations.
Practical Applications of For Each Method in JavaScript
Let's dive into how you can practically apply the forEach
method in JavaScript to iterate over arrays efficiently. Follow these steps to implement it yourself:
-
Basic Usage of
forEach
:- Start by creating an array, for example
letters
, with elements likea
,b
, `c. - Define a function, for example
log
, that logs the index and element of the array. - Use the
forEach
method on the array and pass in thelog
function as a callback. - Run the code and observe the index and elements being logged for each iteration.
- Start by creating an array, for example
-
Using Inline Functions:
- Instead of defining a separate function, you can use inline functions directly within the
forEach
method. - Create an inline function that takes the element and index as parameters and logs them.
- Use this inline function directly inside the
forEach
method call. - Execute the code to see the same results without defining an external function.
- Instead of defining a separate function, you can use inline functions directly within the
-
Working with Different Parameters:
- Understand that in the callback function within
forEach
, the first parameter is always the element, the second is the index, and the third is the array itself. - Although the array parameter is not commonly used, you can leverage it if needed within your function.
- Understand that in the callback function within
-
Managing the
this
Keyword:- Explore how to handle the
this
keyword within theforEach
method. - When calling
this
inside the function, it typically refers to the global object. - To change the context of
this
, pass in another object as the second parameter in theforEach
method. - Run the code with the object as the second parameter to see
this
referencing the provided object instead of the global object.
- Explore how to handle the
Try experimenting with these steps in your JavaScript environment to solidify your understanding of the forEach
method. Remember to play around with different scenarios and see how you can efficiently iterate over arrays with ease!
Test your Knowledge
Which method adds an element to the end of an array?
Which method adds an element to the end of an array?
Advanced Insights into Array Methods in JavaScript
When delving deeper into array methods in JavaScript, it's crucial to grasp the intricacies of the forEach
method. This method allows for efficient iteration through arrays, providing a streamlined alternative to traditional for
loops.
Tips for Mastery:
-
Callback Functions: Utilize callback functions within the
forEach
method to perform actions on each element in an array. Remember, the first parameter passed to the callback function is always the element, followed by the index. This order ensures clarity and consistency in your code structure. -
Inline Functions: Experiment with inline functions for concise code. Whether using the
function
keyword or arrow functions, maintain consistency in parameter order (element first, index second) for effective implementation. -
Breaking Out of Loops: While
forEach
offers efficient array iteration, remember that you cannot use thebreak
statement within it. If you need to break out of a loop for specific conditions, revert to traditionalfor
loops or other array methods likeevery
,some
,find
, orfindIndex
.
Expert Recommendation:
- Understanding
this
Keyword: Explore the capabilities of thethis
keyword withinforEach
. By passing a second argument asthisArg
, you can manipulate the context in whichthis
operates. This feature proves valuable when seeking to reference specific objects or elements within your code.
Curiosity Question:
How can you leverage the this
keyword in conjunction with forEach
to optimize your JavaScript code further? What unique applications or scenarios might benefit from customizing the context of this
within array methods?
By embracing these advanced insights and recommendations, you can elevate your comprehension and utilization of array methods in JavaScript, unlocking new possibilities for efficient and elegant code solutions. Experiment, practice, and continue your exploration of JavaScript's vast capabilities in array manipulation.
Additional Resources for Arrays and the forEach Method
- MDN Web Docs - Array.prototype.forEach()
- JavaScript.info - Array Methods
- W3Schools - JavaScript Array forEach() Method
- freeCodeCamp - Learn JavaScript Array Iteration Methods
- Codecademy - JavaScript Arrays and Iterators
These resources offer in-depth explanations, examples, and exercises to further enhance your understanding of arrays and the forEach method in JavaScript. Happy coding!
Practice
Task
Task: Create an array of numbers and use map()
to double each value.
Task: Write a program that filters out all odd numbers from an array.
Task: Implement a function that sorts an array of strings alphabetically.
Task: Use reduce()
to sum all values in an array of numbers.
Task: Create a program that finds the maximum value in an array.
Task: Implement a program that reverses the order of elements in an array.
Task: Create an array of objects and use filter()
to find all items that meet a specific condition.
Task: Implement a program to merge two arrays into one.
Task: Create a program that removes the first element from an array using shift()
.
Task: Write a function that checks if an element exists in an array.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Learn Ruby Programming Online with Step-by-Step Video Tutorials
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Learn Java Programming Online with Step-by-Step Video Tutorials
Explore our Java Programming course online with engaging video tutorials and practical guides to boost your skills and knowledge.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Python Programming
Learn how to use Python for general programming, automation, and problem-solving.