Variables, Data Types, and Type Casting
Java variables are containers used to store values with specific data types. Different data types have different memory sizes and ranges of values that can be stored. Declaring variables involves specifying the type and name of the variable, followed by optional assignment. Type casting allows for converting between different data types.
Lets Go!
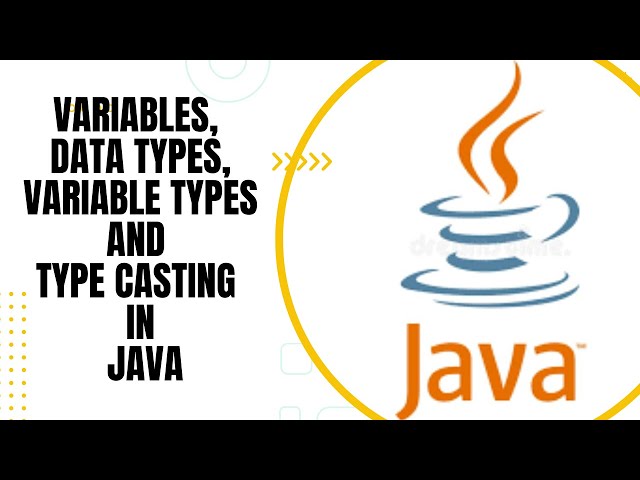
Variables, Data Types, and Type Casting
Lesson 5
Understand how to declare and initialize variables, use different data types, and perform type casting in Java.
Get Started 🍁Introduction to Java Variables
Welcome to the course on Variables! Have you ever wondered how programming languages like Java handle storing data? Well, in this course, we will delve into the concept of variables and how they are used to store values in memory.
Variables play a crucial role in programming as they act as containers for holding different types of data. In Java, each variable has a specific type that determines the kind of data it can store. By understanding variables, you will be able to efficiently manage and manipulate data in your programs.
Throughout this course, we will cover key topics such as:
- The different types of variables in Java
- How to declare and assign values to variables
- Exploring primitive and non-primitive data types
- Understanding type casting and its importance in programming
Are you ready to embark on a journey into the world of variables and data storage? Join us as we unravel the mysteries of variables in Java and pave the way for creating efficient and effective programs! Let's dive in and discover the power of variables together
Main Concepts of Variable Declaration and Type Casting
-
Variables: Variables are containers used to store values in memory. In Java, variables have specific types and data types that determine the size and layout in memory.
-
Data Types: Variables can hold values of different data types such as string, integer, and float, which determine the range of values that can be stored and the memory space allocated to the variable.
-
Variable Declaration: To declare a variable in Java, the syntax is
type varName = value
. Multiple variables of the same type can be declared in a single line using commas. -
Final Keyword: The
final
keyword is used to declare constants, meaning the value of the variable cannot be changed once assigned. -
Variable Naming Rules: Variable names must begin with a letter, can start with a lower case letter, dollar sign, or underscore, and cannot contain white spaces. Names are case sensitive and cannot be reserved words or special characters.
-
Primitive Data Types: Java has primitive data types such as byte, short, int, long, char, float, and double, each with a specific range of values and memory allocation.
-
Type Casting: Type casting is the process of converting one data type to another. It can be done through widening (automatic conversion from smaller to larger type) or narrowing (explicit conversion from larger to smaller type).
-
Narrowing Type Casting: In narrowing type casting, a larger data type is converted to a smaller data type, and decimal points are truncated if needed.
-
Widening Type Casting: In widening type casting, a smaller data type is automatically converted to a larger data type without the need for explicit casting.
By understanding these main concepts of variable declaration and type casting in Java, you can effectively create and manipulate different types of data in your programs.
Practical Applications of Variables
Variables are essential in programming as they allow us to store and manipulate data in memory. Let's explore how to declare variables, assign values, and perform type casting in Java.
Step-by-Step Guide
-
Declare Variables
- To declare a variable, use the syntax
type varName = value;
. - Example:
int myFirstVariable = 4;
. - You can declare multiple variables of the same type in a single line using commas.
- To declare a variable, use the syntax
-
Assign Values to Variables
- To assign different values to the same type of variables, simply use the variable name followed by
=
and the new value. - Example:
int mySecondVariable = 7, myThirdVariable = 9;
- To assign different values to the same type of variables, simply use the variable name followed by
-
Type Casting
- Type casting is the process of converting one data type to another.
- Narrow Type Casting: Converting from a larger type to a smaller type, e.g.,
double
toint
.- Change
double
toint
with potential loss of precision:int myInt = (int) myDouble;
.
- Change
- Widen Type Casting: Converting from a smaller type to a larger type, done automatically.
- Assign
int
todouble
without explicit casting:double myDouble = myInt;
.
- Assign
Let's Try It Out!
- Declare an
int
variable calledmyNumber
and assign it a value of your choice. - Declare a
double
variable calledmyDecimal
and assign it a decimal number. - Convert
myDecimal
to anint
using narrow type casting and store the result in a newint
variable. - Print out both
myNumber
and the converted value ofmyDecimal
to see the difference.
Feel free to experiment with different data types and values to solidify your understanding of variables and type casting in Java. Happy coding! 🚀
Test your Knowledge
What is the correct syntax to declare an integer variable in Java?
What is the correct syntax to declare an integer variable in Java?
Advanced Insights into Variables
In Java, variables serve as containers that store values in memory. These values are associated with specific data types, determining the size and layout of memory allocation. When declaring variables, it is crucial to consider the type of variable as it dictates the memory space allocated.
Tips and Recommendations:
- When declaring multiple variables of the same type, use a single line with comma separation for concise code.
- Utilize the
final
keyword to declare unchangeable variables, ensuring the assigned value remains constant. - Follow naming conventions for variables, such as starting with a lowercase letter, avoiding white spaces, and using dollar sign or underscore if needed.
Curiosity Question:
What are the implications of using the final
keyword to declare variables in Java?
Diving deeper into data types, primitive data types in Java are categorized as numeric (character, integer) and non-numeric. Understanding the memory space allocated for each data type is crucial when assigning values to variables.
Expert Advice:
- Be mindful of type casting when converting data types, as it can lead to information loss in narrowing type casting.
- Utilize widening type casting for automatic conversion of smaller data types to larger ones without explicit declarations.
By exploring variable declaration, data types, and type casting intricacies, you can enhance your understanding of Java programming and optimize memory utilization in your code. Experiment with different data types and casting operations to strengthen your grasp of Java variables.
Additional Resources for Java Variables:
Articles:
- Understanding Java Variables and Data Types
- Type Casting in Java
- Java Primitive Data Types Explained
Online Courses:
Books:
- "Java: A Beginner's Guide" by Herbert Schildt
- "Effective Java" by Joshua Bloch
Explore these resources to enhance your understanding of Java variables and dive deeper into the topic. Happy learning! 📚💻
Practice
Task: Write a program that declares variables of different data types (e.g., int, double, char) and demonstrates implicit and explicit type casting.