Array Methods (Push, Pop, Shift & Unshift)
This video tutorial focuses on how to add elements to the beginning and end of an array, as well as how to remove elements from those positions.
Lets Go!
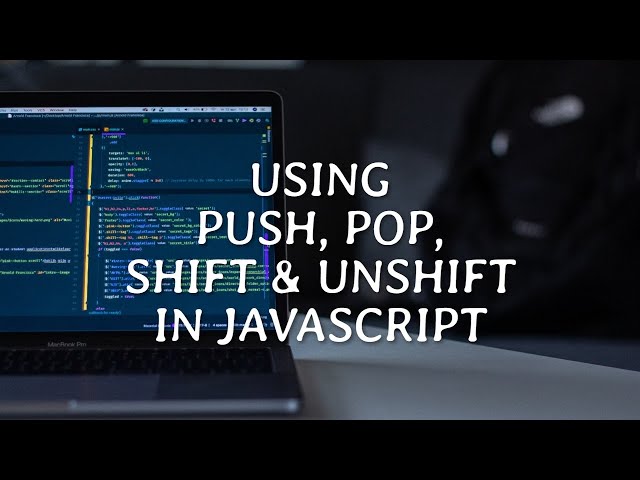
Array Methods (Push, Pop, Shift & Unshift)
Lesson 12
Learn how to use push, pop, shift, and unshift methods to manipulate arrays.
Get Started 🍁Welcome to Introduction to Array Manipulation
Are you ready to delve into the world of manipulating arrays? In this course, we will explore essential concepts such as adding elements to the beginning and end of an array, as well as removing elements from these positions. Array manipulation is a crucial skill for any programmer, and in this course, we will focus on the less known methods like shift
and unshift
.
Have you ever wondered how to effortlessly modify the contents of an array? Join us on this exciting journey as we uncover the ins and outs of array manipulation.
Let's start by adding an element to the end of an array, then move on to adding an element to the beginning. Through hands-on examples and explanations, you will gain a deeper understanding of how these methods work.
No prior knowledge is required to join this course - just be curious and eager to learn! Get ready to enhance your programming skills and become a master of array manipulation techniques. Are you ready to unlock the secrets of arrays? Let's get started!
Main Concepts of Array Manipulation
-
Adding Elements to an Array:
- To add an element to the end of an array, you can use the
push
method. This adds the element to the end of the array, increasing its length by one. - Example: If we have an array
[2, 3, 4, 5, 6]
, and we usepush(7)
, the array will now be[2, 3, 4, 5, 6, 7]
.
- To add an element to the end of an array, you can use the
-
Adding Elements to the Beginning of an Array:
- To add an element to the beginning of an array, you can use the
unshift
method. This adds the element to the beginning of the array, shifting all other elements to the right. - Example: If we have an array
[2, 3, 4, 5, 6, 7]
, and we useunshift(1)
, the array will now be[1, 2, 3, 4, 5, 6, 7]
.
- To add an element to the beginning of an array, you can use the
-
Removing Elements from the End of an Array:
- To remove an element from the end of an array, you can use the
pop
method. This removes the last element from the array, decreasing its length by one. - Example: If we have an array
[1, 2, 3, 4, 5, 6, 7]
, and we usepop()
, the array will now be[1, 2, 3, 4, 5, 6]
.
- To remove an element from the end of an array, you can use the
-
Removing Elements from the Beginning of an Array:
- To remove an element from the beginning of an array, you can use the
shift
method. This removes the first element from the array, shifting all other elements to the left. - Example: If we have an array
[1, 2, 3, 4, 5, 6]
, and we useshift()
, the array will now be[2, 3, 4, 5, 6]
.
- To remove an element from the beginning of an array, you can use the
By understanding these basic array manipulation methods like push
, unshift
, pop
, and shift
, you can effectively add or remove elements to or from the beginning and end of an array in JavaScript.
Practical Applications of Array Manipulation
Array manipulation is an essential concept in programming. In this tutorial, we learned how to add elements to the beginning and end of an array using push
and unshift
, as well as how to remove elements from the beginning and end of an array using pop
and shift
.
Step-by-Step Guide:
-
Adding an Element to the End of the Array:
- Start by creating an array of numbers (e.g.,
let arr = [2, 3, 4, 5, 6];
). - Use the
push
method to add an element to the end of the array:arr.push(7);
- Test the updated array by logging it to the console:
console.log(arr);
- Start by creating an array of numbers (e.g.,
-
Adding an Element to the Beginning of the Array:
- After adding the element to the end of the array, use the
unshift
method to add an element to the beginning:arr.unshift(1);
- Verify the updated array by console logging it:
console.log(arr);
- After adding the element to the end of the array, use the
-
Removing an Element from the End of the Array:
- To remove an element from the end of the array, use the
pop
method:arr.pop();
- Check the modified array:
console.log(arr);
- To remove an element from the end of the array, use the
-
Removing an Element from the Beginning of the Array:
- Finally, remove an element from the beginning of the array with the
shift
method:arr.shift();
- See if the array is back to its original state:
console.log(arr);
- Finally, remove an element from the beginning of the array with the
Get Hands-On:
Now is the perfect time to try these array manipulation methods in your own code. Create an array, follow the steps above, and see how you can add and remove elements easily. Don't forget to experiment and explore further possibilities with array manipulation. Happy coding!
Test your Knowledge
What does the pop() method do?
What does the pop() method do?
Advanced Insights into Array Manipulation
Array manipulation is a fundamental skill in programming, but there are some lesser-known methods that can be incredibly useful. In this section, we will delve into more advanced aspects of adding and removing elements from the beginning and end of an array.
Adding Elements to the End and Beginning
In the tutorial, we learned how to add elements to the end of an array using the push
method and to the beginning using the unshift
method. These methods are efficient ways to expand an array and maintain the order of its elements.
Removing Elements from the End and Beginning
Similarly, removing elements from the end of an array can be done with the pop
method, and from the beginning using the shift
method. Understanding when to use these operations can make your code more concise and efficient.
Expert Tip
It may seem odd that the methods for adding and removing elements from the front of an array are named shift
and unshift
. This serves as a reminder to approach coding with an open mind and be willing to adapt to different naming conventions.
Curiosity Question
Have you ever considered why these specific methods are named as they are? How would you design a method for adding or removing elements from the front of an array?
By exploring these advanced insights into array manipulation, you can enhance your programming skills and gain a deeper understanding of how arrays work in JavaScript. Remember to experiment with different scenarios and practice implementing these methods in your own projects for a more comprehensive grasp of the topic.
Additional Resources for Array Manipulation
-
MDN Web Docs: Array - A comprehensive guide on JavaScript arrays, including methods like
push
,pop
,shift
, andunshift
. -
JavaScript Array Methods - An overview of various array methods in JavaScript with examples.
-
Codecademy: Arrays - Interactive lessons on manipulating arrays in JavaScript.
-
FreeCodeCamp: JavaScript Array Methods - Learn about common array methods used in JavaScript.
Explore these resources to deepen your understanding of array manipulation and enhance your coding skills. Happy coding!
Practice
Task: Create an array of numbers and use push() to add an element to the end.
Task: Use pop() to remove the last element, shift() to remove the first, and unshift() to add a new element to the beginning.
Task: Log the array after each operation.