Defining and Using Arrays
An introduction to arrays in JavaScript, covering how arrays work as data structures and how to interact with them in code.
Lets Go!
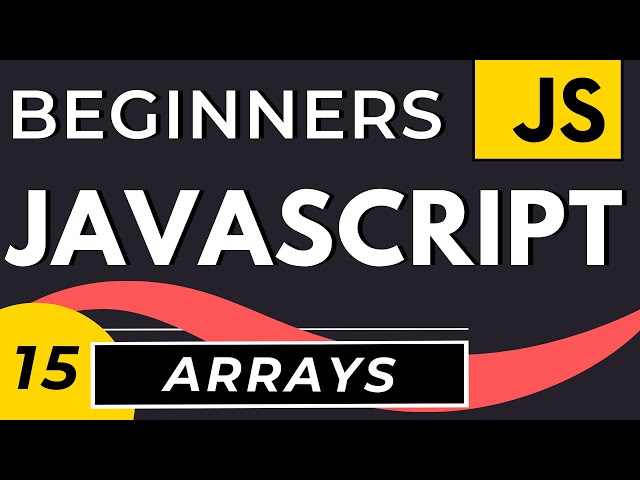
Defining and Using Arrays
Lesson 11
Understand how to create, access, and manipulate arrays in JavaScript.
Get Started 🍁Introduction to Data Structures: Arrays in JavaScript
Welcome to "Introduction to Data Structures: Arrays in JavaScript"!
In this course, we will embark on an exciting journey into the world of data structures, focusing specifically on arrays in JavaScript. Just like shelves where we store items, arrays are essential data structures that allow us to organize and manage different types of data efficiently.
We will start by exploring the basics of arrays, how to define and manipulate them, and uncover their fundamental properties. During this interactive course, you will learn how to work with arrays, add and remove elements, access specific items, and even delve into the world of nested arrays.
Are you ready to unlock the power of arrays and elevate your coding skills? Let's dive in and discover the fascinating world of data structures in JavaScript together!
Curiosity Question:
- Have you ever wondered how arrays can be used as powerful tools for storing and manipulating data in JavaScript? Join us to explore the endless possibilities!
Get ready to master arrays like a pro and take your coding skills to new heights. Let's begin our array adventure! 🚀
Main Concepts of Data Structures
-
Arrays in JavaScript Arrays in JavaScript serve as a data structure, acting as shelves to store various elements. They are defined using brackets ([]), indicating an empty array or initializing it with specific elements. Unlike variables defined with
const
, the elements within an array are mutable and can be reassigned. -
Adding and Accessing Elements Elements can be added to an array using bracket notation to specify the position in the array. Remember, array positions start at zero, just like indexing in strings. Accessing elements within an array involves referencing the specific position using brackets and the position number.
-
Array Methods (Push, Pop, Shift, Unshift)
push
: Add new elements to the end of the array, increasing its length.pop
: Remove the last element of the array and return that element.shift
: Remove the first element of the array and return that element.unshift
: Add new elements to the beginning of the array, shifting existing elements.
-
Array Methods (Slice, Reverse, Join)
slice
: Extracts a section of the array and returns it as a new array.reverse
: Reverses the order of elements in the array.join
: Converts the array into a string, with an optional separator between elements.
-
Array Processing (Delete, Splice)
delete
: Removes a specific element from the array, leaving an empty slot (undefined) in its place.splice
: Allows for deletion and replacement of elements within the array, providing more control over modifications.
-
Concatenation and Spread Operator
concat
: Joins two arrays together, creating a new array with the combined elements.- Spread Operator (
...
): Expands an array into individual elements, enabling easy merging of multiple arrays without nesting.
-
Nested Arrays Nested arrays involve arrays within arrays, creating multiple dimensions. Accessing elements in multi-dimensional arrays requires navigating through each level of nesting, identifying the correct positions at each level.
By understanding these concepts and practicing array manipulation in JavaScript, you can effectively store and retrieve data structures in your programs.
Practical Applications of Arrays in JavaScript
Arrays in JavaScript serve as a fundamental data structure for storing and organizing data. They are versatile and can hold various types of data, making them essential in programming. Here is a step-by-step guide on how to work with arrays in JavaScript:
-
Creating an Array:
- Define an empty array using brackets:
const myArray = [];
- Add elements to the array using positions:
myArray[0] = "Dave";
- Different data types can coexist in the array: string, number, boolean, etc.
- Define an empty array using brackets:
-
Accessing Array Elements:
- Check the length of the array:
console.log(myArray.length);
- Access specific elements using their positions:
console.log(myArray[1]);
- Check the length of the array:
-
Adding and Removing Elements:
- Add an element to the end of the array:
myArray.push("School");
- Remove the last element:
myArray.pop();
- Remove the first element:
myArray.shift();
- Add an element to the end of the array:
-
Manipulating Array Elements:
- Use
splice
to delete or replace elements:myArray.splice(1, 1);
- Use
slice
to extract a portion of an array:myArray.slice(0, 2);
- Use
-
Working with Multi-dimensional Arrays:
- Create nested arrays for complex data structures
- Access elements in multi-dimensional arrays:
myArray[1][0][1];
-
Advanced Array Methods:
- Reverse an array:
myArray.reverse();
- Join array elements into a string:
myArray.join();
- Concatenate arrays using
concat
or the spread operator
- Reverse an array:
-
Practical Example:
- Create a multi-level array representing a sports store
- Access specific items within nested arrays by navigating through dimensions
Get Hands-On: Try creating your array, adding, removing, and manipulating elements. Experiment with multi-dimensional arrays to understand their power in organizing complex data structures. Happy coding! 💻🚀
Test your Knowledge
How do you access the third element in an array named myArray?
How do you access the third element in an array named myArray?
Advanced Insights into Arrays in JavaScript
Now that you have a solid understanding of arrays in JavaScript, let's delve into some advanced insights that will enhance your proficiency with this data structure.
Nested Arrays and Multi-Dimensional Arrays
Arrays can contain other arrays, creating nested arrays. This means you can have arrays within arrays, also known as multi-dimensional arrays. By nesting arrays, you can organize and structure your data hierarchically. Accessing values within multi-dimensional arrays involves referring to the specific dimensions and positions within each array.
Array Methods Beyond Basic Operations
Beyond basic array operations like adding, removing, and accessing elements, JavaScript provides powerful array methods to manipulate and work with arrays more effectively. These methods include slice
, reverse
, join
, split
, concat
, and leveraging the spread operator (...
) for array concatenation.
Best Practices for Array Management
When working with arrays, it's important to handle array modifications carefully. Avoid using the delete
operator for removing elements from arrays as it leaves behind undefined
values. Instead, prefer using the splice
method for precise element removal and replacement within arrays.
Performance Considerations and Nested Array Access
As arrays grow in size and complexity, performance considerations become crucial. Pay attention to efficient ways of accessing elements within nested arrays to prevent unnecessary computation overhead. Optimize your code by minimizing iterations and accessing elements directly using the correct array indices.
Continuous Learning and Practice
To master arrays in JavaScript, consistent practice and exploration of advanced array concepts are essential. Challenge yourself with complex array structures, experiment with different array methods, and aim to optimize your code for better performance. Embrace the learning journey and strive for continuous improvement.
Curiosity Question
How can you implement a recursive function to traverse through a multi-dimensional array and perform specific operations on each element recursively? Give it a try and see how recursion can enhance your array manipulation skills. Happy coding!
Additional Resources for Arrays in JavaScript
Here are some additional resources to further your understanding of arrays in JavaScript:
- MDN Web Docs: Arrays - A comprehensive guide to arrays in JavaScript provided by Mozilla Developer Network.
- JavaScript Arrays Explained - An in-depth tutorial on arrays in JavaScript from W3Schools.
- JavaScript Array Methods - Explore various methods available for manipulating arrays in JavaScript.
- JavaScript Data Structures and Algorithms - A Udemy course on mastering data structures and algorithms in JavaScript.
Dive into these resources to enhance your knowledge and skills in working with arrays in JavaScript. Happy learning! 🚀
Practice
Task: Create an array of your favorite fruits and log the first and last elements.
Task: Add a new fruit to the array and remove one fruit.
Task: Log the updated array to the console.