JavaScript Syntax and Basic Structure
JavaScript syntax and structure are crucial for beginners to grasp in order to write functional code. This video explains the basics and importance of adhering to JavaScript's case sensitivity, statement structure, use of semicolons, handling of whitespace, commenting, and the order of code execution.
Lets Go!
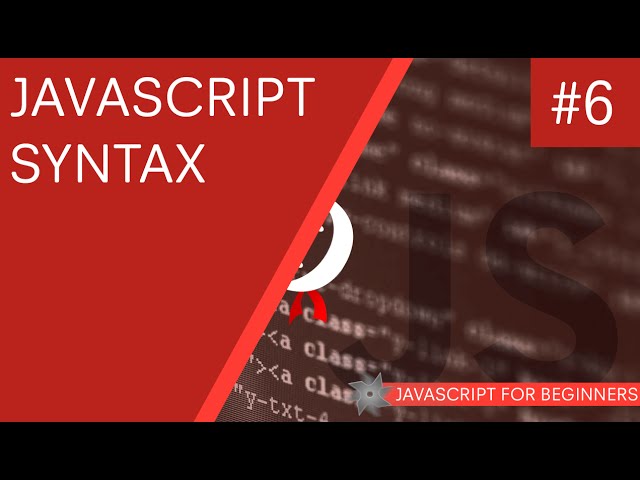
JavaScript Syntax and Basic Structure
Lesson 4
Understand the basic syntax, including variables, functions, and loops, and the overall structure of JavaScript programs.
Get Started 🍁Introduction to JavaScript Syntax and Structure
Welcome to "Introduction to JavaScript Syntax and Structure"! Have you ever wondered how JavaScript, a powerful programming language, can be used to create dynamic and interactive web pages?
In this course, we will dive into the fundamental aspects of JavaScript syntax and structure that lay the foundation for writing efficient and effective code. Whether you are a beginner looking to learn the basics or someone seeking to enhance their existing skills, this course is designed to provide you with a comprehensive understanding of key concepts.
Topics covered in this course include:
- Case sensitivity: Understand the importance of case sensitivity in JavaScript and how it can impact the functionality of your code.
- Statements and semicolons: Learn how to structure statements in JavaScript and the significance of ending each statement with a semicolon.
- Whitespace: Explore how JavaScript handles whitespace and line breaks, and how it impacts the readability of your code.
- Comments: Discover how to incorporate comments in your code to enhance its clarity and maintainability.
- Execution order: Gain insights into how JavaScript executes code from top to bottom and why the order of statements is crucial for achieving desired outcomes.
By the end of this course, you will have a solid grasp of JavaScript syntax and structure, equipping you with the knowledge and skills to write clean and functional code.
Are you ready to embark on this exciting journey into the world of JavaScript? Let's get started!
Main Concepts of JavaScript Syntax and Structure
-
Case Sensitivity: JavaScript is case sensitive, meaning that the use of uppercase and lowercase letters in functions, variables, and statements must be consistent. For example,
alert
andAlert
would refer to two different functions in JavaScript due to the difference in letter casing. -
Statements and Semicolons: In JavaScript, most actions are considered statements, such as displaying an alert box, changing the background color of a webpage, or positioning an image. Each statement in JavaScript should end with a semicolon, which helps to separate different actions and maintain clarity in the code.
-
Whitespace: Whitespace, including spaces and line breaks, is not significant in JavaScript and can be used to format the code for readability. It is common practice to group related statements together with minimal whitespace or line breaks, while separating distinct portions of code with additional whitespace or comments.
-
Comments: Comments in JavaScript can be used to provide explanations or document code. Single-line comments start with
//
, while multiline comments are enclosed between/*
and*/
. Comments help developers understand the purpose of different sections of code and can be especially useful when working with longer scripts. -
Execution Order: JavaScript code is executed from top to bottom, following the order of statements in the script. Ensuring the correct sequence of actions is crucial to achieving desired outcomes in JavaScript programs. Changing the order of statements can significantly impact the behavior of the code and the result it produces.
By grasping these fundamental concepts of JavaScript syntax and structure, beginners can enhance their understanding of how to write effective and organized code in the language. Each principle plays a crucial role in shaping the way JavaScript functions and how developers interact with and manipulate elements within a web page or application.
Practical Applications of JavaScript
Now that we have gone over the basic syntax and structure of JavaScript, let's dive into some practical applications. Follow these steps to start implementing JavaScript in your projects:
-
Case Sensitivity:
- Remember that JavaScript is case sensitive. Always use the correct capitalization when calling built-in functions or naming your own functions and variables. For example,
myFunction
is not the same asMyFunction
.
- Remember that JavaScript is case sensitive. Always use the correct capitalization when calling built-in functions or naming your own functions and variables. For example,
-
Statements and Semicolons:
- In JavaScript, most actions are considered statements. Each statement should end with a semicolon to separate them properly. Ensure you add a semicolon after each statement to avoid confusion or errors.
-
Whitespace and Line Breaks:
- While whitespace and line breaks are not necessary for JavaScript functionality, it is essential for readability. Organize your code by grouping related statements together and adding line breaks for clarity.
-
Comments:
- Use comments to annotate your code and provide context for yourself and other developers. Use
//
for single-line comments and/* */
for multi-line comments. This will help you remember the purpose of each section of your code.
- Use comments to annotate your code and provide context for yourself and other developers. Use
-
Order of Operations:
- JavaScript executes code from top to bottom. Ensure that you place your statements in the correct order to achieve the desired outcome. Changing the order of statements can significantly impact the functionality of your code.
Take the following steps to practice applying these principles:
- Create a new JavaScript file in your text editor.
- Write a simple function with proper capitalization and semicolons.
- Add comments to explain each step of the function.
- Arrange multiple statements in the correct order to achieve a specific outcome.
- Save your file and test your JavaScript code in a browser console.
By following these steps, you will gain a better understanding of how to structure and write JavaScript code effectively. Don't hesitate to experiment with different examples and seek support in the developer community if needed. Ready to put your JavaScript skills to the test? Give it a try and see the results in action! 🚀
Test your Knowledge
What is the correct way to declare a variable in JavaScript?
What is the correct way to declare a variable in JavaScript?
Advanced Insights into JavaScript
Now that we have covered the basic syntax and structure of JavaScript in previous lessons, let's delve into some advanced insights to enhance your understanding of this powerful language.
Case Sensitivity in JavaScript
JavaScript is case-sensitive, meaning that the capitalization of letters matters. For example, calling a function with an uppercase letter when it should be lowercase will result in an error. Always remember to use the correct casing for functions and variables to avoid issues in your code.
Statements and Semicolons
In JavaScript, most actions are considered statements, such as displaying an alert box or changing the background color of a webpage. Each statement should end with a semicolon to indicate its completion. While omitting semicolons may not always cause immediate errors, it is best practice to include them for clarity and to prevent potential issues when adding more statements.
White Space and Comments
Unlike case sensitivity, JavaScript is not sensitive to white space or line breaks. You can format your code with spaces and line breaks as you see fit for readability. Utilize comments to annotate your code, providing context for yourself and other developers. Single-line comments start with two forward slashes "//", while multi-line comments are enclosed between "/" and "/".
Code Execution Order
JavaScript code is executed from top to bottom, following the order in which statements are written. Pay attention to the sequence of your statements, especially when tasks need to be performed in a specific order. Changing the order of statements can significantly impact the functionality of your code.?
By understanding these advanced insights into JavaScript, you can create more efficient and organized code that functions as intended. Experiment with different formatting styles and comment strategies to find what works best for you!
What creative ways can you use comments to enhance collaboration with other developers in a JavaScript project?
Additional Resources for JavaScript Basics
Explore these resources to deepen your understanding of JavaScript syntax and structure. Happy coding! 🚀
Practice
- Write a script that:
- Declares a variable to store your name.
- Writes a function to print 'Hello, [Your Name]' to the console.
- Uses a loop to print numbers from 1 to 5.