Basic syntax and structure of a C++ program
This lesson delves into the basics of C++ syntax, focusing on classes, objects, and keywords. It explains the concepts of classes as templates for objects, objects as instances of classes, methods as behaviors within classes, and instance variables for unique object properties.
Lets Go!
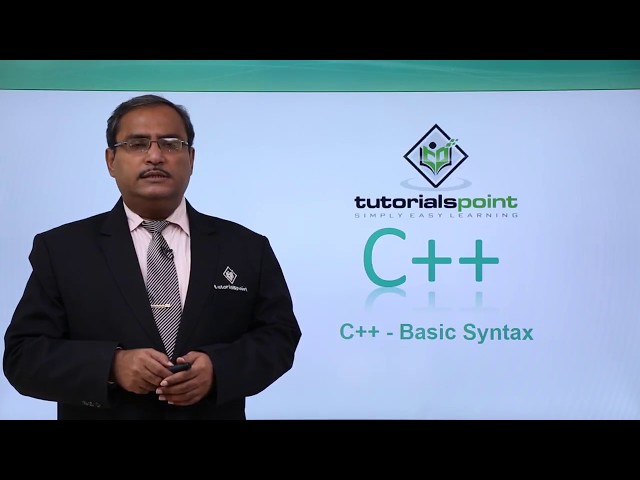
Basic syntax and structure of a C++ program
Lesson 5
Understand the components of a C++ program, such as headers, the main() function, and statements.
Get Started 🍁Introduction to C++ Programming
Welcome to the "Introduction to C++ Programming" course! In this course, we will delve into the fundamental aspects of C++ programming, starting with the basic syntax. By the end of this course, you will have a solid understanding of key concepts such as classes, objects, methods, instance variables, tokens, identifiers, and more.
Have you ever wondered how a simple C++ program functions behind the scenes?
In this course, we will explore the building blocks of C++ programming, beginning with defining objects as collections that communicate through invoking methods. We will decipher the meanings of classes, objects, methods, and instance variables, understanding how memory spaces are allocated and instances are instantiated during runtime.
Throughout the course, we will dissect the syntax of a C++ program, including tokens, keywords, whitespace usage, and line terminations. By the end of this course, you will be equipped with the essential knowledge to write, analyze, and comprehend C++ code effectively.
Join us on this educational journey as we uncover the intricate world of C++ programming. Let's embark on a rewarding learning experience together!
Main Concepts of C++ Basics
-
Objects: Objects are a collection of properties and behaviors that communicate by invoking each other's methods. Properties are values held in variables, and behaviors are actions denoted by methods. For example, a dog object may have properties like color, name, breed, and behaviors like wagging, barking, eating, etc.
-
Class: A class is a template or blueprint that describes the behavior and states that objects of its type will support. An object is an instance of a class, which means memory space is allocated during runtime for its properties.
-
Methods: Methods are behaviors in a class that contain logic, manipulate data, and execute actions. They can be called class methods, member functions, or member methods.
-
Instance Variables: Each object has a unique set of instance variables that create the object's state. These variables, also known as member variables, hold specific values for the object.
-
Tokens: In C++, a program consists of various tokens like keywords, identifiers, constants, string literals, and symbols. Tokens are essential for writing and interpreting code correctly.
-
Semicolons: In C++, a semicolon is a statement terminator that indicates the end of each individual statement. Different programming languages may have different punctuation symbols, but C++ uses a semicolon for statement termination.
-
Blocks: A block is a set of logically connected statements surrounded by opening and closing braces. In C++, statements enclosed within curly braces form a block and denote logically connected instructions.
-
C++ Identifiers: An identifier in C++ is a name used to identify variables, functions, classes, modules, or any user-defined item. Identifiers must start with an alphabet (lower or upper case) or an underscore and can include digits after the initial character.
-
Keywords: Keywords are reserved words in C++ that have predefined meanings and cannot be used as identifiers. These words are part of the language's syntax and serve specific purposes.
-
White Spaces: White spaces in C++ are blank lines or characters that include spaces, tabs, and newlines. Whitespace is used to separate tokens and improve code readability but is ignored by the compiler during compilation.
Practical Applications of C++ Basic Syntax
In this section, we will explore practical applications of C++ basic syntax discussed in the video. Follow these steps to understand and implement the concepts:
-
Understanding Objects, Classes, Methods, and Instance Variables:
- Objects: Represent instances of classes holding properties and behaviors.
- Classes: Templates or blueprints describing behavior states.
- Methods: Behaviors defined in classes for manipulating data.
- Instance Variables: Unique set of variables in each object to hold values.
-
Working with Tokens in C++:
- C++ program consists of various tokens like keywords, identifiers, constants, string literals, or symbols.
- Each statement must end with a semicolon, acting as a statement terminator.
-
Writing and Understanding Blocks in C++:
- A block is a set of logically connected statements enclosed within curly braces
{}
. - Use opening and closing braces to denote statements within the same block.
- A block is a set of logically connected statements enclosed within curly braces
-
Using C++ Identifiers and Keywords:
- Identifiers are names for variables, functions, classes, etc.
- Variable names should start with an alphabet or underscore, followed by alphabets or digits.
- C++ has reserved keywords for specific functions and operations.
-
Managing White Spaces in C++:
- White spaces are blank lines or spaces in the code.
- White spaces and comments are ignored by the compiler.
- Use white spaces for readability and organization in the code.
-
Practice Hands-On:
- Create a C++ program with classes, methods, and instance variables.
- Define identifiers using valid naming conventions.
- Experiment with white spaces to enhance code readability.
Try It Out!
- Create a simple C++ program with a class named
Animal
containing methods likeeat()
,sleep()
, and instance variables likecolor
,name
, etc. - Define identifiers for variables following C++ naming conventions.
- Experiment with different token types like keywords and symbols in your program.
- Use white spaces effectively to organize your code and make it readable.
Engage with these practical applications to deepen your understanding of C++ basic syntax. Have fun exploring and coding! 🚀
Test your Knowledge
Which function is mandatory in every C++ program?
Which function is mandatory in every C++ program?
Advanced Insights into C++ Syntax
In this section, we will delve deeper into the basic syntax of C++ and explore some advanced aspects of this programming language.
Classes, Objects, Methods, and Variables
-
Objects: Objects have properties and behaviors. Properties hold values in variables, while behaviors are represented by methods. For example, a dog's properties may include color, breed, and name, while behaviors can be wagging, barking, and eating.
-
Classes: A class is a template or blueprint describing the behavior and states that objects of its type will support. An object is an instance of a class, with memory spaces allocated during runtime, known as instantiation.
-
Methods: Methods are behaviors written in classes, where logic is executed, actions are performed, and data is manipulated. They are also referred to as member functions or member methods.
-
Instance Variables: Each object has a unique set of instance variables, creating the object's state. These variables hold values and can be called member variables residing in an object.
Curiosity Question: How can the principles of encapsulation and inheritance be applied to optimize the design and implementation of classes and objects?
Tokens in C++
-
Tokens: In C++, a program comprises various tokens like keywords, identifiers, constants, string literals, and symbols (e.g., semicolon). Different languages may have distinct punctuation symbols for statement termination.
-
Lines and Blocks: A semicolon terminates a statement in C++. Statements separated by semicolons can be written on the same line or different lines for readability. Logical instructions encapsulated in opening and closing braces form blocks in C++.
Curiosity Question: How does the syntax of C++ contribute to its readability and maintainability in large-scale software development projects?
Identifiers, Keywords, and White Spaces
-
Identifiers: An identifier in C++ is a name used for variables, functions, classes, or user-defined items. It must start with an alphabet and can contain digits and underscores. Punctuation characters are disallowed within identifiers.
-
Keywords: C++ has a list of predefined keywords that serve specific purposes, aiding in language understanding and syntax parsing.
-
White Spaces: White spaces, including blank lines and escape characters, are crucial for code readability. While they are essential for the compiler to differentiate elements, excessive or unnecessary white spaces can impact code reading and maintenance.
Curiosity Question: How does the effective use of identifiers, keywords, and white spaces enhance code clarity and facilitate collaboration in a team-based programming environment?
By exploring these advanced insights into C++ syntax, you can deepen your understanding of key concepts and unlock the full potential of this language in your programming endeavors. Remember, mastering the nuances of syntax and structure can significantly impact the efficiency and effectiveness of your coding practices. Happy coding!
Additional Resources for C++ Basic Syntax
For those looking to further their understanding of C++ basic syntax, here are some additional resources to explore:
These resources provide in-depth explanations and examples to help you grasp the concepts discussed in the video. Happy coding!
Practice
Task: Create a C++ program that uses multiple statements to print your name, age, and favorite programming language.