Functions in JavaScript
This content piece provides a comprehensive overview of function expressions, function declarations, and arrow functions in JavaScript. It explains the differences, pros, and cons of each to help users make informed decisions on when and why to use them.
Lets Go!
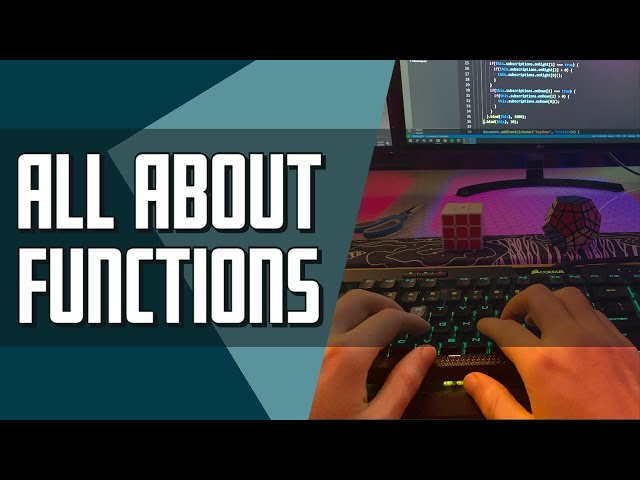
Functions in JavaScript
Lesson 7
Understand how to define functions in different ways (declaration, expression, and arrow functions) and how to call them with arguments.
Get Started 🍁Introduction to Function Expressions, Declarations, and Arrow Functions
Welcome to "Introduction to Function Expressions, Declarations, and Arrow Functions"! In this course, we will explore the fundamental concepts of function expressions, function declarations, and arrow functions in JavaScript.
Have you ever wondered about the differences between function expressions, function declarations, and arrow functions? Do you want to understand the pros and cons of each and know when to use one over the other? If so, this course is perfect for you!
We will start by delving into function declarations, the classic way to define functions in JavaScript, followed by function expressions, which provide a different syntax and functionality. Additionally, we will explore immediately invoked function expressions (IIFEs) and discover how they can improve encapsulation and create function factories.
Furthermore, we will discuss arrow functions, which offer a more concise way to write functions compared to traditional function expressions. You will learn about the differences between arrow functions and other function types and when to use them in your code.
Throughout this course, you will gain a better understanding of function definitions and how to leverage different types of functions in your JavaScript code. By the end of this course, you will have the knowledge and skills to effectively use function expressions, declarations, and arrow functions in your projects.
Are you ready to level up your JavaScript skills and master function types? Let's dive in and explore the world of function expressions, declarations, and arrow functions together!
Main Concepts of Function Expressions, Declarations, and Arrow Functions
-
Function Declaration:
- The classic way to define a function in JavaScript using the
function
keyword followed by the name of the function. - Function declarations are hoisted along with their definitions, meaning they can be used before they are actually written in the code.
- Best utilized when the function needs to be available globally.
- The classic way to define a function in JavaScript using the
-
Function Expression:
- Different from function declarations in that they are written by assigning a function to a variable using
const
,let
, orvar
. - Function expressions are more concise and can be passed directly as arguments to other functions.
- They are hoisted without their definitions, causing a reference error if used before they are defined.
- Different from function declarations in that they are written by assigning a function to a variable using
-
Immediately Invoked Function Expressions (IIFE):
- Allows defining a function expression and invoking it immediately by wrapping it in parentheses and calling it with another set of parentheses.
- Useful for encapsulation, creating private scopes, and avoiding naming conflicts in large codebases.
- Can be used as function factories to generate values that require complex computations in a concise manner.
-
Arrow Functions:
- Super concise function expressions introduced in ES6 in 2015.
- Does not bind its own
this
keyword, making it challenging to access specific values within the function. - More concise syntax compared to regular function expressions, especially for single-line functions.
- Arrow functions are great for quick, one-off tasks and can be passed directly to other functions.
These main concepts highlight the differences between function expressions, declarations, and arrow functions, emphasizing their unique features and best use cases in JavaScript programming.
Practical Applications of Function Declarations, Function Expressions, and Arrow Functions
Step-by-Step Guide:
-
Function Declarations:
- Function declarations are the classic way to define a function in JavaScript.
- To define a function using a function declaration:
function squareNum(num) { return num * num; }
-
Function Expressions:
- Function expressions assign a function to a variable on the fly.
- To transform a function declaration into a function expression:
const squareNum = function(num) { return num * num; };
-
IIFE (Immediately Invoked Function Expressions):
- IIFEs allow you to define and immediately invoke a function.
- Example using an IIFE with squareNum function:
const twoSquared = (function(num) { return num * num; })(2); console.log(twoSquared); // Output will be 4
-
Arrow Functions:
- Arrow functions are concise function expressions introduced in ES6.
- To convert a function expression to an arrow function:
const squareNum = (num) => num * num;
Try It Out:
- Create a simple function using a function declaration.
- Transform the function declaration into a function expression.
- Write an IIFE for a function of your choice and immediately invoke it.
- Experiment with converting a function expression into an arrow function and observe the differences in syntax.
Get hands-on experience with these concepts to solidify your understanding! Let us know in the comments if you have any questions or need further clarification. Happy coding!
Test your Knowledge
Which syntax is correct for declaring a function in JavaScript?
Which syntax is correct for declaring a function in JavaScript?
Advanced Insights into Function Declarations, Function Expressions, and Arrow Functions
In this section, we will delve into advanced aspects of function declarations, function expressions, and arrow functions to provide you with a deeper understanding of these concepts in JavaScript.
Hoisting and Scope
Function declarations are hoisted along with their definitions, meaning they can be used before they are declared in the code. On the other hand, function expressions are not hoisted with their definitions, similar to standard variable bindings. This distinction is crucial when considering the order in which functions can be utilized within your codebase.
Curiosity Question: How does hoisting impact the execution order of functions in JavaScript?
Immediately Invoked Function Expressions (IIFE)
IIFE stands for Immediately Invoked Function Expression, which allows you to define a function and immediately invoke it. This pattern aids in encapsulating code modules and preventing naming conflicts in complex JavaScript applications. By leveraging IIFE, you can create self-contained modules with private scopes, enhancing code maintainability and reusability.
Curiosity Question: How can IIFE enhance code organization and prevent naming conflicts in large-scale JavaScript projects?
Arrow Functions and Conciseness
Arrow functions offer a more concise syntax for writing functions in JavaScript compared to traditional function expressions. They were introduced in ES6 and provide a cleaner and more compact way to define functions. Unlike regular function expressions, arrow functions do not bind their own this
, making them suitable for certain use cases where context binding is not needed.
Curiosity Question: What are the key differences between arrow functions and regular function expressions in JavaScript?
By gaining a deeper understanding of function declarations, function expressions, and arrow functions, you'll be able to leverage these concepts effectively in your JavaScript projects, optimizing code readability and maintainability. Explore these concepts further to unlock their full potential in your coding journey.
Additional Resources for Function Declarations, Function Expressions and Arrow Functions
- MDN Web Docs: Functions: A comprehensive guide on functions in JavaScript, including function declarations, function expressions, and arrow functions.
- JavaScript.info: Arrow functions, the basics: A detailed explanation of arrow functions, their syntax, and how they differ from regular functions.
- ES6 Features: Arrow Functions: An in-depth look at arrow functions in ES6 and why they are considered a clean and concise way to write JavaScript code.
Explore these resources to gain a deeper understanding of function declarations, function expressions, and arrow functions in JavaScript. Happy coding! 🚀
Practice
Task: Write a function using function declaration to calculate the square of a number.
Task: Create an anonymous function assigned to a variable that calculates the sum of two numbers.
Task: Write an arrow function that takes two parameters and returns their product.