Getting Started with Angular Components and Templates
In this lesson, we'll explore the building blocks of an Angular application: components and templates. Components are the heart of Angular applications, encapsulating logic, view, and data. Templates define the structure and appearance of your application.
Lets Go!
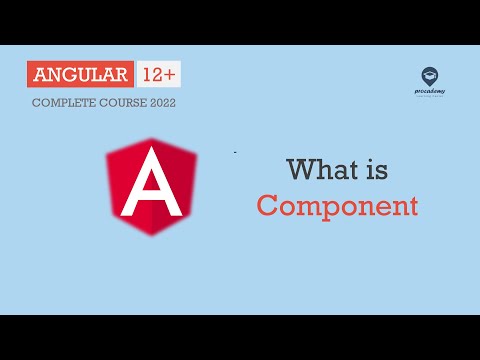
Getting Started with Angular Components and Templates
Lesson 2
Learn to create, customize, and connect Angular components using templates and understand their role in application design.
Get Started 🍁Angular Components and Templates: Building Blocks of Your Application
Welcome to the next step in your Angular journey! In this lesson, we'll delve into components and templates, the core of every Angular application.
Components in Angular enable developers to encapsulate logic, styles, and templates into reusable units, making applications modular and maintainable. Templates are the HTML structure tied to components that define how data is presented to users.
By the end of this lesson, you'll have a solid understanding of how to create, customize, and connect components in Angular applications. Let's get started!
Main Concepts of Angular Components and Templates
-
Components: The core building blocks of Angular applications. Each component consists of:
- A TypeScript class containing logic (e.g.,
AppComponent
). - An HTML template defining the structure and content.
- CSS/SCSS for styling the component.
- A TypeScript class containing logic (e.g.,
-
Templates: HTML structures tied to components. Angular templates use powerful syntax, including:
- Interpolation (
{{}}
) for displaying data. - Directives (e.g.,
*ngIf
,*ngFor
) for dynamic content.
- Interpolation (
-
Component Interaction: Passing data between parent and child components using Input and Output decorators.
Understanding these concepts allows you to create dynamic and interactive web applications effortlessly.
Practical Applications of Components and Templates
Creating Your First Angular Component
- Open your terminal and navigate to your Angular project folder.
- Run the following command to generate a new component:
ng generate component my-first-component
- Open the newly created files in the
src/app/my-first-component
directory. - Update the HTML template (e.g.,
my-first-component.component.html
) to display:<h1>Hello, Angular Components!</h1>
- Add the selector
<app-my-first-component>
toapp.component.html
to render your new component in the main application.
Customizing Components
- Use
@Input
to pass data from a parent to a child component. - Use
@Output
to emit events from a child component to a parent.
Experiment with templates and component logic to understand their interaction.
Test your Knowledge
What is a component in Angular?
What is a component in Angular?
Advanced Insights into Components and Templates
Mastering components and templates is crucial for building scalable Angular applications. Key concepts to explore further include:
- Lifecycle Hooks: Angular components have lifecycle hooks like
ngOnInit
andngOnDestroy
that allow you to execute logic at specific stages. - Dynamic Components: Learn how to create and load components dynamically using Angular's
ComponentFactoryResolver
. - ViewChild and ContentChild: Access child components or DOM elements in your component class for advanced interactions.
Curiosity Question:
How does Angular's template syntax enhance developer productivity compared to traditional HTML development?
Additional Resources for Components and Templates
- Angular Documentation on Components: Comprehensive guide to Angular components.
- Tutorial: Parent-Child Communication: Learn how to pass data between components.
- Angular Template Syntax: Detailed documentation on Angular's powerful template syntax.
Leverage these resources to strengthen your understanding of components and templates. Remember, practice is key!
Practice
Task: Create an Angular component that displays a list of items using the *ngFor
directive.
Task: Build a parent component that passes data to a child component using @Input
.
Task: Develop an Angular component with a button that emits an event using @Output
.
Task: Write an Angular template that conditionally displays content using the *ngIf
directive.
Task: Create a reusable Angular component for displaying user profiles with dynamic data.
Task: Develop a component that fetches data from an API and displays it in a table.
Task: Build a simple Angular form with input fields and submit functionality.
Task: Implement a feature where clicking a button in one component updates content in another component.
Task: Create an Angular app with a navigation bar and multiple routed components.
Task: Write an Angular component that uses a custom pipe to format user data.