Functions (Declaration, Parameters, Return values)
Functions in JavaScript are blocks of reusable code that can be executed when called. Functions help organize code and improve reusability.
Lets Go!
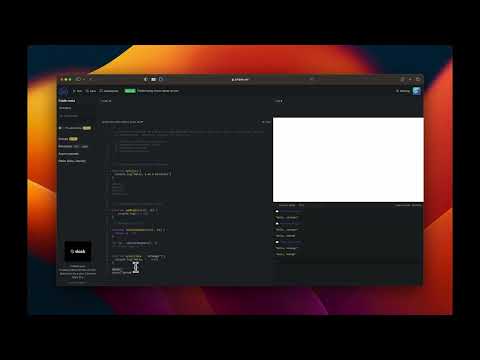
Functions (Declaration, Parameters, Return values)
Lesson 4
Understand how to create and use functions, pass parameters, and return values in JavaScript.
Get Started 🍁Introduction to Functions in JavaScript
Welcome to "Introduction to Functions in JavaScript"! Functions are an essential topic in JavaScript as they are sets of instructions designed to perform specific tasks. When a function is called or invoked, its block of code is executed, making it a powerful feature in programming.
In this course, we will cover key concepts such as declaring and calling functions, handling parameters and return values, working with anonymous functions, and utilizing function expressions. By understanding how functions work, you will be able to create reusable code and streamline your programming tasks effectively.
Have you ever wondered how functions help make your code more efficient and reusable? Join us in exploring the world of functions in JavaScript and unlock the potential of your programming skills. Let's dive in and start our journey into the fascinating realm of JavaScript functions! Happy coding! 🚀🔧📝
Main Concepts of Functions in JavaScript
-
Functions as Sets of Instructions: A function in JavaScript is a block of code designed to perform a specific task. It is executed only when called or invoked.
-
Declaring and Calling Functions: To declare a function, use the
function
keyword followed by the function name, parentheses, and function body. To call a function, use the function name followed by parentheses. -
Reusability of Functions: Functions can be reused multiple times by calling them as needed, making code more efficient and reusable.
-
Parameters and Return Values: Parameters are inputs that a function uses to perform operations. Return values are the results of these operations, which can be sent back to the calling function using the
return
keyword. -
Default Parameters: Functions can have default values for parameters, allowing them to be called without providing all arguments.
-
Anonymous Functions: Anonymous functions are simple functions without a name. They can be created using function expressions and assigned to variables for later use.
-
Function Expressions: Function expressions involve assigning functions to variables, allowing for more flexible and dynamic function calling.
By understanding these main concepts of functions in JavaScript, you can create efficient and reusable code for various tasks.
Practical Applications of Functions in JavaScript:
Step-by-Step Guide:
-
Declaring and Calling Functions:
- Declare a function using the
function
keyword followed by the function name and a block of code. - Inside the function block, include the code that you want to execute when the function is called.
- To call the function, use the function name followed by parentheses.
- Try declaring a function and calling it to see how it works.
function myFunc() { console.log("I am a function"); } myFunc();
- Declare a function using the
-
Using Parameters and Return Values:
- Define parameters inside the function parentheses to pass values to the function.
- Use these parameters in the function block as variables for operations.
- To return a value from a function, use the
return
keyword followed by the value. - Store the returned value in a variable and use it as needed.
function addNumbers(n1, n2) { return n1 + n2; } let result = addNumbers(4, 5); console.log(result); // Output: 9
-
Default Parameters:
- Provide default values for parameters in case they are not passed when calling the function.
- Use the default value if no value is provided for the parameter.
function greet(name = "stranger") { console.log("Hello " + name); } greet(); // Output: Hello stranger greet("Alice"); // Output: Hello Alice
-
Anonymous Functions and Function Expressions:
- Create functions without a name using anonymous functions.
- Use function expressions to assign functions to variables for reuse.
- Call anonymous functions through the assigned variables.
let sayHello = function() { console.log("Hello world"); }; sayHello();
Try out these practical applications by coding along with the examples. Play around with different parameters and return values to get a better understanding of functions in JavaScript. Happy coding!
Test your Knowledge
Which keyword is used to define a function in JavaScript?
Which keyword is used to define a function in JavaScript?
Advanced Insights into Functions in JavaScript
In JavaScript, functions play a crucial role in code organization and reusability. Let's delve deeper into some advanced concepts related to functions:
Function Parameters and Return Values
- Parameters are the inputs that a function uses to perform operations. They are defined within the function declaration and can be utilized as normal variables within the function block.
- Return values are the results of an operation within a function that are sent back to the calling function using the
return
keyword. - Understanding how to effectively use parameters and return values enhances the functionality and flexibility of your functions.
Curiosity Question: How can you optimize the use of parameters and return values to create versatile and efficient functions in JavaScript?
Default Parameters in Functions
- Default parameters allow you to set predefined values for function parameters. If a value is not provided when calling the function, the default value is used.
- This feature enables you to create functions that are resilient to missing arguments, providing a smooth user experience.
Recommendation: Experiment with default parameters in your functions to see how they can simplify your code and handle various use cases effortlessly.
Anonymous Functions and Function Expressions
- Anonymous functions are functions without a defined name. They are often used in scenarios where a function will not be reused or does not require a name.
- Function expressions help in utilizing anonymous functions by assigning them to variables, allowing them to be invoked and utilized within the code.
- By mastering anonymous functions and function expressions, you can enhance the modularity and flexibility of your JavaScript code.
Tip: Practice creating and calling anonymous functions using function expressions to understand their utility and application in different programming contexts.
By exploring these advanced insights into functions in JavaScript, you can elevate your coding skills and develop more robust and efficient code structures.
Curiosity Question: How can you leverage anonymous functions and function expressions to create dynamic and adaptable code solutions in JavaScript?
Additional Resources for Functions in JavaScript
- Article: JavaScript Functions - MDN Web Docs
- Video Tutorial: Understanding Functions in JavaScript
- Book: "Eloquent JavaScript" by Marijn Haverbeke (Chapter 3 covers functions in JavaScript)
Dive deeper into the world of functions in JavaScript by exploring these additional resources. Enhance your understanding and master the concept of functions to level up your coding skills! 🚀
Practice
Task
Task: Create a function that takes two numbers and returns their sum.
Task: Write a function that checks if a number is prime.
Task: Implement a function that takes a string and returns the length of the string.
Task: Create a function that returns the factorial of a number.
Task: Write a function that converts a temperature from Celsius to Fahrenheit.
Task: Create a function that accepts an array and returns the largest number.
Task: Implement a function that accepts an object and logs all of its keys.
Task: Write a function that takes a string and reverses it.
Task: Create a function that takes multiple numbers as arguments and returns their average.
Task: Write a function that checks if a string contains the letter 'a'.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Learn Ruby Programming Online with Step-by-Step Video Tutorials
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Learn Java Programming Online with Step-by-Step Video Tutorials
Explore our Java Programming course online with engaging video tutorials and practical guides to boost your skills and knowledge.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Python Programming
Learn how to use Python for general programming, automation, and problem-solving.