Mastering Angular Services and Dependency Injection
In this lesson, we will dive into Angular services and dependency injection (DI), which are essential for creating scalable and maintainable applications. Services are used to share data, logic, and functions across different parts of an application. Dependency injection simplifies managing dependencies by providing a flexible way to supply them throughout the application.
Lets Go!
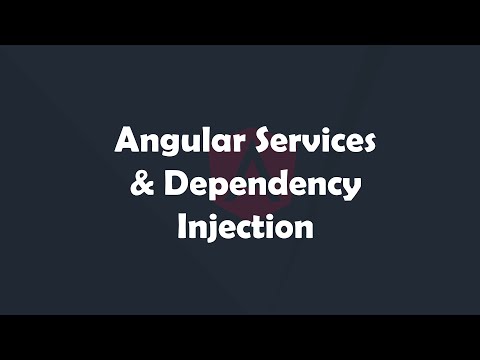
Mastering Angular Services and Dependency Injection
Lesson 3
Understand the purpose of Angular services and dependency injection, and learn how to use them effectively to build scalable and maintainable applications.
Get Started 🍁Angular Services and Dependency Injection: Powering Your Application Logic
Welcome to Level 3 of our Angular course! This lesson focuses on services and dependency injection, key concepts for building robust Angular applications.
Angular services allow you to encapsulate reusable logic that can be shared across components, while dependency injection provides a framework to manage these services efficiently. Together, they enable you to build clean and maintainable applications.
By the end of this lesson, you'll be able to create services, inject them into components, and leverage Angular's DI system for advanced scenarios.
Main Concepts of Angular Services and Dependency Injection
-
Services: Classes that encapsulate reusable logic. Examples include:
- Fetching data from an API.
- Managing application state.
- Providing utility functions.
-
Dependency Injection (DI): A design pattern in Angular for providing dependencies to components or services. Key concepts include:
- Providers: Used to register services.
- Injectable Decorator: Marks a class as injectable.
- Hierarchical Injectors: Control the scope of dependencies.
-
Scoping Services: Define the scope of a service at the module or component level using Angular's DI hierarchy.
Practical Applications of Services and Dependency Injection
Creating and Using a Service
- Open your terminal and navigate to your Angular project folder.
- Generate a new service using the Angular CLI:
ng generate service data
- Update the generated
data.service.ts
file to fetch API data:import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Injectable({ providedIn: 'root' }) export class DataService { constructor(private http: HttpClient) {} getData() { return this.http.get('https://api.example.com/data'); } }
- Inject the service into a component:
import { Component, OnInit } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-data', template: `<div *ngIf="data">{{ data | json }}</div>` }) export class DataComponent implements OnInit { data: any; constructor(private dataService: DataService) {} ngOnInit() { this.dataService.getData().subscribe(res => this.data = res); } }
Creating Scoped Services
- Use the
providers
array in the component or module decorator to scope services locally.
Test your Knowledge
What is the main purpose of Angular services?
What is the main purpose of Angular services?
Advanced Insights into Services and Dependency Injection
Understanding the DI system allows you to build flexible and scalable applications. Explore these advanced concepts:
- Custom Providers: Define custom providers with
useClass
,useValue
, oruseFactory
for more control over service instantiation. - Injection Tokens: Use injection tokens to inject non-class dependencies like configuration objects.
- Tree-Shakable Providers: Optimize your application by leveraging tree-shakable services in Angular.
Curiosity Question:
What are the benefits of a hierarchical dependency injection system in large-scale applications?
Additional Resources for Services and Dependency Injection
- Angular Dependency Injection Guide: Comprehensive guide to Angular's DI system.
- Creating and Managing Services: Learn how to use services effectively.
- Understanding Providers in Angular: Detailed guide on Angular's provider system.
Dive into these resources to deepen your knowledge of services and dependency injection. Keep experimenting to master these concepts!
Practice
Task: Create a service to manage a list of items and inject it into multiple components.
Task: Build a component that uses a service to fetch and display API data.
Task: Develop a service with @Injectable
that utilizes Angular's HttpClient to POST data to a server.
Task: Create a parent component that injects a scoped service to manage local state.
Task: Implement a service with a custom provider using the useFactory
syntax.
Task: Build a component that demonstrates the use of an injection token for passing configuration settings.
Task: Write unit tests for a service using Angular's TestBed.
Task: Develop an application that uses multiple hierarchical injectors for service scoping.
Task: Create a singleton service and demonstrate its usage in various components.
Task: Implement a service that caches API responses to optimize performance.