Basic control structures
Control structures in C++ programming dictate the flow of execution in a program. They include sequential, selection, and iteration structures.
Lets Go!
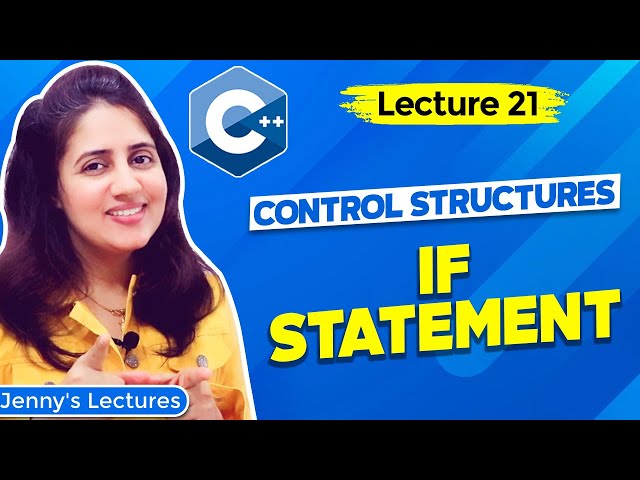
Basic control structures
Lesson 10
Understand how to use 'if', 'else', 'while', 'for', and 'do-while' to control the flow of a program.
Get Started 🍁Introduction to Control Structures in C++
Welcome to our course on "Introduction to Control Structures in C++"!
In this course, we will delve into the fundamental concepts of control structures, which are essential for designing efficient and structured programs. Control structures are mechanisms in programming that allow you to dictate the flow of your program based on certain conditions.
We will start by exploring the three basic types of control structures: sequential, selection, and iteration. Sequential control structures execute statements line by line in the order they appear, selection control structures help you make decisions based on conditions, and iteration control structures allow you to repeat tasks based on conditions.
Have you ever wondered how programming languages decide what action to take based on specific conditions? How do they handle multiple choices or repeated tasks efficiently?
In this course, we will answer these questions and more, as we cover topics such as if statements, and explore the intricacies of writing conditional statements in C++. We will also delve into common pitfalls and tricky questions that arise when working with if statements, and how to overcome them.
Get ready for a deep dive into the world of control structures in C++, where we will demystify complex concepts and equip you with the tools to write efficient and structured programs. So, are you ready to explore the power of if statements in programming? Let's dive in and discover the endless possibilities control structures offer in C++!
Main Concepts of Control Structures in C++
-
What is a Control Structure: Control structures in C++ are used to dictate the flow of a program. There are three basic types of control structures: sequential, selection, and iteration.
-
Sequential Control Structure: In a sequential control structure, statements are executed one after the other in the order they appear in the program. The control starts from the main function and moves through each line of code sequentially until the program reaches the end.
-
Selection Control Structure: Selection control structures are used when decisions need to be made based on certain conditions. If you have multiple choices or conditions to consider, you can use selection control structures to determine which block of code to execute.
-
Types of Selection Statements: There are different types of selection statements in C++, such as
if
,if-else
,else-if ladder
,nested if
, andswitch
. -
Iterative Control Structure: Iterative control structures are used when you need to repeat a block of code multiple times based on a certain condition. This allows for repetitive execution of code until a certain condition is met.
-
Types of Iterative Statements: The three main types of iterative statements in C++ are
for loop
,while loop
, anddo-while loop
. These loops help in repeating a set of statements until a particular condition is no longer true.
Practical Applications of Understanding Control Structures in C++
To apply the concepts of control structures in C++, follow these steps:
-
Setting Up the Program
- Include the necessary header file:
#include <iostream>
- Use the
using namespace std;
directive for convenience.
- Include the necessary header file:
-
Defining a Variable and Implementing an If Statement
- Declare a variable to store the amount of money you have, for example:
int money;
- Ask the user to input the amount of money using
cin >> money;
- Utilize an if statement to check a condition (e.g., if the money is greater than 1000):
if (money >= 1000) { cout << "Coffee in Starbucks" << endl; } else { cout << "Let's go home" << endl; }
- Declare a variable to store the amount of money you have, for example:
-
Running the Program
- Compile and execute the program.
- Enter different values for the money variable to see how the if statement behaves.
- Experiment with different conditions using logical operators (
>
,>=
,<
,<=
,==
,!=
) and logical combinations (&&
,||
,!
).
-
Exploring Advanced If Statement Usage
- Try terminating an if statement with a semicolon and observe its effect.
- Experiment with assignment (
=
) versus equality check (==
) in conditions to see the outcomes. - Test combining multiple conditions using logical operators (
&&
,||
) to create more complex logic.
-
Advanced Techniques
- Challenge yourself by combining multiple if statements.
- Consider using if-else statements to cover alternative scenarios.
- Explore if-else-if chains for multi-step decision-making.
-
Experiment and Practice
- Modify the program to include additional conditions and enhance the decision-making process.
- Test different scenarios, inputs, and conditions to gain a deeper understanding of how control structures work.
Remember to play around with the concepts and code snippets provided above to get a hands-on experience with control structures in C++. Feel free to share your results and questions in the comments section. Happy coding!
Test your Knowledge
Which of the following is a control structure in C++?
Which of the following is a control structure in C++?
Advanced Insights into C++ Programming: Control Structures
In this section, we will delve deeper into the concept of control structures in the C++ programming language. Control structures enable us to dictate the flow of our programs, allowing us to make decisions and repeat tasks based on specific conditions. Let's explore some advanced aspects and insights:
1. Sequential Control Structure:
Sequential control structure is the default behavior of programs, where statements are executed line by line in the order they appear. It is essential to understand how the flow of execution progresses through a program, starting from the main function.
Curiosity Question: How does the sequential control structure impact program efficiency and readability?
2. Selection Control Structures:
Selection control structures help us make decisions in our programs based on certain conditions. By using if, if-else, and switch statements, we can determine which block of code to execute depending on the evaluation of a specific condition.
Tip: Experiment with combining logical operators and relational operators within if statements to create more complex decision-making scenarios.
3. Iterative Control Structures:
Iterative control structures allow us to repeat tasks multiple times based on a given condition. By utilizing for loops, while loops, and do-while loops, we can iterate through code blocks until a certain condition is met.
Expert Advice: Consider the efficiency of different loop structures based on the specific requirements of your program to optimize performance.
Curiosity Question: How can you optimize the use of control structures to enhance program logic and readability?
By mastering control structures in C++, you can enhance the functionality and flexibility of your programs while improving the overall structure and logic flow. Dive deeper into these concepts, experiment with different scenarios, and challenge yourself with complex decision-making tasks to further solidify your understanding of control structures in C++.
Additional Resources for C++ Programming Control Structures
Explore these resources to deepen your understanding of control structures in C++ programming. They provide valuable insights, examples, and explanations to enhance your knowledge and skills in this topic. Happy learning!
Practice
Task: Write a C++ program that asks the user for their name and age, and then prints a greeting message using their input.