Input and output operations (cin, cout)
This lesson discusses how to take input from the user and give output in C++ programming. It covers the basics of input and output operations in C++ code.
Lets Go!
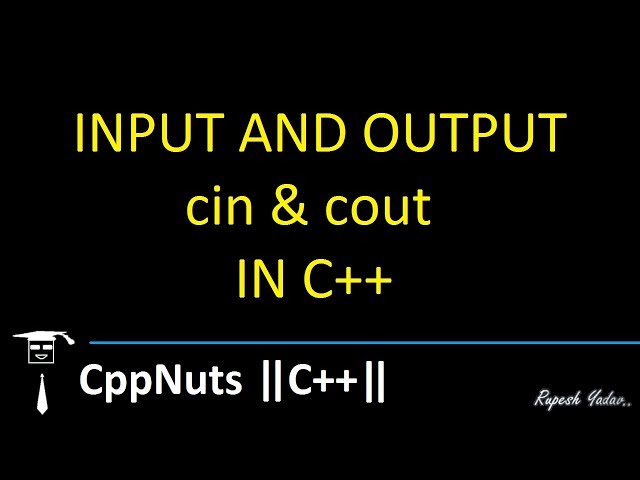
Input and output operations (cin, cout)
Lesson 9
Learn to take user input using cin and display output using cout.
Get Started 🍁Introduction to C++ Programming: Input and Output Operations
Welcome to the course "Introduction to C++ Programming"! In this course, we will demystify the world of C++ and explore the fundamental concepts that form the backbone of programming in this powerful language.
Have you ever wondered how to take input from a user and provide output in a C++ program? If so, you're in the right place! Throughout this course, we will cover essential topics such as input and output operations in C++ code, the role of variables, and different data types at your disposal.
We will start by understanding how to take input from the user using the Cin
command and how to display output using the Cout
command. By the end of this course, you will be able to perform operations on variables, handle different data types like integers, strings, and even floating-point numbers, and much more.
If you are a beginner in programming or looking to enhance your C++ skills, this course is perfect for you. Get ready to dive into the world of C++ programming and unlock the potential to create exciting programs that showcase your creativity and logic!
Are you ready to embark on this coding journey? Let's get started!
Main Concepts of C++ Programming
-
Input and Output Operations:
- In C++, input and output operations involve taking input from the user and giving output to the user. This is achieved using
cin
for input andcout
for output.
- In C++, input and output operations involve taking input from the user and giving output to the user. This is achieved using
-
Concatenating Output Strings:
- When using
cout
to output strings, you can concatenate multiple strings by using multiple<<
operators and spaces between the strings.
- When using
-
Breaking Lines in Output:
- To break a line in the output, you can use the
endl
command or\n
in yourcout
statement to move the output to the next line.
- To break a line in the output, you can use the
-
Taking Input from the User:
- To take input from the user in C++, you need to create a variable to store the input. This variable needs to match the data type of the input you are expecting (e.g., integer, string).
-
Variables and Data Types:
- Variables in C++ are containers that hold values, and they must be declared with a specific data type (integer, string, float, etc.) to define what type of values they can store.
-
Performing Operations on Variables:
- Once you have taken input into a variable, you can perform various operations on it, such as addition, subtraction, multiplication, and division, depending on the data type of the variable.
-
Using Different Data Types:
- C++ provides various data types like integers, strings, floats, etc. Understanding and using different data types correctly is essential for effective programming and manipulation of data.
-
Advanced C++ Programming:
- Learning the basics of input, output, variables, and data types in C++ is fundamental to approaching more advanced C++ programming concepts and building complex applications.
Practical Applications of Input and Output Operations in C++
Step-by-Step Guide:
-
Introducing
std::endl
for Line Breaks:- In your C++ code, use
std::endl
to break a line when outputting text. - Example:
std::cout << "Hi there" << std::endl; std::cout << "Bingo Dingo" << std::endl;
- In your C++ code, use
-
Taking User Input using
std::cin
:- Create a variable to store the user input, defining its data type (e.g., integer or string).
- Use
std::cin
to prompt the user for input and store it in the variable. - Example for integer input:
int number; std::cin >> number;
-
Outputting User Input:
- Output the user input stored in a variable.
- Example:
int number; std::cout << "Enter a number: "; std::cin >> number; std::cout << "The number you entered is: " << number << std::endl;
-
Performing Operations on User Input:
- You can manipulate user input by performing calculations or operations.
- Example for adding 10 to user input:
int number; std::cin >> number; std::cout << "Original number: " << number << std::endl; std::cout << "After adding 10: " << (number + 10) << std::endl;
Try It Out:
- Create a C++ program following the steps above to take user input, output it, and perform an operation on the input.
- Experiment with different data types (e.g., float, string) and operations to further understand input and output operations in C++.
- Play around with calculations and outputs to get comfortable with manipulating user input through your code.
Enjoy experimenting with input and output operations in C++! Feel free to explore more advanced concepts in the next video on variables and data types.
Test your Knowledge
Which object is used to take input in C++?
Which object is used to take input in C++?
Advanced Insights into Input and Output Operations in C++
Understanding how to take input from the user and provide output is fundamental in programming. Let's delve deeper into some advanced aspects of input and output operations in C++.
Output and Line Breaks
- When using
cout
to give output to the user, remember that you can include multiple strings or variables in the same line. Use<< endl;
or<< "\n";
to explicitly break the line and move to the next line. - Curiosity Question: How can line breaks be used strategically to enhance readability and user experience in C++ programs?
Handling User Input
- Creating variables in C++ is essential to storing user input effectively. Variables act as placeholders in the computer's memory (RAM) to store data provided by the user.
- Use
cin
to receive input from the user and assign it to the appropriate variable. Ensure that the data type of the variable matches the type of input expected (e.g., integer, string). - Curiosity Question: How does the computer allocate memory when creating variables to store user input in C++ programs?
Variable Operations
- Variables in C++ can be manipulated just like mathematical entities. Perform operations such as addition, subtraction, multiplication, and division on variables to achieve desired results.
- Experiment with different operations on variables to understand their behavior and impact on the final output.
- Curiosity Question: How can variable operations be utilized creatively to optimize code functionality and performance in C++ programs?
When diving into programming concepts such as input and output operations, remember to explore the practical implications and experiment with various scenarios to solidify your understanding. Stay curious and keep exploring the vast possibilities that C++ offers in the world of programming.
Additional Resources for Input and Output Operations in C++
- Article: C++ Input/Output: A comprehensive guide on basic input and output operations in C++.
- Book: "C++ Primer" by Stanley B. Lippman: A highly recommended book for mastering C++ programming, including input and output operations.
- Online Tutorial: C++ Input and Output Streams: An interactive tutorial on input and output streams in C++ programming.
- Video Tutorial: C++ Input and Output Streams: A video tutorial explaining various input and output operations in C++.
Explore these resources to deepen your understanding of input and output operations in C++ programming. Happy learning!
Practice
Task: Write a C++ program that asks the user for their name and age, and then prints a greeting message using their input.