Creating and using Objects in JavaScript
JavaScript is an object-oriented programming language, where objects act as blueprints to group properties and methods for efficient code organization. Objects in JavaScript are essential for organizing data and functionalities in a structured manner.
Lets Go!
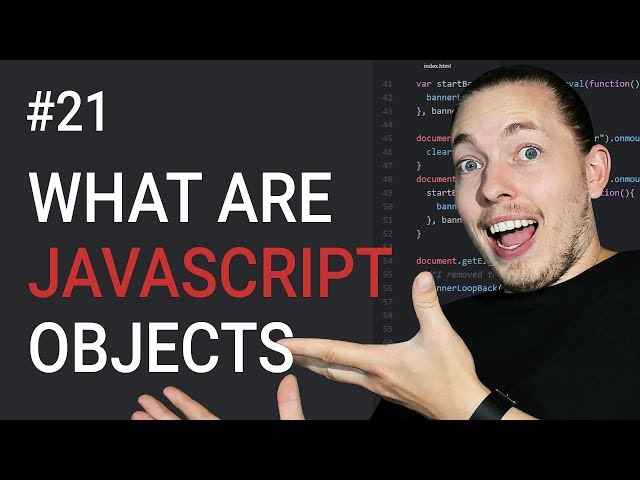
Creating and using Objects in JavaScript
Lesson 8
Learn how to create objects in JavaScript, assign properties and methods, and use them in your program.
Get Started 🍁Introduction to Objects in JavaScript
Welcome to "Introduction to Objects in JavaScript," where you'll embark on an exciting journey to explore the fundamental concept of objects in JavaScript. In this course, we will delve into the world of object-oriented programming within JavaScript, uncovering how objects serve as blueprints for grouping properties and methods to organize code efficiently.
Have you ever wondered about the underlying structure that powers JavaScript and how it handles data and functionality? Objects play a crucial role in this process, allowing programmers to encapsulate related information and behaviors within a single entity.
In this course, we will demystify the concept of objects by examining how they streamline code organization and enhance readability. You'll discover how built-in objects in JavaScript, such as strings and arrays, contain properties and methods that simplify data manipulation and conversion.
Through hands-on examples and interactive exercises, you will learn how to create your own objects, define properties, and implement custom methods to add dynamic functionality to your code. By the end of this course, you'll gain a deeper understanding of how objects are the building blocks of JavaScript applications and unlock the potential to efficiently manage and manipulate data structures.
So, are you ready to unlock the power of objects in JavaScript and elevate your programming skills to the next level? Join us on this learning journey and let's dive into the fascinating world of object-oriented programming!
Main Concepts of JavaScript Objects
-
Object-Oriented Programming: JavaScript is an object-oriented programming language, where objects serve as blueprints for grouping similar properties and methods together to organize code efficiently.
-
Built-In Objects: JavaScript includes built-in objects with pre-defined properties and methods, such as the
string
global object with properties likelength
and methods liketoString
. -
Creating Custom Objects: Users can create their own objects in JavaScript by defining a variable as an object and assigning values to its properties. For example,
let person = new Object(); person.name = "Daniel";
. -
Properties and Methods: Properties and methods inside objects are similar to variables and functions in the global scope. Properties store data, while methods perform actions and can be accessed using dot notation (
object.property
). -
Creating Methods: Methods can be added to objects by defining functions as properties. For instance,
person.updateAge = function() { return ++person.age; };
. -
Shorthand Object Creation: Objects can be created more concisely using shorthand notation within curly brackets. For example,
let person = { name: "Daniel", age: 27, updateAge: function() { return ++person.age; } };
. -
Object Constructors: Object constructors allow for creating multiple instances of an object based on a blueprint object. This helps in efficiently organizing and managing multiple instances of similar data by defining a template for object creation.
Practical Applications of Objects in JavaScript
Objects in JavaScript serve as blueprints for grouping similar properties and methods together to organize code more efficiently. Let's dive into creating and utilizing objects in JavaScript through a step-by-step guide.
Step 1: Creating an Object
First, create a variable to store the object using the new Object
syntax. Use the new
keyword followed by the type of object (in this case, a regular object).
let person = new Object;
Step 2: Assigning Values to the Object
Next, assign values to the object's properties. Use dot notation to set values for each property.
person.name = "Daniel"; person.eyeColor = "blue"; person.age = 27;
Step 3: Adding a Method to the Object
You can also add methods to an object. Define a method within the object using an anonymous function.
person.updateAge = function() { return ++person.age; };
Step 4: Accessing Object Properties and Methods
To access the properties and methods of the object, use dot notation. Console log the desired information to see the data.
console.log(person.name); console.log(person.updateAge());
Step 5: Creating Object Using Shorthand Syntax
Alternatively, you can create an object using shorthand syntax by directly assigning values within curly brackets.
let person = { name: "Daniel", eyeColor: "blue", age: 27, updateAge: function() { return ++person.age; } };
Step 6: Testing the Object
After creating the object, console log the object to see all the assigned properties and methods.
console.log(person);
Step 7: Further Exploration
In the next episode, we will delve into object constructors, which allow for creating blueprints to generate multiple object instances efficiently. Stay tuned for advanced object creation techniques in JavaScript.
Now, try creating your own objects following these steps and explore the power of object-oriented programming in JavaScript! Happy coding! 🚀✨
Test your Knowledge
How do you create an object in JavaScript?
How do you create an object in JavaScript?
Advanced Insights into JavaScript Objects
In this section, we will delve deeper into the concept of objects in JavaScript and explore more advanced aspects of using objects in your code.
Understanding Objects in JavaScript
Objects in JavaScript can be seen as blueprints that group together similar properties and methods. While we may have unknowingly used objects in our code before, it's crucial to understand that JavaScript has built-in objects that contain properties and methods we commonly use.
Building Your Own Objects
By creating your own objects in JavaScript, you can organize data more effectively than using variables. Instead of cluttering your code with multiple variables, structuring data within an object offers a cleaner and more manageable approach.
Creating Objects
To create objects in JavaScript, you typically use the new
keyword followed by the object type. Assign values to your object's properties using dot notation. You can also define methods within objects to perform specific actions, enhancing the functionality of your object.
Shorthand Object Creation
You can create objects using a shorthand notation by directly listing properties and values within curly braces. This concise method streamlines object creation and makes your code more readable.
Exploring Object Constructors
In the next lesson, we will dive into object constructors, which allow you to create blueprint templates for generating multiple instances of objects. Object constructors provide a structured approach to creating objects and are essential for efficient code organization.
Curiosity Question:
How can object constructors enhance the scalability and organization of your JavaScript codebase?
By mastering the creation and utilization of objects in JavaScript, you will be able to design more structured and efficient code. Keep exploring and experimenting with objects in JavaScript to deepen your understanding and optimize your development practices.
Additional Resources for Object-Oriented Programming in JavaScript
- MDN Web Docs: Objects
- JavaScript.info: Objects
- FreeCodeCamp: Introduction to Object-Oriented JavaScript
- Learn JavaScript Objects in Just 5 Minutes
- Object-Oriented Programming Concepts in JavaScript
These resources offer in-depth explanations and examples to enhance your understanding of working with objects in JavaScript. Happy learning! 🚀
Practice
Task: Create an object named person with properties name, age, and hobbies. Add a method introduce() that logs a greeting using the name and age.
Task: Instantiate an object for a car with properties brand, model, and year. Log the object to the console.