Building Reusable and Dynamic Angular Components
In this lesson, we'll focus on creating reusable and dynamic components in Angular. You'll learn how to design components that can be easily customized and reused across multiple parts of your application, saving development time and maintaining consistency.
Lets Go!
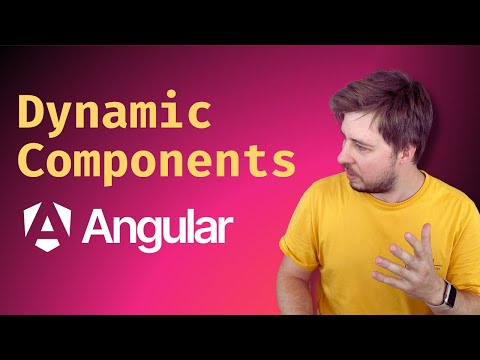
Building Reusable and Dynamic Angular Components
Lesson 5
Learn to design reusable and dynamic components in Angular using content projection, input properties, and dynamic templates.
Get Started 🍁Reusable and Dynamic Angular Components
Welcome to Level 5 of the Angular course! Reusable components are essential for efficient development and consistent UI. This lesson covers advanced techniques to make your components flexible and adaptable using Angular's tools like content projection, dynamic templates, and configuration options.
By the end of this lesson, you'll be able to create components that are not only reusable but also highly customizable, making them a valuable addition to your developer toolkit.
Main Concepts of Reusable and Dynamic Components
-
Content Projection:
- Use
<ng-content>
to project custom content into components. - Example:
<ng-content></ng-content>
- Use
-
Dynamic Templates:
- Use
ngTemplateOutlet
to render dynamic templates. - Example:
<ng-container *ngTemplateOutlet="dynamicTemplate"></ng-container>
- Use
-
Input and Output Properties:
- Use
@Input
for customizable data binding and@Output
for event handling. - Example:
@Input() title: string; @Output() onAction = new EventEmitter<void>();
- Use
-
Custom Directives:
- Create reusable behavior with directives that can be applied to any component or element.
-
Dynamic Components:
- Use
ComponentFactoryResolver
or Angular's new dynamic component rendering APIs to generate components dynamically.
- Use
Mastering these concepts will help you design versatile components for any Angular application.
Practical Applications of Reusable Components
1. Creating a Card Component
- Define a reusable card component with dynamic content:
<app-card [title]="'Card Title'"> <p>Custom content inside the card!</p> </app-card>
2. Building a Modal Component
- Use
@Input
and<ng-content>
to customize modal headers and bodies:<app-modal [visible]="isVisible" (close)="onClose()"> <ng-container modal-header> <h2>Custom Header</h2> </ng-container> <p>Modal content goes here!</p> </app-modal>
3. Implementing Dynamic Templates
- Use
ngTemplateOutlet
to create highly flexible components:<ng-template #templateA>Template A Content</ng-template> <ng-template #templateB>Template B Content</ng-template> <app-dynamic [template]="templateA"></app-dynamic>
Test your Knowledge
What is the primary purpose of the <ng-content>
tag?
What is the primary purpose of the <ng-content>
tag?
Advanced Insights into Reusable and Dynamic Components
Creating reusable and dynamic components is essential for scalable applications. Key insights include:
-
Dynamic Component Loading:
- Use Angular's
ViewContainerRef
to dynamically insert components at runtime. - Example:
this.viewContainerRef.createComponent(componentFactory);
- Use Angular's
-
Themed Components:
- Pass configuration options via
@Input
for themes, such as dark or light modes.
- Pass configuration options via
-
Lazy Loading Components:
- Combine lazy loading with dynamic components to reduce the initial application load time.
Curiosity Question:
How can dynamic templates improve the flexibility of complex UI elements?
Additional Resources for Reusable and Dynamic Components
- Content Projection in Angular: Learn about the
<ng-content>
tag. - Dynamic Component Loading: Official guide to loading components dynamically.
- Angular Directives: A comprehensive guide on custom directives.
- Template Syntax: Documentation for template features.
These resources will help you dive deeper into creating flexible and reusable components.
Practice
Task: Create a reusable button component that accepts color and size as inputs.
Task: Build a card component that uses <ng-content>
for customizable content projection.
Task: Implement a modal component that supports dynamic headers and bodies using ngTemplateOutlet
.
Task: Write a directive that toggles visibility when a condition is met.
Task: Design a dynamic component that renders different templates based on user input.
Task: Develop a reusable table component with sortable and filterable columns.
Task: Build a reusable alert component that displays different types of messages (success, error, warning).