Setting Up A JavaScript Development Environment
Setting up a development environment for learning JavaScript involves selecting a text editor, installing Node.js for JavaScript runtime, working with a browser, and utilizing browser extensions.
Lets Go!
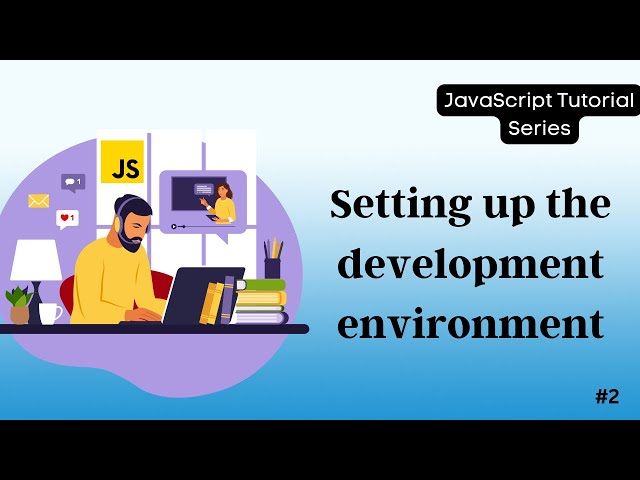
Setting Up A JavaScript Development Environment
Lesson 2
Learn how to set up a comprehensive development environment for JavaScript, covering text editor choice, Node.js installation, browser setup, and essential browser extensions.
Get Started 🍁Introduction to JavaScript Development
Welcome to our course, "Introduction to JavaScript Development"! In this course, we will provide you with all the necessary guidance to set up a robust development environment for learning JavaScript. From choosing a text editor and installing Node.js to creating a development server and working with a browser, we've got you covered.
Have you ever wondered how websites and web applications are built? JavaScript is a fundamental programming language that powers dynamic web content and interactivity. By the end of this course, you will have a complete development environment ready to dive into the world of JavaScript and start creating amazing projects.
We will start by exploring the importance of selecting a text editor or integrated development environment (IDE) for writing and editing your code efficiently. Then, we will delve into installing Node.js, a crucial JavaScript runtime for building web applications and APIs. Additionally, we will introduce you to npm (Node Package Manager) for managing third-party libraries and frameworks.
Next, we will guide you through setting up a development server using Node.js and Express.js, a popular framework for creating web applications. You will learn how to create a basic server and serve HTML files to test your JavaScript applications effectively.
We will also talk about utilizing modern web browsers, particularly Google Chrome, and leveraging its developer tools to inspect and debug your code. Furthermore, you will discover the benefits of browser extensions such as LiveReload, Vue.js DevTools, and React Developer Tools for enhancing your development experience.
Are you ready to embark on an exciting journey into the world of JavaScript development? Let's set up your development environment and start building innovative projects together!
Main Concepts of Setting Up a Development Environment for Learning JavaScript:
-
Choosing a Text Editor or IDE:
- A text editor is a lightweight tool for writing and editing text files, while an IDE is a more powerful tool with features like code highlighting and auto-completion.
- Visual Studio Code (VS Code) is recommended, and can be downloaded for free from code.visualstudio.com.
-
Installing Node.js:
- Node.js is a JavaScript runtime outside of a browser, essential for web applications and APIs.
- Download Node.js from nodejs.org for your operating system, and follow the installation instructions.
-
Installing a Package Manager (npm):
- npm (Node Package Manager) helps manage and install third-party libraries for JavaScript projects.
- Use terminal/command prompt to check npm installation with "npm -v" and install packages with "npm install <package-name>".
-
Setting Up a Development Server with Express.js:
- Express.js is a Node.js framework for creating web applications and APIs.
- Create and navigate to a project folder, initialize npm package, install Express.js, create 'index.js' file, and run the server with 'node index.js'.
-
Working with a Browser and Chrome Developer Tools:
- Google Chrome is recommended for its developer tools to inspect and debug HTML, CSS, and JavaScript.
- Right-click on a web page and select 'Inspect' to access these tools for debugging and inspecting elements.
-
Browser Extensions for JavaScript Development:
- Browser extensions like LiveReload, Vue.js DevTools, and React Developer Tools add extra functionality for development.
- Find and install these extensions from the Chrome Web Store, which provide features like auto-refresh, advanced debugging for Vue.js and React.js applications.
Practical Applications of Setting Up a Development Environment for Learning JavaScript
Steps:
-
Choose a Text Editor or IDE
- Decide on a text editor or integrated development environment (IDE) to write and edit your JavaScript code.
- In this tutorial, we are using Visual Studio Code (VS Code).
- Visit VS Code website to download for your operating system.
- Follow installation instructions to set up the software.
-
Install Node.js
- Node.js allows you to run JavaScript code outside of a browser, making it essential for web development.
- Visit Node.js website to download for your operating system.
- Follow installation instructions for Node.js.
- Confirm installation by typing "node -v" in your terminal.
-
Set Up a Package Manager
- Use npm (Node Package Manager) to manage and install third-party libraries for your JavaScript projects.
- Confirm npm installation by typing "npm -v" in your terminal.
- Install JavaScript libraries and frameworks using "npm install <package-name>".
-
Set Up a Development Server
- Use Node.js and Express.js framework to create a local server for running JavaScript applications.
- Create a new project folder and open in your text editor.
- In your terminal, navigate to the project folder and type "npm init" to create a new npm package.
- Install Express.js framework by typing "npm install express" in your terminal.
- Create a new file called "index.js" in your project folder.
- Import Express.js, create app instance, and define a route for serving HTML.
- Run the server using "node index.js" in your terminal.
-
Work with a Browser
- Test your JavaScript applications in a browser, starting with Google Chrome.
- Use Chrome's developer tools to inspect HTML, CSS, and JavaScript on a web page.
- Right-click on a page and select "Inspect" to access developer tools.
- Familiarize yourself with the tools for debugging and inspection.
-
Browser Extensions
- Install browser extensions for additional functionality in your browser.
- Search for and install LiveReload, Vue.js DevTools, and React Developer Tools from the Chrome Web Store.
- These extensions provide features like automatic page refresh, advanced debugging for Vue.js and React.js applications.
Try it out!
Go ahead and follow the steps outlined above to set up your development environment for learning JavaScript. Experiment with installing the required software, setting up a local server, and testing your code in a browser. Happy coding!
Test your Knowledge
Which tool is used to run JavaScript outside a browser?
Which tool is used to run JavaScript outside a browser?
Advanced Insights into JavaScript Development
Building on the basics covered in the tutorial, let's delve into some advanced insights into setting up a development environment for learning JavaScript.
Using an IDE for Advanced Development
While a text editor is great for getting started, consider transitioning to an Integrated Development Environment (IDE) for more advanced features like code highlighting, auto-completion, and debugging. IDEs like Visual Studio Code offer powerful tools to streamline your development process.
Expert Tip: Utilize Visual Studio Code's extensions marketplace to enhance your coding experience further. Search for and install extensions that cater to your specific needs, such as linters, code formatters, and debugging tools.
Curiosity Question: How can you customize your IDE to optimize your workflow and improve productivity?
Harnessing the Power of Node.js
Beyond just running JavaScript code outside the browser, Node.js enables the creation of robust web applications and APIs. Familiarize yourself with advanced concepts like asynchronous programming, package management, and server-side development using Node.js.
Expert Tip: Explore Node.js frameworks like Express.js, Koa, or Nest.js to build scalable and efficient web services. These frameworks provide additional functionalities and tools to elevate your backend development projects.
Curiosity Question: How does Node.js facilitate event-driven, non-blocking I/O operations, and what advantages does this offer for web application development?
Leveraging Browser Extensions for Enhanced Development
Browser extensions like LiveReload, Vue.js DevTools, and React Developer Tools can significantly boost your JavaScript development workflow. These tools offer advanced debugging, inspection, and real-time code updates for popular JavaScript frameworks.
Expert Tip: Experiment with other browser extensions tailored to your specific needs, such as code validators, snippet managers, or performance analyzers. Customizing your browser environment can enhance your coding efficiency and effectiveness.
By exploring these advanced insights and implementing best practices in your JavaScript development journey, you can elevate your skills, build impressive projects, and stay ahead in the dynamic world of web development. Keep exploring, learning, and experimenting to unlock the full potential of JavaScript!
Additional Resources for JavaScript Development
References:
- MDN Web Docs - JavaScript - Comprehensive documentation for JavaScript.
- JavaScript.info - A modern JavaScript tutorial.
Articles:
- 10 JavaScript Tips and Tricks Every Developer Should Know - Useful tips to enhance your JavaScript skills.
- The Evolution of JavaScript - An overview of JavaScript's journey and its latest features.
Tools:
- CodeSandbox - An online code editor for rapid prototyping and sharing of JavaScript projects.
- JSFiddle - Another online tool for experimenting with JavaScript, HTML, and CSS.
Communities:
- r/javascript on Reddit - Engage with the JavaScript community and stay updated on the latest trends.
- Stack Overflow - JavaScript - A platform to ask and find answers to JavaScript-related questions.
Explore these resources to deepen your understanding and enhance your skills in JavaScript development! Happy coding! 🚀
Practice
Task: Install a text editor (e.g., Visual Studio Code) and configure it for JavaScript development.
Task: Install Node.js on your machine and verify its installation using the terminal command node -v.