Accessing Nested Objects
This tutorial covers the concept of accessing nested objects in JavaScript, where objects are nested within each other to store properties and values. The video demonstrates how to access these nested values using dot notation and bracket notation.
Lets Go!
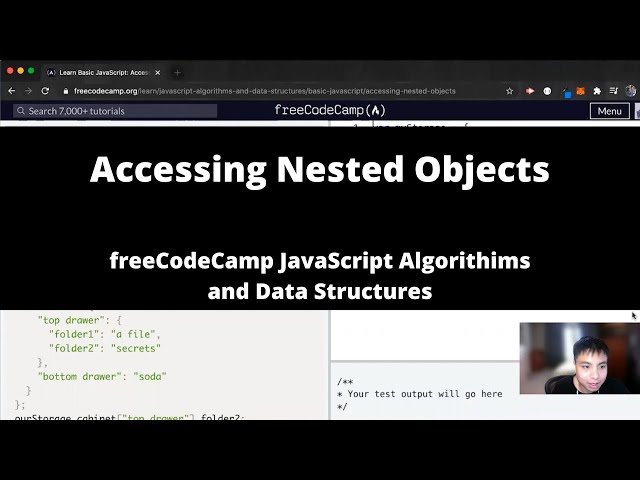
Accessing Nested Objects
Lesson 10
Learn how to access and modify properties in nested objects.
Get Started 🍁Welcome to Introduction to Accessing Nested Objects in JavaScript!
Are you ready to dive into the world of nested objects and arrays in JavaScript? In this course, we will explore how to access values within objects and properties within nested objects, using both dot notation and bracket notation.
Throughout the course, we will learn how to navigate through nested objects and arrays, unraveling the layers to access specific values. We will also understand the concept of nested objects and arrays, where objects or arrays are contained within one another.
By the end of this course, you will have a solid grasp on how to access nested objects and arrays efficiently, empowering you to work with complex data structures in your JavaScript projects.
Now, are you curious to uncover the secrets hidden within nested objects? Let's get started!
Main Concepts of JavaScript Nested Objects Tutorial
-
Nested Objects: In JavaScript, nested objects refer to objects that are nested within other objects. This means that an object can contain properties that are also objects themselves.
-
Accessing Nested Objects: To access values within nested objects, you need to use dot notation or bracket notation. Dot notation is used to access properties directly, while bracket notation is used when the property name is dynamic or contains special characters.
-
Example: In the video, an object
myStorage
is provided with nested properties likecar
,inside
, andglove box
. To access the contents of theglove box
, you would use dot notation likemyStorage.car.inside['glove box']
. -
Using Dot Notation vs. Bracket Notation: When accessing nested objects, try to use dot notation as it provides a cleaner and more readable code. However, if the property name requires special characters or is dynamic, then bracket notation should be used.
-
Assignment: The exercise in the video involves assigning the contents of the
glove box
to a variable. This is done by navigating through the nested objects using the correct notation.
By understanding how to access and work with nested objects in JavaScript, you can effectively manage and manipulate complex data structures in your code. Experimenting with different notations and practicing accessing nested objects will solidify your understanding of this concept.
Practical Applications of Accessing Nested Objects
In this tutorial, we learned how to access values within nested objects using JavaScript.
Step-by-Step Guide:
- Identify Nested Object Structure:
const myStorage = { car: { inside: { glove box: "maps" } } };
- Access Nested Value:
const gloveBoxContents = myStorage.car.inside["glove box"]; console.log(gloveBoxContents); // Output: "maps"
- Actionable Task:
- Access the contents of the glove box in the
myStorage
object. - Utilize dot notation for properties where possible, otherwise use bracket notation.
- Access the contents of the glove box in the
Try It Out:
- Open your browser's console or a code editor.
- Create a JavaScript file or use a code snippet tool.
- Copy and paste the provided object structure.
- Access the glove box contents using the given step-by-step guide.
- See the output "maps" displayed in the console.
Get Hands-On:
Go ahead and try accessing nested objects in JavaScript using the steps provided above. Experiment with different object structures and access other nested values to solidify your understanding. Remember to observe the syntax closely and practice accessing nested objects to enhance your skills. Happy coding!
Test your Knowledge
How do you access the math property in the following object?**
let student = {
grade: {
math: 90,
science: 85 }
}
How do you access the math property in the following object?**
let student = { grade: { math: 90, science: 85 } }
Advanced Insights into JavaScript Nested Objects
In JavaScript, nested objects refer to having objects within objects, presenting a hierarchy of properties and values. Accessing these nested objects can be achieved through a combination of dot notation and bracket notation.
When dealing with nested objects, it is crucial to understand how to access specific properties and values within them. For instance, if we have an object myStorage
with various nested properties, we can access a value like "maps" inside the "glove box" by drilling down using dot and bracket notations like myStorage.car.inside["glove box"]
.
One important tip to remember is to use quotations when using bracket notation to access properties, ensuring the code is interpreted correctly. Additionally, logging the accessed values can help verify if the correct information is retrieved.
Curiosity Question: How can you effectively handle deep nesting in JavaScript objects to maintain readability and optimize performance?
By mastering the technique of accessing nested objects in JavaScript, you can navigate complex data structures with ease and unlock the full potential of your code. Keep practicing and experimenting to enhance your proficiency in handling nested objects effectively.
Additional Resources for JavaScript Nested Objects
- Article: Understanding Nested Objects and Arrays in JavaScript
- Video Tutorial: Working with Nested Objects in JavaScript
- Interactive Practice: Nested Objects Exercise on Codecademy
Explore these resources to further enhance your understanding of accessing nested objects in JavaScript. Happy coding!
Practice
Task: Create an object student with nested properties: personalInfo containing name and age, and grades containing math, science, and english.
Task: Access the math grade using dot notation. Modify the science grade.
Task: Log the student's name and all grades to the console.