Accessing Object Properties
Learn how to access the properties of objects in JavaScript using dot notation. This video tutorial covers the basics of accessing and saving object properties into variables.
Lets Go!
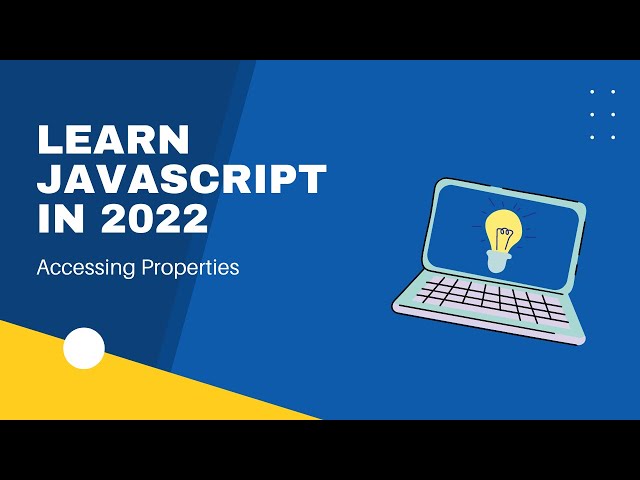
Accessing Object Properties
Lesson 9
Understand how to access object properties using dot notation and bracket notation.
Get Started 🍁Introduction to JavaScript Objects
Welcome to "Introduction to JavaScript Objects"! In this course, we will dive into the fascinating world of objects in JavaScript. Objects are a fundamental part of JavaScript and understanding how to work with them is essential for any aspiring coder.
In this course, we will explore various ways to access and manipulate properties within objects. One of the primary methods we will cover is using dot notation, which allows us to access specific properties of an object by appending them to the object name with a dot.
Have you ever wondered how to access and extract valuable information stored within objects? If so, this course is perfect for you!
By the end of this course, you will be able to confidently access properties within objects using dot notation and even save them into variables for further use. So, if you are ready to enhance your JavaScript skills and unlock the power of objects, let's get started! Get ready to level up your coding expertise and embark on an exciting journey into the world of JavaScript objects. Let's begin!
Main Concepts of JavaScript Objects:
-
Dot notation: Dot notation is a way to access properties and methods of objects in JavaScript. By using dot notation, you can access specific properties of an object by typing the object's name followed by a dot and then the property name. For example,
object.property
is how you access a property using dot notation. -
Accessing properties: When using dot notation to access properties of an object, you can retrieve the values associated with those properties. For instance, if we have an object called
spaceship
with a propertycolor
set tosilver
, we can access this property by typingspaceship.color
, which will return the valuesilver
. -
Handling non-existent properties: If you try to access a property that does not exist on an object using dot notation, the value
undefined
will be returned. This is important to keep in mind when working with objects and accessing their properties. -
Saving properties into variables: One of the benefits of accessing properties using dot notation is that you can save those properties into variables. This allows you to store and manipulate specific values associated with an object's properties.
-
Practice with dot notation: Practicing using dot notation to access properties of objects is essential for mastering this concept. By working through exercises that require accessing and storing property values using dot notation, you can solidify your understanding and apply it to real-world scenarios in JavaScript programming.
By understanding and practicing these concepts related to accessing properties of objects using dot notation, you can enhance your skills in JavaScript programming and effectively work with objects in your code.
Practical Applications of Accessing Properties in JavaScript Objects
In this section, we will learn how to access properties inside an object using dot notation. Let's dive in and practice accessing properties of an object.
-
Accessing a Specific Property:
- Create a new object with properties. For example:
let spaceship = { homePlanet: 'Earth', color: 'silver' };
- To access the value of a specific property, use dot notation. For example:
console.log(spaceship.homePlanet); // Output: Earth console.log(spaceship.color); // Output: silver
- Create a new object with properties. For example:
-
Practical Task - Practice with Dot Notation:
- In the code editor, create a variable
crewCount
and assign thenumCrew
property of thespaceship
object to it.let crewCount = spaceship.numCrew; console.log(crewCount); // Output: (value of numCrew)
- Next, create a variable
planetArray
and assign theflightPath
property of thespaceship
object to it.let planetArray = spaceship.flightPath; console.log(planetArray); // Output: (value of flightPath)
- In the code editor, create a variable
-
Summary:
- By practicing with dot notation, you can easily access and manipulate properties within an object.
- Feel free to experiment with different properties and objects to deepen your understanding.
Take a moment to try these tasks yourself in your own JavaScript environment. Understanding how to access properties in objects is a fundamental concept in JavaScript, and mastering it will enhance your coding skills. Have fun exploring and applying these concepts!
Test your Knowledge
How do you access the name property of an object named user?
How do you access the name property of an object named user?
Advanced Insights into Objects in JavaScript
In the realm of JavaScript objects, there are advanced techniques beyond basic property access using dot notation. Let's delve into some deeper insights to enhance your understanding:
Chaining Properties
One interesting aspect of accessing object properties is the ability to chain multiple properties together. For example, you can access nested properties by using multiple dot notation sequences. This allows you to dig deep into complex nested objects to retrieve specific values.
Tip: Keep in mind to ensure each property in the chain exists; otherwise, you might encounter errors.
Curiosity Question: Can you think of a real-life analogy that showcases the concept of chaining properties in JavaScript objects?
Computed Property Names
Another advanced feature in JavaScript objects is the use of computed property names. This technique involves dynamically computing property names at runtime, allowing for more dynamic and flexible object manipulation.
Recommendation: Experiment with using variables or expressions to compute property names when interacting with objects.
Curiosity Question: How can computed property names enhance the efficiency and flexibility of your JavaScript code?
By exploring these advanced insights into working with objects in JavaScript, you can elevate your programming skills and tackle more complex data structures effectively. Keep practicing and experimenting to master the intricacies of object manipulation. Happy coding! 🚀
Additional Resources for JavaScript Objects
-
MDN Web Docs - Working with Objects: A comprehensive guide on working with objects in JavaScript, including accessing properties using dot notation.
-
JavaScript.info - Objects and Classes: An in-depth tutorial on JavaScript objects, covering various ways to access and manipulate properties.
-
FreeCodeCamp - JavaScript Object Basics: A beginner-friendly guide to understanding JavaScript objects and how to work with them effectively.
-
Codecademy - JavaScript Objects Course: Dive deeper into the objects section on Codecademy to expand your knowledge on accessing and manipulating properties using dot notation.
Explore these resources to enhance your understanding of JavaScript objects and how to effectively access properties within them. Happy coding! 🚀
Practice
Task: Write a program to create an object representing a book with properties title, author, and yearPublished. Log the title using dot notation and the yearPublished using bracket notation.
Task: Modify the author property and add a new property genre to the book object.