Introduction to Angular
Angular is a popular open-source framework for building dynamic web applications. Developed by Google, Angular allows developers to create highly scalable and performant single-page applications (SPAs) with ease. It uses TypeScript as its primary programming language, ensuring enhanced developer experience and robust applications.
Lets Go!
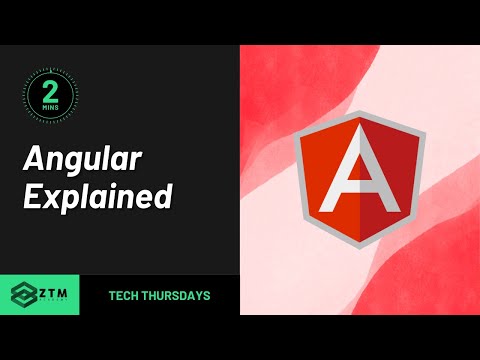
Introduction to Angular
Lesson 1
Understand what Angular is, its architecture, and how it simplifies web development.
Get Started 🍁Introduction to Angular Framework: Everything You Need to Know
Welcome to the "Introduction to Angular" course! My name is Caleb, and I am thrilled to guide you through this series where you'll learn everything you need to become a proficient Angular developer.
In this course, we will start with the basics, suitable for complete beginners, and by the end, you'll be developing complex, scalable Angular applications. Unlike other tutorials online, I aim to make this journey engaging and enjoyable while diving deep into Angular concepts.
Angular is a powerful and versatile framework that simplifies the development of modern web applications. It ensures seamless user experiences through client-side rendering, reactive forms, and dependency injection. Throughout this series, you'll gain an understanding of Angular's architecture, powerful CLI tools, and features like components, directives, and services.
To get started, you'll need to install Node.js and Angular CLI, the command-line tool that simplifies Angular development. Don't worry; I'll guide you through every step. Each video in this series will be paired with a blog post containing code examples and additional resources for deeper understanding.
Are you ready to start your Angular development journey? In the next video, we will create our first Angular application together. Remember, practice is key to mastering Angular, and with dedication, you'll be building stunning web applications in no time!
If you have any questions or need help, feel free to leave a comment. Don't forget to subscribe to the channel for updates. Let’s dive into Angular and unlock your web development potential! 🚀
Subscribe to the channel Check out the Angular crash course Explore our sponsor, Pramp
Main Concepts of Angular Development
-
Angular is a powerful framework: It provides tools for building feature-rich, single-page applications (SPAs) with a focus on performance and maintainability.
-
Component-Based Architecture: Angular applications are built with reusable components, making code organization and scaling much easier.
-
Reactive Programming: Using RxJS, Angular supports reactive programming for handling asynchronous data streams effortlessly.
-
Dependency Injection: Angular's built-in dependency injection system simplifies the management of services and shared resources across an application.
-
Angular CLI: The Angular Command Line Interface is a powerful tool for scaffolding, managing, and building Angular projects with minimal setup.
-
Two-Way Data Binding: Angular provides seamless synchronization between the UI and application state.
By understanding these concepts, learners can create robust, scalable Angular applications and improve their web development skills.
Practical Applications of Angular Development
Getting Started with Angular
Let's dive into creating your first Angular application! Follow these steps to set up your development environment:
- Download and install Node.js.
- Install Angular CLI globally by running
npm install -g @angular/cli
in your terminal. - Create a new Angular application using
ng new my-angular-app
and follow the prompts to set up the project. - Navigate into your project folder and run
ng serve
to start the development server. - Open your browser and visit
http://localhost:4200
to see your first Angular application in action.
Feel free to ask questions or share your progress in the comments. Happy coding! 🚀
Test your Knowledge
What is Angular primarily used for?
What is Angular primarily used for?
Advanced Insights into Angular Development
To master Angular, understanding its architecture and core principles is essential. Angular leverages TypeScript and a modular approach to enhance development efficiency and maintainability.
Tips for Mastery:
-
Component Interaction: Learn how to pass data between components using Input and Output decorators.
-
Routing: Understand Angular's powerful Router module for navigating between different views in your application.
-
Reactive Forms: Use reactive forms for handling complex form scenarios with advanced validation.
Expert Advice:
Grasping Angular’s core concepts like change detection, lazy loading, and RxJS can significantly enhance your productivity and ability to handle complex projects. Experiment with Angular’s CLI commands to speed up repetitive tasks and maintain consistency in your codebase.
Curiosity Question:
How does Angular’s dependency injection system improve the development experience compared to traditional JavaScript development? Explore how Angular simplifies managing shared services and resources.
Additional Resources for Angular Development
-
[Blog Posts](link to blog posts): Each video in this series is paired with a blog post that includes examples and explanations.
-
[Angular Crash Course](link to Angular crash course): If you prefer a concise overview of Angular, check out the crash course with practical examples.
-
[Pramp](link to Pramp website): Our sponsor, Pramp, offers a great platform for honing your coding and technical interview skills. It’s a valuable resource for preparing for top-tier job opportunities.
Leverage these resources to strengthen your Angular knowledge and build impressive applications. If you have any questions, feel free to ask. Don't forget to subscribe for more Angular content!
Practice
Task: Create a simple Angular component that displays "Hello, Angular!" on the screen.
Task: Build an Angular component that accepts a user's name as input and displays a personalized greeting.
Task: Develop an Angular form for user registration with validation for required fields.
Task: Create an Angular service that fetches data from an API and displays it in a component.
Task: Write an Angular application with a navigation menu and multiple routes.
Task: Create a to-do list application using Angular, allowing users to add and remove tasks.
Task: Implement a search feature in an Angular application that filters a list of items based on user input.
Task: Create an Angular app that uses a custom pipe to format dates in a specific style.
Task: Build an Angular application that displays data in a table with sorting and filtering capabilities.
Task: Write an Angular app that demonstrates lazy loading for different modules.