Java Syntax and Program Structure
Java program structure is essential for writing any Java code. It consists of package declaration statements, import statements, class declarations, comments sections, main method, declarations, conditional blocks, loop blocks, and method declarations.
Lets Go!
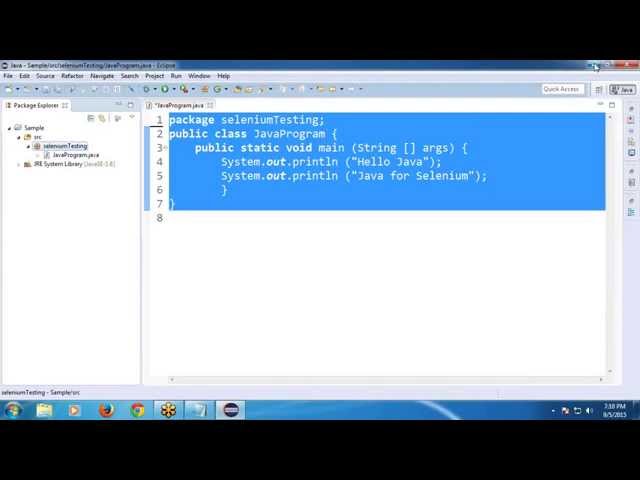
Java Syntax and Program Structure
Lesson 4
Understand the key components of Java syntax, including classes, methods, statements, and program structure.
Get Started 🍁Introduction to Java Programming
Welcome to the course "Introduction to Java Programming"! In this course, we will explore the foundational aspects of Java programming, from syntax to program structure. Whether you are a beginner looking to dive into the world of programming or someone with prior experience wanting to strengthen your Java skills, this course is designed to cater to learners of all levels.
Have you ever wondered how Java programs are structured or what syntax rules govern them? If so, this course is the perfect starting point for you. We will cover key topics such as package declaration, import statements, class declaration, comments, main method, declarations, conditional and loop blocks, method declarations, and much more.
By the end of this course, you will have a solid understanding of Java syntax rules and program structure, enabling you to write your own Java programs with confidence. So, are you ready to embark on this exciting journey into the world of Java programming? Let's get started!
Main Concepts of Java Program Structure
-
Package Declaration Statement
- The package statement declares a package name. It is automatically generated when you create a Java package. Packages help organize classes in Java code.
-
Import Statements
- Import statements are used to access built-in and user-defined libraries in Java. They allow you to use classes from those libraries in your program.
-
Class Declaration
- A class declaration in Java is surrounded by curly braces. It is the building block of Java programs and defines the structure and behavior of objects.
-
Comment Section
- Comments are optional but considered best practice in Java programming. They provide information and documentation about the code for better understanding.
-
Main Method
- The main method is where the execution of a Java program starts. It is a mandatory part of every Java program and is used to run the code.
-
Declarations
- In Java, you declare variables and constants to store and manipulate data. Variables can vary, while constants hold fixed values. Use final modifier to declare constants.
-
System.out.print
System.out.print
is used to display output in Java programs. It is a part of theSystem
class that allows you to print messages to the console.
-
Conditional Blocks
- Conditional blocks in Java, like
if-else
statements, allow you to execute certain code based on specified conditions. They help control the flow of the program.
- Conditional blocks in Java, like
-
Loop Blocks
- Loop blocks, such as
for
andwhile
loops, are used to iterate over a block of code multiple times. They help in executing repetitive tasks efficiently.
- Loop blocks, such as
-
Method Declarations
- Methods in Java define the actions or behaviors of objects. They are defined within classes and can be called to perform specific tasks as needed.
These main concepts form the foundation of Java program structure and syntax, enabling you to write and execute Java code effectively.
Practical Applications of Java Program Structure
Follow these steps to create a basic Java program using the structure and syntax explained in the video:
-
Package Declaration Statement:
- When starting a new Java program, include a package declaration statement.
- Use the format:
package [package_name];
- For example,
package selenium.testing;
-
Import Statements:
- Import built-in or user-defined libraries as needed.
- Use the format:
import [package].[class_name];
orimport [package].*;
to import all classes from a package.
-
Class Declaration:
- Define the main class of your program within the package.
- Use the format:
public class [class_name] { }
.
-
Comment Section:
- Add comments to your code for clarity and best practices.
- Use
//
to start a comment line.
-
Main Method:
- The main method serves as the entry point for your program.
- Define it with:
public static void main(String[] args) { }
.
-
Declarations:
- Declare variables and constants as needed in your program.
- Use
int
,String
, or other data types followed by the variable name.
-
System.out.println Statement:
- Use
System.out.println
to display messages or output from your program. - Format:
System.out.println("Your message here");
.
- Use
-
Conditional Blocks and Loop Blocks:
- Use
if
for conditional blocks andfor
,while
, ordo-while
for loop blocks. - Make sure to enclose blocks in curly braces
{ }
.
- Use
Try It Out:
- Start by creating a new Java file with the specified package and class structure.
- Import relevant libraries using the
import
statements. - Define variables, constants, and the
main
method in your class. - Add comments to describe the purpose of your code.
- Use conditional blocks and loop blocks to control the flow of your program.
- Display messages or results using
System.out.println
. - Ensure your program structure follows the syntax rules discussed.
By following these steps, you can build a simple Java program that demonstrates the Java program structure and syntax explained in the video. Take the opportunity to experiment with different elements and see how they impact the behavior of your program. Happy coding! 🚀
Test your Knowledge
What is the correct way to declare a class in Java?
What is the correct way to declare a class in Java?
Advanced Insights into Java Program Structure
In this section, we will delve deeper into the Java program structure and syntax, exploring advanced aspects that will enhance your understanding of programming in Java.
Main Method and Access Modifiers
One crucial element of a Java program is the main method. The main method serves as the entry point for program execution and is mandatory in every Java program. By understanding access modifiers like public
and non-access modifiers like static
, you can optimize your program's structure for efficient execution. Access modifiers define the accessibility of classes, methods, and variables, while non-access modifiers like static
allow you to invoke methods without the need for object instantiation.
Expert Tip: Utilize the main method efficiently to streamline program execution and leverage access modifiers to control the visibility and accessibility of your program components.
By delving into these advanced insights into Java program structure, you can elevate your programming skills and create more robust and efficient Java applications. Experiment with these concepts in your own programs to unlock the full potential of Java programming. Happy coding!
Additional Resources for Java Programming
Enhance your understanding of Java programming by exploring these additional resources:
-
Java Tutorial for Beginners: Official Java tutorials by Oracle to get started with Java programming.
-
Java Programming and Software Engineering Fundamentals: A comprehensive Coursera specialization covering Java programming and software engineering fundamentals.
-
Effective Java: Book by Joshua Bloch that provides insights on best practices and techniques for writing Java code efficiently.
-
Journal of Object Technology: An online journal featuring articles and research on object-oriented programming and Java technology.
Dive deeper into Java programming concepts and advance your skills with these valuable resources. Happy coding!
Practice
Task: Write a Java program with a class that includes at least one method. Ensure the program follows proper indentation and naming conventions.