Variables and data types
Variables in C++ act as placeholders to store values such as numbers or text. Data types, such as integer and double, define the kind of values that can be stored in a variable.
Lets Go!
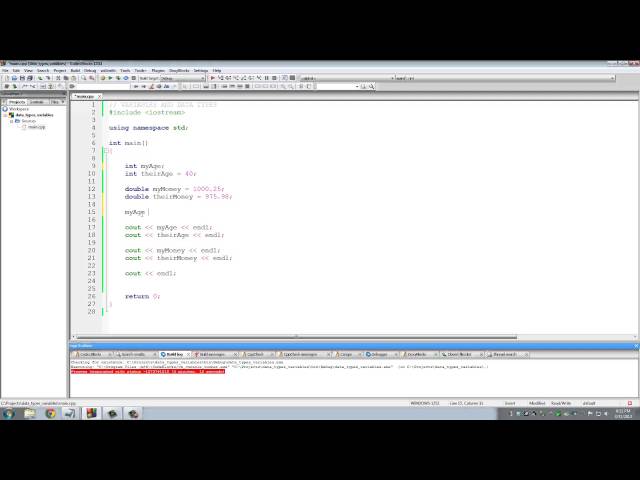
Variables and data types
Lesson 6
Understand the concept of variables, data types, and how they are used in C++ programming.
Get Started 🍁Introduction to Variables and Data Types in C++
Welcome to our course on "Introduction to Variables and Data Types in C++"! In this course, you will delve into the fundamental concepts of variables and data types in the programming language C++.
Variables play a crucial role in programming as they act as placeholders to store information that can be manipulated and processed by a computer. Just like how you store numbers in your memory before performing a calculation, variables in C++ store values like integers (whole numbers) or doubles (numbers with fractions).
Throughout this course, you will learn how to declare and initialize variables, explore the two most common data types (integer and double), and understand the syntax for creating variables in C++. By the end of this course, you will be equipped with the knowledge and skills to effectively utilize variables and data types in C++ programming.
But before we begin, here's a question to spark your curiosity: Why is it important to initialize variables in programming? Join us as we uncover the answer and embark on an exciting journey into the world of C++ variables and data types.
Are you ready to dive into the world of C++ programming? Let's get started!
Main Concepts of Variables and Data Types in C++
-
Variables: In computer programming, variables are placeholders that store values like numbers or text. Just like we store numbers in memory before processing them, variables in programming hold values that can be manipulated and used in calculations or output to the user.
-
Declaring Variables: To declare a variable in C++, you need to specify the data type (e.g., integer, double) and give it a name (e.g., my_age, their_money). This tells the computer what kind of value the variable will hold.
-
Data Types:
-
Integer: An integer data type is used for whole numbers like 1, 5, 10, or 1 million. Decimals are not considered integers, so numbers like 7.5 would not be suitable for an integer variable.
-
Double: The double data type can store fractional numbers like 0.5, 7.5, or 1.11. It allows for more precision in calculations involving decimal points.
-
-
Initializing Variables: When declaring a variable, you can also assign an initial value to it. This value can be zero, a specific number, or a text string depending on the type of data the variable will hold.
-
Assignment Operator: In programming, the equal sign (=) is used as an assignment operator to assign a value to a variable. For example,
my_age = 25
assigns the value 25 to the variable my_age. -
Best Practice: It is recommended to always initialize variables when declaring them to avoid unexpected results. Uninitialized variables may contain random values that can cause errors in more complex programs.
-
Variable Reusability: Variables can be reassigned new values during the execution of a program. This allows for the reuse of variables with different values in different parts of the code.
-
Syntax: The common syntax for declaring a variable includes specifying the data type, choosing a relevant name, and ending with a semicolon. For example,
int my_age;
declares an integer variable named my_age.
These main concepts provide the foundation for understanding how variables and data types work in C++ programming. By mastering these concepts, learners can effectively manage and manipulate data in their programs for various computational tasks.
Practical Applications of Variables and Data Types in C++
To get hands-on experience with declaring and initializing variables in C++, follow these steps:
-
Open a C++ IDE: Begin by opening a C++ integrated development environment (IDE) such as Visual Studio or Code::Blocks.
-
Declare Integer Variables:
- In your C++ code editor, type the following:
int myAge; int theirAge;
- These lines declare two integer variables,
myAge
andtheirAge
.
- In your C++ code editor, type the following:
-
Declare Double Variables:
- Next, add the following lines to declare two double variables:
double myMoney; double theirMoney;
- These lines create variables
myMoney
andtheirMoney
of type double.
- Next, add the following lines to declare two double variables:
-
Initialize Variables:
- Assign initial values to the variables right after declaration:
myAge = 25; theirAge = 40; myMoney = 1.25; theirMoney = 975.198;
- These assignments give initial values to each variable.
- Assign initial values to the variables right after declaration:
-
Print Variable Values:
- Use
cout
to display the values of the variables:cout << "My Age: " << myAge << endl; cout << "Their Age: " << theirAge << endl; cout << "My Money: $" << myMoney << endl; cout << "Their Money: $" << theirMoney << endl;
- These lines will output the values stored in the variables to the console.
- Use
-
Build and Run the Program:
- Save your code, build it to check for errors, and then run it to see the output.
-
Reassign Variables:
- Experiment by reassigning values to the variables within the program:
theirAge = 50;
- Reassigning values demonstrates the flexibility of variables in C++.
- Experiment by reassigning values to the variables within the program:
-
Explore Variable Initialization:
- Test the impact of not initializing a variable by commenting out the initialization:
//int myAge;
- Notice how the variable gets assigned a random value.
- Test the impact of not initializing a variable by commenting out the initialization:
-
Optimal Practice:
- Always initialize variables to prevent unexpected behavior in your programs:
int myAge = 0; int theirAge = 0;
- Always initialize variables to prevent unexpected behavior in your programs:
-
Iterate and Improve:
- Experiment with creating new variables, assigning values, and manipulating them in your program to solidify your understanding.
By following these steps, you can practice working with variables and data types in C++ and gain a deeper understanding of their practical applications. Remember to save your progress, build your code, and analyze the output to reinforce your learning. Enjoy exploring the world of variables and data types in C++! Give it a try and see the results for yourself. Happy coding!
Test your Knowledge
What is a variable in C++?
What is a variable in C++?
Advanced Insights into Variables and Data Types in C++
In programming, the concept of variables is crucial for storing and manipulating data. Understanding advanced aspects of variables and data types can enhance your coding skills. Let's delve deeper into this topic:
Declaring Variables:
- Initialization: When declaring variables, it is essential to assign an initial value. Failing to do so may result in the variable holding a random value, potentially causing errors.
- Reusing Variables: Variables can be reassigned new values, making them versatile and useful for iterative processes like loops.
Common Data Types:
- Integer (int):
- Represents whole numbers (e.g., 5, 10, 1000).
- Ideal for quantities that do not require fractional values.
- Double:
- Accommodates fractional numbers (e.g., 0.5, 7.5, 1.11).
- Offers precision for calculations involving decimals.
Tips and Recommendations:
- Initialization Best Practices: Always initialize variables to avoid unpredictable behavior. Use meaningful values or set them to zero if an initial value is unknown.
- Variable Naming: Choose descriptive names for variables to convey their purpose clearly, aiding in readability and maintenance.
Expert Advice:
- Variable Utilization: Understand the intended use of a variable before declaring it. Proper variable management enhances code efficiency and clarity.
Curiosity Question:
Why is initializing variables considered a good practice in programming, and how does it impact the reliability of your code?
By honing your understanding of variables and data types in C++, you can write more robust and efficient code. Remember to practice declaring, initializing, and utilizing variables effectively to master this fundamental aspect of programming. Keep exploring and experimenting with different data types to broaden your coding skills further.
Additional Resources for Variables and Data Types in C++
Here are some helpful resources to further enhance your understanding of variables and data types in C++:
- Article: Introduction to C++ Programming
- Video Tutorial: C++ Programming for Beginners
- Online Course: C++ Programming - From Beginner to Expert
These resources provide comprehensive explanations and examples to help you master the concepts discussed in this video. Happy learning!
Practice
Task: Write a C++ program to declare variables of different data types (int, float, char, and bool) and display their values.