Operators (Arithmetic, Relational, Logical, Bitwise)
Java operators are symbols used to perform operations on operands, such as arithmetic calculations, comparisons, and evaluations. They can be categorized into arithmetic, relational, logical, and conditional operators.
Lets Go!
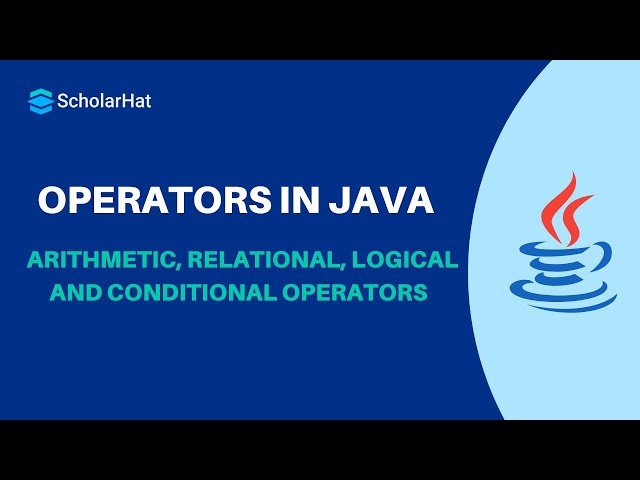
Operators (Arithmetic, Relational, Logical, Bitwise)
Lesson 6
Learn how to use different types of operators in Java to perform calculations, comparisons, and logical operations.
Get Started 🍁Introduction to Operators in Programming
Welcome to the course on "Introduction to Operators in Programming"! In this course, we will explore the fundamental concept of operators and how they are used in programming languages like Java.
Operators are symbols that perform various operations based on operands. They help in calculations, comparisons, and evaluations. Throughout this course, we will delve into different categories of operators including arithmetic, relational, logical, ternary, and unary operators.
Have you ever wondered how arithmetic operators like addition, subtraction, multiplication, and division are used in real-time scenarios? Or how relational operators help in decision making by comparing values?
Join us as we unravel the world of operators, understand their roles in programming, and explore practical examples to solidify your understanding. Let's embark on this exciting journey together!
Are you ready to dive into the world of operators and enhance your programming skills? Let's begin!
Main Concepts of Operators in Java
-
Operators: Symbols used to perform operations on operands.
-
Arithmetic Operators: Symbols like plus, minus, multiply, divide, and modulus used for calculations.
- Example provided for calculating discount percentage based on selling price and cost price.
-
Relational Operators: Symbols for comparisons like greater than, less than, greater than or equal to, less than or equal to, equal to, and not equal to.
- Used in decision-making applications to determine profit, loss, or no profit no loss scenarios.
-
Logical Operators: Used on Boolean expressions (and, or, not) to check if an expression is true or false.
- Example given for allowing a user to make a purchase only if certain conditions are met.
-
Conditional Operator (Ternary Operator): Assigns a value to an expression based on condition evaluation.
- Allows writing a single line of condition instead of if-else block.
- Example provided for showing a sign-in or sign-out button based on user authentication status.
Practical Applications of Conditional Operators
Let's dive into applying conditional operators in Java to make decisions in our code.
Step-by-Step Guide:
- Define the Problem: Imagine you need to write a program to check whether a salesperson has made a profit, a loss, or neither based on their selling price and cost price.
- Use Relational Operators:
int sellingPrice = 12000; int costPrice = 10000; if (sellingPrice > costPrice) { System.out.println("Profit"); } else if (costPrice > sellingPrice) { System.out.println("Loss"); } else { System.out.println("No Profit, No Loss"); }
- Run the Program: See the output based on the conditions you set for selling price and cost price. Try changing the values to see different results.
Hands-On Task:
- Define your own values for
sellingPrice
andcostPrice
. - Run the program and observe the outcome based on the relational operators used in the conditions.
Give it a try and see how relational operators can help you make decisions in your Java programs!
Test your Knowledge
What will be the output of the following code?
int a = 5, b = 3;
System.out.println(a & b);
What will be the output of the following code?
int a = 5, b = 3; System.out.println(a & b);
Advanced Insights into Java Operators
Operators in Java play a crucial role in performing various operations on operands. Beyond the basic arithmetic, relational, logical, ternary, and unary operators, let's delve into more advanced insights into how operators can be leveraged for more complex tasks.
Tips and Recommendations:
- Formatting Outputs: Utilizing number formats like percent iteration helps in presenting results more clearly and professionally.
- Nesting Conditions: Nested conditions can be used to handle multiple scenarios within a single operation effectively.
- Boolean Interpretation: Understanding how Boolean expressions are used in operators is key to accurate comparisons and evaluations.
Expert Advice:
- Efficiency is Key: Efficient use of operators not only enhances code readability but also improves the performance of the program.
- Practice Makes Perfect: Regular practice with different operator types is essential to gain mastery and fluency in Java programming.
Explore these advanced insights further to enhance your grasp of Java operators and elevate your programming skills!
Additional Resources for Operators in Java
- Article: Understanding Java Operators
- Reference Book: "Java: The Complete Reference" by Herbert Schildt
- Online Course: Java Operators Tutorial on YouTube
- Practice Problems: HackerRank Java Operator Challenges
Explore these resources to gain a deeper understanding of operators in Java and enhance your programming skills. Happy coding!
Practice
Task: Write a Java program that uses arithmetic, relational, logical, and bitwise operators to perform operations and print the results.