Multidimensional Arrays and “For In” Loops
A multi-dimensional array in JavaScript is an array of arrays used to store data in a table-like structure. This tutorial explores how to set up and access values in multi-dimensional arrays, as well as discusses the use of objects instead of arrays for certain situations.
Lets Go!
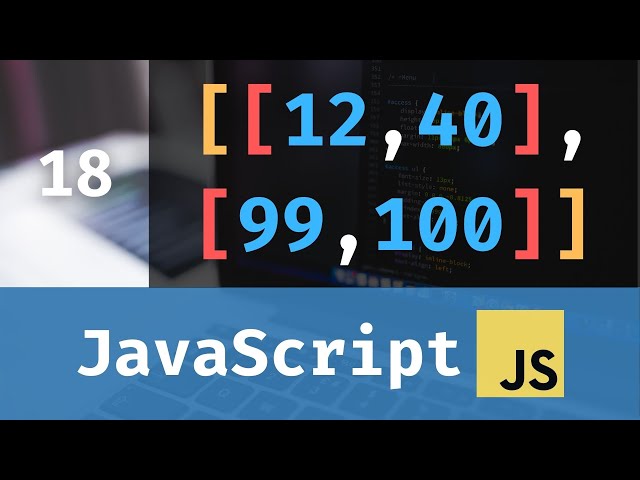
Multidimensional Arrays and “For In” Loops
Lesson 14
Learn how to create and traverse multidimensional arrays, and understand how to use for...in loops
Get Started 🍁Introduction to Multi-Dimensional Arrays in JavaScript
Welcome to "Introduction to Multi-Dimensional Arrays in JavaScript"! In this course, we will delve into the world of multi-dimensional arrays in JavaScript. Even though JavaScript does not natively support true multi-dimensional arrays, we can approximate them using arrays of arrays. Through this tutorial, we will explore how to set up multi-dimensional arrays and how to retrieve values efficiently.
What Will You Learn?
- Setting up an array of arrays to store data, much like a table with rows and columns.
- Accessing individual values within multi-dimensional arrays using index notation.
- Exploring the use of objects with arrays and the advantages it offers over arrays of arrays.
Curiosity Question
Ever wondered how you can efficiently store and access data represented in a table-like format in JavaScript?
Join us in this course to unravel the secrets of multi-dimensional arrays and discover the best practices for managing complex data structures in JavaScript. Let's embark on this exciting journey together!
Main Concepts of Multi-Dimensional Arrays in JavaScript
-
Definition of Multi-Dimensional Arrays: Javascript does not support true multi-dimensional arrays, but they can be approximated with an array of arrays. In this tutorial, we will explore multi-dimensional arrays in JavaScript and see how to use objects as well.
-
Setting Up Multi-Dimensional Arrays: To set up a multi-dimensional array, you start by declaring a variable as an array, and then you can include additional arrays within it. These additional arrays represent different rows and columns of data.
-
Accessing Values in Multi-Dimensional Arrays: To access individual values in a multi-dimensional array, you use nested square brackets. The outermost bracket accesses the main array, while subsequent brackets access the inner arrays. For example,
table[0][2]
would access the value at row 1, column 3. -
Looping Through Multi-Dimensional Arrays: To iterate through all the values in a multi-dimensional array, you can use nested
for
loops. The outer loop iterates through the main array, while the inner loop iterates through the inner arrays to access all values. -
Using Objects vs. Arrays of Arrays: Sometimes it makes more sense to use objects with arrays rather than arrays of arrays, especially when data is associated with specific types or categories. Objects can provide a more logical structure for organizing and accessing data.
-
Accessing Values in Object of Arrays: When using objects with arrays, you can access individual values by specifying the property key (like 'mammals' or 'fish') followed by the index position within the array associated with that property.
-
Looping Through Object of Arrays: When dealing with an object of arrays, you can use a
for-in
loop to iterate through the properties of the object. Within this loop, you can then use afor
loop to cycle through the arrays associated with each property to access and display all values.
By understanding these main concepts, you can effectively work with multi-dimensional arrays in JavaScript and choose the most suitable data structure for your programming needs.
Practical Applications of Multi-Dimensional Arrays in JavaScript
A multi-dimensional array is a great way to store data like a table with rows and columns. Let's break down how to set up and access values in a multi-dimensional array in JavaScript.
Setting up an Array of Arrays
- Declare a variable for your multi-dimensional array. For example,
table = []
. - Populate the array with additional arrays representing rows and columns. This creates an array of arrays structure.
- You can speed up this process by copying and pasting the array contents, making sure each array represents a row or column.
Accessing Individual Values
- To access specific values in the multi-dimensional array, use console log statements.
- Use index reference to fetch values from inner arrays. For example:
table[0][2]
would retrieve the value in the first row and third column.
Cycling Through and Displaying All Values
- Use nested for loops to cycle through and display all values in the multi-dimensional array.
- The outer loop iterates over the main array, while the inner loop navigates through each inner array.
- Use curly braces to encapsulate the console log statement with indices
table[I][J]
.
- Use curly braces to encapsulate the console log statement with indices
Use of Objects Instead of Arrays
- Sometimes it's more logical to use objects with arrays, especially when data is associated with specific types or categories.
- Accessing values in an object of arrays is similar to arrays but uses keys instead of indices.
Cycling Through and Displaying Values in Object of Arrays
- Utilize a for...in loop to iterate through the object properties.
- Within the loop, use a for loop to navigate through each array associated with the object key.
- Display values using
table2[item][K]
inside the console log statement.
- Display values using
By following these steps, you can efficiently work with multi-dimensional arrays in JavaScript and explore the possibilities of using objects for structured data. Feel free to experiment with different scenarios and let us know your thoughts!
Test your Knowledge
How do you access the value 4 in the following array?
let matrix = [[1, 2], [3, 4]];
How do you access the value 4 in the following array? let matrix = [[1, 2], [3, 4]];
Advanced Insights into Multi-Dimensional Arrays in JavaScript
When working with multi-dimensional arrays in JavaScript, it's important to understand how to set them up effectively and efficiently. Let's delve deeper into some advanced aspects and insights on this topic.
Set up Multi-Dimensional Arrays
To create a multi-dimensional array, you can use an array of arrays approach. This involves declaring an array and populating it with additional arrays to represent your data structure. ensure each dimension is accounted for in the setup to accurately store and access your data.
Tip:
- Use a structured approach to organize your arrays within arrays for better data management.
Curiosity Question:
- How does the order of dimensions impact the storage and retrieval of data in multi-dimensional arrays?
Accessing Values in Multi-Dimensional Arrays
Once you have set up your multi-dimensional array, accessing individual values becomes crucial. By utilizing nested loops, you can efficiently iterate through the dimensions to retrieve specific data points.
Recommendation:
- Implement nested loops to navigate through the various dimensions of your multi-dimensional array for accurate data retrieval.
Curiosity Question:
- How can you optimize the process of accessing values in multi-dimensional arrays for improved performance?
Objects vs. Arrays of Arrays
In some scenarios, using objects with arrays might be a more logical choice than using arrays of arrays. Objects allow for a more structured organization of data based on key-value pairs, enhancing readability and clarity in your code.
Expert Advice:
- Consider using objects when your data has distinct categories or associated properties for better data representation.
Curiosity Question:
- What are the key advantages of utilizing objects over arrays of arrays in certain data structuring scenarios?
Cycling Through Values in Objects
When utilizing objects instead of arrays of arrays, iterating through all values stored in the object requires a different approach. By leveraging for...in
loops along with nested loops, you can effectively display and access all values within the object.
Tip:
- Combine
for...in
loops with nested loops to cycle through and display values in objects efficiently.
Curiosity Question:
- How does the use of
for...in
loops differ from traditional loops when working with objects in JavaScript?
By exploring these advanced insights into multi-dimensional arrays in JavaScript and understanding the nuances between arrays and objects, you can enhance your proficiency in data structuring and manipulation. Practicing these concepts will solidify your understanding and improve your development skills. Experiment with different data structures to gain a deeper understanding of handling complex data in JavaScript. Happy coding!
Additional Resources for Multi-dimensional Arrays in JavaScript
Here are some additional resources to enhance your understanding of multi-dimensional arrays in JavaScript:
-
MDN Web Docs: Arrays - Explore in-depth explanations and examples of working with arrays in JavaScript.
-
JavaScript.info: Array Methods - Learn about various array methods that can help you manipulate multi-dimensional arrays effectively.
-
W3Schools: JavaScript Arrays - Practice and experiment with arrays using interactive examples and exercises.
-
FreeCodeCamp: JavaScript Data Structures - Dive into more advanced data structures in JavaScript beyond arrays.
These resources will provide you with comprehensive knowledge and practical experience to master multi-dimensional arrays in JavaScript. Happy learning!
Practice
Task: Create a 2D array representing a matrix (e.g., [[1, 2], [3, 4]]). Log each row and each element within the rows.
Task: Use a for...in loop to log the index and corresponding value of a simple array.