Operators (arithmetic, relational, logical, bitwise)
This lesson covers the four main categories of operators in C++: arithmetic, comparison, logical, and bitwise operators.
Lets Go!
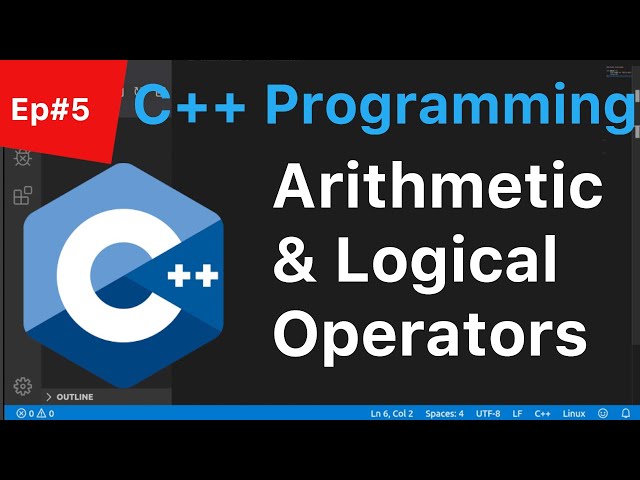
Operators (arithmetic, relational, logical, bitwise)
Lesson 8
Understand and use various operators in C++ to perform operations on variables.
Get Started 🍁Introduction to C++ Operators: A Comprehensive Overview
Welcome to the "Introduction to C++ Operators" course! In this course, we will delve into the fundamental aspects of C++ operators, covering key categories such as arithmetic, comparison, logical, and bitwise operators.
Have you ever wondered how mathematical and logical operations are performed within a program? Are you curious about the various types of operators available in C++ and how they can be used to manipulate values effectively?
Throughout this course, we will explore essential operators like addition, subtraction, multiplication, division, modulus, increment, decrement, comparison operators, logical operators (AND, OR, NOT), and bitwise operators (AND, OR, Left Shift, Right Shift).
By the end of this course, you will have a solid understanding of how to utilize different operators to perform complex operations and make your programs more efficient and dynamic. Join us on this journey to enhance your C++ programming skills and explore the world of operators!
Let's embark on this exciting learning experience together and unlock the power of C++ operators. Get ready to expand your programming knowledge and take your skills to the next level. Are you ready to dive in? Let's get started! 🚀
Main Concepts of C++ Operators
-
Arithmetic Operators
- Arithmetic operators in C++ are used to perform mathematical operations on values.
- The main arithmetic operators include: plus (+), minus (-), multiplication (*), division (/), modulus (%), increment (++), and decrement (--).
- For example, using these operators can help in adding, subtracting, multiplying, or dividing numbers in a program.
-
Comparison Operators
- Comparison operators are used to compare values and return a true or false result.
- Common comparison operators include: less than (<), greater than (>), less than or equal to (<=), greater than or equal to (>=), equal to (==), and not equal to (!=).
- These operators are useful for making decisions based on the relationship between two values in a program.
-
Logical Operators
- Logical operators help in combining multiple conditions or boolean values together.
- Important logical operators are: logical AND (&&), logical OR (||), and logical NOT (!).
- Logical operators are crucial for creating complex conditions in decision-making logic within a program.
-
Bitwise Operators
- Bitwise operators perform operations on binary digits at the bit level.
- Main bitwise operators include: bitwise AND (&), bitwise OR (|), bitwise left shift (<<), and bitwise right shift (>>).
- Bitwise operators are useful for manipulating individual bits within binary data in a program.
Practical Applications of C++ Operators
Arithmetic Operators
-
Addition Operator (+)
- Create two float variables,
value1
andvalue2
. - Initialize
value1
to 2 andvalue2
to 4. - Use the addition operator to add
value1
andvalue2
and display the result:cout << value1 + value2 << endl;
- Expected result: 6
- Create two float variables,
-
Subtraction Operator (-)
- Use the subtraction operator to subtract
value2
fromvalue1
:cout << value1 - value2 << endl;
- Expected result: -2
- Use the subtraction operator to subtract
-
Multiplication Operator (*)
- Use the multiplication operator to multiply
value1
andvalue2
:cout << value1 * value2 << endl;
- Expected result: 8
- Use the multiplication operator to multiply
-
Division Operator (/)
- Use the division operator to divide
value1
byvalue2
:cout << value1 / value2 << endl;
- Expected result: 0.5
- Use the division operator to divide
-
Modulus Operator (%)
- Create two integer variables,
value3
andvalue4
. - Initialize
value3
to 2 andvalue4
to 4. - Use the modulus operator to find the remainder of
value3
divided byvalue4
:cout << value3 % value4 << endl;
- Expected result: 2
- Create two integer variables,
-
Increment and Decrement Operator (++, --)
- Use the
++
operator to increment a value:++value1;
- Use the
--
operator to decrement a value:--value2;
- Use the
Comparison Operators
-
Less Than (<)
- Compare if
value3
is less thanvalue4
:cout << (value3 < value4) << endl;
- Expected result: 1 (True)
- Compare if
-
Greater Than (>)
- Compare if
value3
is greater thanvalue4
:cout << (value3 > value4) << endl;
- Expected result: 0 (False)
- Compare if
-
Equality Operators (<=, >=, ==, !=)
- Compare if
value3
is less than or equal tovalue4
:cout << (value3 <= value4) << endl;
- Compare if
value3
is greater than or equal tovalue4
:cout << (value3 >= value4) << endl;
- Compare if
value3
is equal tovalue4
:cout << (value3 == value4) << endl;
- Compare if
value3
is not equal tovalue4
:cout << (value3 != value4) << endl;
- Compare if
Logical Operators
-
Logical AND (&&)
- Create two boolean variables,
result1
andresult2
, and set them to true and false respectively. - Check if both
result1
andresult2
are true:cout << (result1 && result2) << endl;
- Expected result: 0 (False)
- Create two boolean variables,
-
Logical OR (||)
- Check if at least one of
result1
orresult2
is true:cout << (result1 || result2) << endl;
- Expected result: 1 (True)
- Check if at least one of
-
Logical NOT (!)
- Invert the value of
result1
:cout << !result1 << endl;
- Expected result: 0 (False)
- Invert the value of
Bitwise Operators (Left Shift, Right Shift, AND, OR)
- Experiment with bitwise operators by creating binary representations of numbers and applying operations like
&
,|
,<<
, and>>
.
Explore and Experiment!
Try out these examples in your own C++ environment to deepen your understanding of operators. Experiment with different values and combinations to solidify your knowledge. Happy coding!
Test your Knowledge
Which operator is used for addition in C++?
Which operator is used for addition in C++?
Advanced Insights into C++ Operators
Expanding on the fundamental concepts covered in the video, let's delve into more advanced insights into C++ operators. Understanding these advanced aspects can elevate your programming skills and problem-solving abilities.
Arithmetic Operators
In addition to the basic arithmetic operators like addition, subtraction, multiplication, division, modulus, and increment/decrement operators, we can explore more complex operations. For example, exploring operator precedence and how it impacts the outcome of expressions. Experiment with parentheses to alter the order of evaluation and observe the different results.
Curiosity Question: How does operator precedence affect the evaluation of complex arithmetic expressions in C++?
Comparison Operators
Moving beyond the commonly used less than, greater than, equal to, and not equal to operators, consider scenarios where you might need to combine multiple comparison operators for more intricate conditional statements. Experiment with nested conditions and chained comparisons to create versatile decision-making processes in your programs.
Curiosity Question: How can you optimize nested comparison operators for efficiency and readability in your code?
Logical Operators
Unveil the power of logical operators like &&
(AND), ||
(OR), and !
(NOT) in creating sophisticated control flow structures. Delve into short-circuit evaluation to improve the performance of your applications by minimizing unnecessary computations in conditional statements.
Curiosity Question: How can short-circuit evaluation enhance the efficiency of logical expressions in your C++ programs?
Bitwise Operators
For programmers seeking optimization and manipulation at the bit level, bitwise operators like AND, OR, left shift, and right shift provide a playground for creativity. Experiment with bitwise operations to encode and decode information, optimize memory usage, and implement complex algorithms efficiently.
Curiosity Question: What innovative applications can you develop using bitwise operators in C++ to address specific computational challenges?
By exploring these advanced insights into C++ operators, you can gain a deeper understanding of their functionalities and unleash your potential to craft robust and efficient solutions in your programming endeavors. Happy coding!
Additional Resources for C++ Operators
If you're looking to enhance your understanding of C++ operators, here are some additional resources to explore:
-
C++ Operator Overloading - This article delves into operator overloading in C++, providing examples and explanations to help you grasp this advanced topic.
-
Mastering C++ Operators - Tutorialspoint offers a comprehensive guide on various operators in C++, covering arithmetic, comparison, logical, and bitwise operators in detail.
-
C++ Programming: From Problem Analysis to Program Design - This book is a valuable resource for beginners as it covers fundamental concepts in C++ programming, including operators, with practical examples and exercises.
-
C++ Reference - The C++ reference website provides an extensive list of operators in C++ along with detailed explanations and usage examples.
Feel free to dive into these resources to deepen your knowledge of C++ operators and elevate your programming skills. Happy learning! 😊
Practice
Task: Write a C++ program to demonstrate the use of arithmetic, relational, logical, and bitwise operators with examples.