Functions in C++
Functions in C++ programming are a group of program statements with a unique name that performs a specific task. They provide modularity and reusability to a program, making it more efficient and organized.
Lets Go!
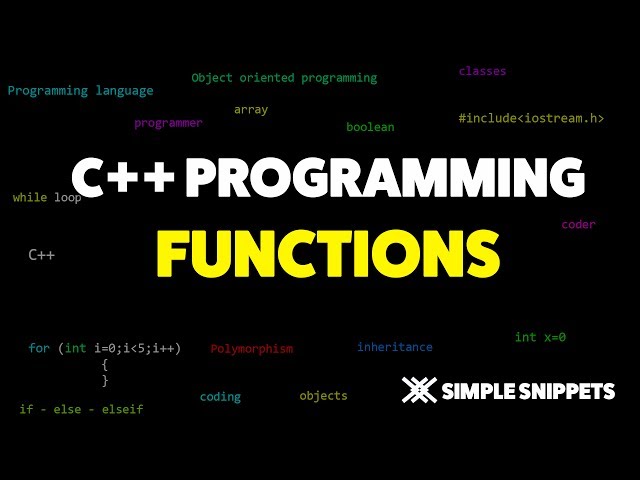
Functions in C++
Lesson 11
Learn how to define functions, pass parameters, and call them in a C++ program.
Get Started 🍁Introduction to Functions in C++ Programming
Welcome to "Introduction to Functions in C++ Programming"! This course will provide you with a comprehensive understanding of functions, a crucial topic in the procedural paradigm of C++ programming.
Functions in programming are defined as a group of program statements with a unique name that perform specific tasks. They play a vital role in providing modularity and reusability to a program, making it more organized and efficient.
In this course, we will delve into the theoretical aspects of functions, including their syntax, return types, function names, parameter lists, and function signatures. We will also explore the practical implementation of functions through coding examples.
Have you ever wondered how functions work behind the scenes in programming languages like C++? What advantages do functions offer in terms of code organization and reusability? Join us on this learning journey to uncover the answers to these questions and more.
Let's kickstart your understanding of functions and empower you to write more efficient and structured C++ programs. Get ready to explore the power of functions in programming and enhance your coding skills! Are you ready to dive in?
Main Concepts of Functions in C++ Programming
-
Functions: In C++ programming, a function is a group of program statements with a unique name that performs a specific task. Functions are essential in providing modularity and reusability to a program.
-
Control Transfer: When a program contains a function, the control transfers from the main function to the user-defined function, executes the function, and then transfers back to the main function to continue execution until the program ends.
-
Syntax of Functions:
- Return Type: The data type of the value that the function returns. If a function does not return a value,
void
is used. - Function Name: The unique identifier of the function used when calling the function inside the main function.
- Parameter List: The list of parameters the function takes.
- Function Body: The code block within curly braces that defines what the function does.
- Return Type: The data type of the value that the function returns. If a function does not return a value,
-
Function Signature: The entire first line of the function (return type, function name, and parameter list) is known as the function signature. It uniquely identifies the function.
-
Function Definition vs. Function Call: The function definition specifies what the function does, while the function call involves using the function's name to execute its task.
-
Forward Declaration: It is essential to declare the function signature above the main function to inform the compiler about the existence of the user-defined function to prevent errors during compilation.
-
User-Defined Functions: Functions created by the user to perform specific tasks, enhancing code reusability and modularity.
-
Function Parameters: Functions can be categorized as parametric (taking parameters) or non-parametric (not taking parameters). Parameters allow passing values to functions for computation.
Practical Applications of Functions in C++ Programming
Functions in C++ programming are essential for providing modularity and reusability to a program. By encapsulating a set of program statements with a unique name that performs a specific task, functions allow for efficient coding and easier maintenance. Let's dive into a step-by-step guide to implementing and understanding functions in C++:
Step 1: Define and Implement a Function
- Open your C++ IDE and start a new file (e.g.,
example.cpp
). - Include the necessary header files (
#include <iostream>
). - Define your main function with the essential boilerplate code (
int main() { }
). - Declare any required variables and prompt the user for inputs (e.g., integers a and b).
- Define a function outside the main function with a unique name and return type (
int maximum(int x, int y) { }
). - Implement the function logic to determine and return the maximum value of two input parameters (e.g.,
return (x > y) ? x : y;
). - Call the function within your main function and store the returned value in a variable (e.g.,
int result = maximum(a, b);
). - Print the result to the console using
cout
.
Step 2: Handle Function Declarations
- If you define the function below the main function, use a forward declaration at the top of your file to inform the compiler about the function's signature (
int maximum(int x, int y);
). - This declaration ensures that the compiler recognizes the function before encountering its usage.
Step 3: Optimize Function Usage
- To demonstrate reusability, consider calling the function multiple times within your code for different inputs.
- Explore scenarios where functions may not return a value (void return type) but perform specific tasks like printing messages.
- Experiment with different variations of functions, such as parameterized and non-parameterized functions.
By following these steps, you can gain hands-on experience with implementing functions in C++ programming and understand their significance in enhancing code organization, efficiency, and maintainability. Don't hesitate to experiment further and explore additional functionalities of functions in C++ programming. Happy coding! 🚀
Feel free to try out these practical steps in your C++ IDE and enhance your understanding of functions by applying them to real-world scenarios. If you encounter any challenges or have questions, feel free to ask in the comments. Share your experience with using functions in programming and don't forget to subscribe for more tutorials! 🖥️🔍👨💻
Test your Knowledge
What is the correct syntax to define a function in C++?
What is the correct syntax to define a function in C++?
Advanced Insights into Functions in C++ Programming
In the realm of C++ programming, functions play a crucial role in enhancing the modularity and reusability of a program. Understanding the advanced aspects of functions can elevate your programming skills to the next level.
Advanced Aspects:
-
Return Type:
- The return type of a function dictates the data type of the value that the function will return upon completion.
- Utilize the return type wisely to ensure clarity and coherence in your code.
-
Function Name:
- The name of a function serves as a unique identifier and is essential for invoking the function within the main program.
- Opt for descriptive and meaningful names to enhance code readability and maintainability.
-
Parameter List:
- Parameters allow you to pass values into a function for processing.
- Carefully define the parameter list to match the data types and quantities required by the function.
Expert Recommendation:
- When creating a function, consider the broader context of your program and strive for a balance between simplicity and efficiency.
- Practice using functions in diverse scenarios to solidify your understanding and proficiency in leveraging this fundamental programming concept.
Curiosity Question:
How can you optimize the use of functions in your programs to achieve maximum efficiency and code reusability?
By delving into the intricacies of functions and experimenting with different implementations, you can unlock the true potential of this foundational concept in C++ programming. Happy coding!
Additional Resources for Functions in C++ Programming
Explore these resources to gain a deeper understanding of functions in C++ programming. Enhance your knowledge and skills by learning more about function syntax, return types, and parameter passing. Happy coding! 🖥️👨💻
Practice
Task: Write a C++ program that defines a function to calculate the factorial of a number and calls it to display the result for a user-provided number.