Writing Your First Java Program: 'Hello, World!'
This tutorial provides a step-by-step guide on creating a basic 'Hello World' Java application using IntelliJ IDEA. The tutorial covers setting up the project, creating packages and classes, defining the main method, and running the application.
Lets Go!
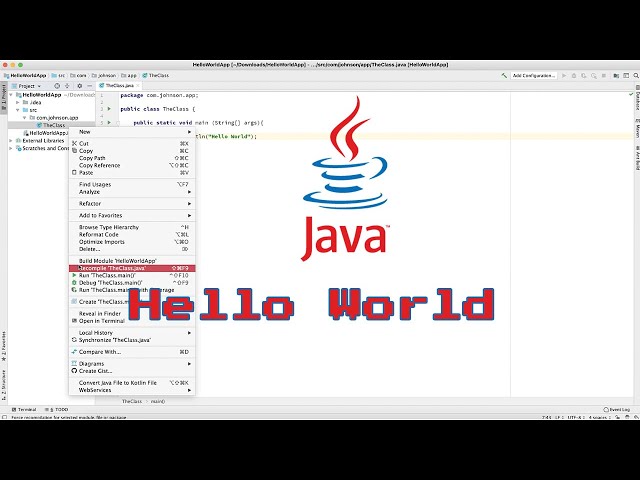
Writing Your First Java Program: 'Hello, World!'
Lesson 3
Learn how to create your first Java application with this comprehensive tutorial. Follow along to understand the basics of Java programming and get started with coding.
Get Started 🍁Introduction to Java Programming
Welcome, aspiring programmers! Are you ready to dive into the world of Java application development? In this course, "Introduction to Java Programming," you will embark on a journey to create your very first Java application.
Java is a versatile and powerful programming language used in a wide range of applications, from web development to mobile app creation. By the end of this course, you will have a solid understanding of Java fundamentals and be able to build your own applications.
Before we get started, have you ever wondered how programming languages like Java work behind the scenes? Do you have a passion for problem-solving and logical thinking? If so, this course is perfect for you!
To begin, ensure you have your favorite Integrated Development Environment (IDE) ready. Whether you prefer IntelliJ, Eclipse, or another IDE, make sure to set up your project with the necessary configurations to kickstart your Java coding journey.
So, are you ready to write your first "Hello World" application in Java and take your first step into the world of programming? Let's dive in and explore the exciting world of Java together!
Main Concepts of Java Programming
-
Setting Up a Java Project: In order to create a Java application, you need to first install or open up an Integrated Development Environment (IDE) like IntelliJ or Eclipse. Within your IDE, you can create a new project, specify the project SDK (in this case, Java 1.8), and name your project.
-
Creating Packages: Packages in Java act as folders to organize classes, interfaces, etc. They help prevent conflicts or collisions in your code. By creating a package within the source folder, you can group related classes together for better organization.
-
Declaring Classes and Methods: In Java, classes are blueprints for objects, while methods are blocks of code that can be executed. Classes are declared using the keyword "class", and methods like the main method can be created within classes to specify the starting point of execution.
-
Understanding Main Method: The main method is where Java applications begin execution. It is a required entry point for a Java program. By defining a main method with the signature
public static void main
, you indicate where the program should start running. -
Using Print and Println: In Java, you can display output to the console using
System.out.print
orSystem.out.println
. The former prints the text without moving to the next line, while the latter also includes a newline character at the end of the output. -
Running a Java Application: To run your Java application, you can right-click on the class file and select the main method to execute. This will display the output of your program in the console.
-
Future Learning: The video mentions that future videos will cover more in-depth explanations of Java keywords, terminology, and concepts. As you progress in your Java programming journey, you'll explore topics like access modifiers (
public
,static
), data types, control structures, and more.
By grasping these main concepts presented in the video, you are on your way to understanding the fundamentals of Java programming and building your first Java application successfully.
Practical Applications of Java
Ready to create your very first Java application? Follow these steps to write a basic "Hello World" program:
-
Open your favorite IDE: Install or open up IntelliJ IDEA, Eclipse, or any IDE of your choice.
-
Create a new project:
- Click on "Create New Project" in IntelliJ.
- Choose Java and select 1.8 for the project SDK.
- Name your project (e.g., "HelloWorldApp") and click finish.
-
Create a package:
- In the source folder, right-click, go to "New," and click on "Package."
- Create a package name (e.g., com.johnson.app).
-
Create a Java class:
- Right-click on the package, go to "New," and click on "Java Class."
- Name your class (e.g., "Main").
-
Add a main method:
- Type
public static void main(String[] args)
inside the Main class.
- Type
-
Write your code:
- Inside the main method, type
System.out.println("Hello, World!");
to print "Hello, World!". - Remember to end the statement with a semicolon.
- Inside the main method, type
-
Run your program:
- Right-click on the Main class file and select "Run Main."
- Check the output console to see "Hello, World!" printed.
Congratulations! You've just run your first Java application. Stay tuned for future videos where we dive deeper into Java programming concepts. Now, give it a try and enjoy coding!
Test your Knowledge
Which keyword is used to define the entry point of a Java application?
Which keyword is used to define the entry point of a Java application?
Advanced Insights into Java Application Development
Now that you've created your first Java application following the introductory steps, let's delve into some advanced insights into Java development.
Package Structure
- Packages act as containers for your classes and interfaces, allowing you to organize your code effectively.
- Choosing meaningful package names can prevent naming collisions in larger projects.
- Consider creating a nested package structure to further organize your code for better maintainability.
Tip: When naming packages, adhere to industry-standard conventions like reverse domain naming (e.g., com.companyname.projectname) for better clarity and consistency.
Main Method
- The
main
method is the entry point for any Java application, serving as the starting point for execution. - Understanding the
public static void main(String[] args)
signature is crucial for launching your application successfully. - By passing command-line arguments through
args
, you can enhance the flexibility and functionality of your Java program.
Expert Advice: Practice writing robust main
methods with error handling and argument parsing to handle various scenarios efficiently.
Output Formatting
- In Java, you can use
System.out.println()
for printing output with a newline character at the end, improving readability. - Experiment with different output formatting techniques, such as concatenation and formatting strings using placeholders for dynamic content.
By exploring these advanced concepts in Java application development, you can elevate your coding skills and create more sophisticated projects. Stay curious and keep practicing to unlock the full potential of Java programming. Happy coding!
Additional Resources for Java Programming
- Article: Java Programming Basics
- Video Tutorial Series: Java Programming for Beginners
- Online Course: Introduction to Java Programming
These resources will help you further understand Java programming concepts and enhance your skills. Dive deeper into the world of Java with these valuable references. Happy coding!
Practice
Task: Write a Java program that prints 'Hello, World!' to the console. Compile and run it to verify that it works as expected.