Type Modifiers
Data type modifiers in C++ are used to modify the length of a data type, allowing for increased or decreased storage capacity. These modifiers include signed, unsigned, long, and short, which can be applied to data types like int, char, and long.
Lets Go!
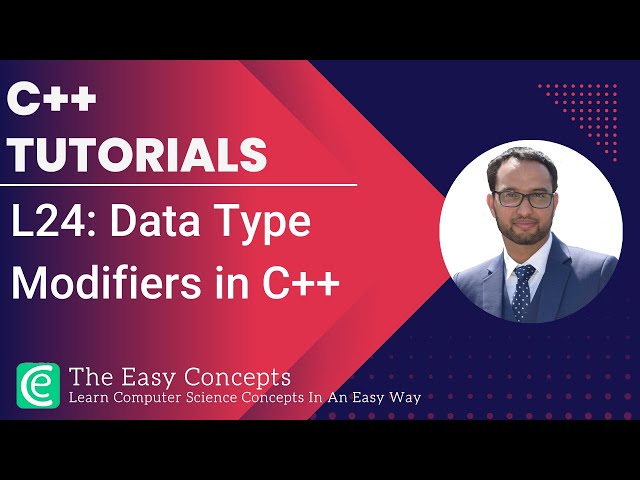
Type Modifiers
Lesson 7
Learn how type modifiers like short, long, signed, and unsigned are used to alter the properties of data types.
Get Started 🍁Introduction to Data Type Modifiers in C++
Welcome to the "Introduction to Data Type Modifiers in C++" course! In this course, we will delve into the world of data type modifiers in the C++ programming language.
In the previous tutorial, we explored data types in C++. Today, we will focus on data type modifiers, which are used to adjust the length of data that a specific data type can hold.
Have you ever wondered how to modify the size of data types in C++ to suit your needs? This course will equip you with the knowledge to do just that!
We will discuss key modifiers such as 'signed,' 'unsigned,' 'long,' and 'short,' and how they can be used with different data types like 'int,' 'char,' and more.
By understanding data type modifiers, you will have the power to manipulate the range and size of data types within your programs.
Moreover, we will explore practical examples and calculations to solidify your understanding of data type modifiers.
Are you ready to unlock the potential of data type modifiers in C++? Let's dive in and start exploring the fascinating world of data type manipulation in programming!
Main Concepts of Data Type Modifiers in C++
-
Data type modifiers are used to modify the length of data that a particular data type can hold in C++.
- For example, integers consist of four bytes in a 64-bit machine, but data type modifiers can increase or decrease this length.
-
Various data type modifiers include signed, unsigned, long, and short.
- These modifiers can be used with different data types like int, char, and long prefix to alter their length and range.
-
Example: When we specify
short int
, it is of 2 bytes in size in the same compiler. The range for a 2-byte signed integer is -32,768 to 32,767.- Using the unsigned prefix, we can change the range to start from 0 and go up to 65,535.
-
Range Calculation: The range of a data type can be calculated using the formula: (2 to the power n) - 1.
- For signed char, with 1 byte or 8 bits, the range is from -128 to 127. For unsigned char, the range is from 0 to 255.
-
Programming Exercise: To practice understanding data type ranges, users are encouraged to write a program that takes an input (such as number of bits) and calculates the range for signed and unsigned values.
- Follow the provided formula to derive the minimum and maximum values for the given number of bits.
-
Practice and Support: The video concludes with an invitation for users to try the programming exercise and seek help in the comments section. Users are encouraged to like, share, and subscribe for more tutorials on C++ concepts.
Practical Applications of Data Type Modifiers
Let's put our knowledge of data type modifiers into action by creating a simple C++ program to calculate the range of signed and unsigned values based on the number of bits provided.
Step 1: Create the Program
#include <iostream> #include <cmath> int main() { int numBits; std::cout << "Enter the number of bits: "; std::cin >> numBits; int minValueSigned = -pow(2, numBits - 1); int maxValueSigned = pow(2, numBits - 1) - 1; int maxValueUnsigned = pow(2, numBits) - 1; std::cout << "Range of signed value: " << minValueSigned << " to " << maxValueSigned << std::endl; std::cout << "Range of unsigned value: 0 to " << maxValueUnsigned << std::endl; return 0; }
Step 2: Compile and Run the Program
Compile the program using a C++ compiler (e.g., g++) and run it to input the number of bits you want to calculate the ranges for.
Step 3: Analyze the Output
After running the program, observe the ranges of signed and unsigned values calculated based on the number of bits you input.
Step 4: Experiment and Edit
Feel free to modify the program to explore different scenarios and gain a deeper understanding of how data type modifiers impact the range of values.
Give it a try and explore the ranges of signed and unsigned values in C++ based on the number of bits provided. Share your experience in the comments section and stay tuned for more tutorials! Happy coding!
Test your Knowledge
Which of the following is a type modifier in C++?
Which of the following is a type modifier in C++?
Advanced Insights into Data Type Modifiers in C++
In the previous tutorial, we discussed data types in C++. Now, let's delve into data type modifiers, which allow us to modify the length of data a particular data type can hold. This is essential for fine-tuning the storage capacity of variables in our programs.
Understanding Data Type Modifiers
Data type modifiers such as signed, unsigned, long, and short can be applied to data types like int, char, and long to adjust their lengths. For example, by using the 'short' modifier with 'int', we can reduce the size from 4 bytes to 2 bytes, impacting the range of values the variable can hold.
When we add the 'unsigned' modifier, it changes the range of possible values for a variable. For instance, 'unsigned short int' increases the range to go from 0 to 65,535 instead of having negative values included.
Calculating Data Type Ranges
To deeply understand the ranges of data types, we can apply mathematical formulas. For signed char, with 8 bits, we apply the formula -2^(n-1)
to determine the minimum value (e.g., -128), with a slight adjustment for unsigned char to cover a range from 0 to 255.
Understanding how bits play a crucial role in determining the range is fundamental when working with different data types. Breaking down the numbers into bits and applying the formulas adds a layer of comprehension to the behavior of data type modifiers.
Challenge: Creating a Range Calculator Program
To further solidify your grasp on data type modifiers, a challenge is presented. Create a program that takes the number of bits as input and calculates the range for both signed and unsigned values based on the provided formulas. This hands-on practice will enhance your understanding and application of the concepts discussed.
Curiosity Question
How does the utilization of data type modifiers impact memory utilization and program efficiency in real-world software development scenarios?
By exploring and experimenting with these concepts, you can deepen your expertise in utilizing data type modifiers effectively in your C++ programs, enhancing your programming skills.
Remember, practice and experimentation are key to mastering complex programming concepts. Happy coding!
Additional Resources for Data Type Modifiers
- C++ Data Types and Modifiers - GeeksforGeeks
- A Complete Guide to Data Type Modifiers in C++ - Tutorialspoint
- Understanding Data Types in C++ - YouTube Tutorial
Explore these resources to deepen your understanding of data type modifiers in C++. Enhance your knowledge and skills by delving into more detailed explanations and examples provided in these references. Happy learning!
Practice
Task: Write a C++ program demonstrating the use of signed and unsigned integers, showing the difference in their range.