String Interpolation with Template Literals
String interpolation with template literals in JavaScript allows for the embedding of expressions within strings using backticks. This feature simplifies string handling and formatting compared to traditional single and double quotes.
Lets Go!
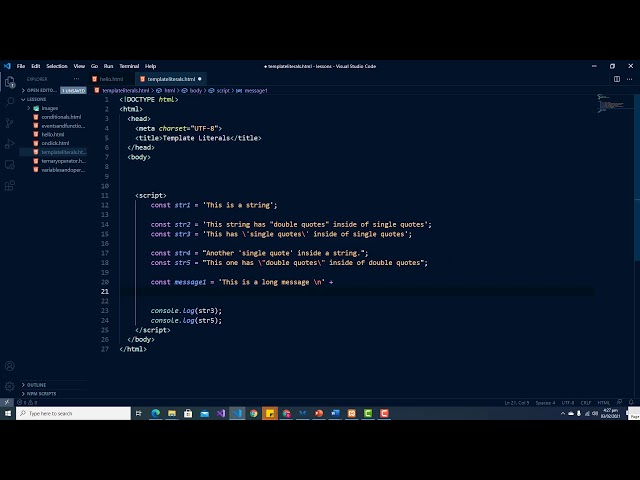
String Interpolation with Template Literals
Lesson 16
Learn how to embed expressions and variables within strings using template literals.
Get Started 🍁Introduction to Template Literals
Welcome to "Introduction to Template Literals"! In this course, we will dive deep into the powerful concept of template literals in JavaScript. Template literals allow you to use strings or embedded expressions enclosed in backticks, providing a more flexible and efficient way to work with strings compared to traditional single or double quotes.
Before we explore template literals further, we will take a step back and review how earlier versions of JavaScript implemented strings using single and double quotes. This foundational understanding will set the stage for grasping the significance and benefits of template literals.
Have you ever encountered challenges when working with strings that contain single or double quotes within them? In this course, we will address common issues like escaping characters and formatting multi-line strings, showcasing how template literals offer a seamless solution to these problems.
Additionally, we will delve into advanced usage of template literals, such as incorporating ternary operators within strings for efficient conditional statements. By the end of this course, you will have a comprehensive understanding of template literals and how they can enhance your JavaScript programming skills.
Are you ready to unlock the full potential of template literals and level up your coding expertise? Join us on this exciting journey and let's explore the world of template literals together!
Main Concepts of Template Literals
-
Template Literals: Template literals allow the use of strings or embedded expressions within a string by enclosing them in backticks. This feature simplifies string handling compared to earlier versions of JavaScript.
-
String Handling in Earlier Versions: In older versions of JavaScript, strings were usually enclosed in single or double quotes. However, there were limitations when trying to include the same type of quote inside the string, which required the use of escape characters like the backslash.
-
Multi-Line Strings: Prior to template literals, creating multi-line strings in JavaScript required concatenating strings with new line characters. Template literals simplify this process by allowing multi-line strings within backticks.
-
String Interpolation: Template literals support string interpolation, where variables or expressions can be included within the string using
${}
syntax. This allows for dynamic content based on conditions, making templating more versatile. -
Ternary Operators within Template Literals: Template literals can efficiently integrate ternary operators, enabling conditional statements directly within the string. This capability enhances the flexibility and readability of template literals, especially when dealing with conditional content.
-
Function Declarations in Template Literals: Template literals can incorporate function calls directly within the string, making it convenient to include dynamic results based on function outputs. This feature enhances the interactive and dynamic nature of template literals, enabling complex operations within strings.
Practical Applications of Template Literals
Step-by-Step Guide
-
Creating Multi-line Strings with Template Literals:
- In the video, it was shown that in older versions of JavaScript, multi-line strings were created by using newline characters and concatenation.
- To create a multi-line string with template literals:
const message = `This is a multi-line string using template literals.`; console.log(message);
- Ensure not to indent or add additional lines in the string if they are not desired to preserve formatting.
-
Embedding Ternary Operators in Template Literals:
- Template literals can be particularly useful with ternary operators, allowing conditionals within a string.
- To embed a ternary operator in a template literal:
const age = 23; const message = `You ${age > 18 ? 'can' : 'cannot'} get your driver's license since you are over 18.`; console.log(message);
- Adjust the condition based on the specific use case to display the appropriate message.
-
Using Functions in Template Literals:
- Functions can also be incorporated within template literals to dynamically display values.
- To include a function in a template literal:
const sum = (x, y) => x + y; const x = 4; const y = 100; const result = `The sum of ${x} and ${y} is ${sum(x, y)}.`; console.log(result);
- Define the function, provide parameters, and call the function within the template literal to display the calculated result.
Try It Out
- Copy and paste the provided code examples into a JavaScript file.
- Open the file in a browser console to see the output of each practical application.
- Experiment with different values and conditions to observe how template literals handle dynamic content.
Test your Knowledge
Which syntax is used for template literals in JavaScript?
Which syntax is used for template literals in JavaScript?
Advanced Insights into Template Literals
Template literals provide a powerful way to work with strings and embedded expressions in JavaScript. While they offer flexibility and ease of use, understanding some advanced concepts can enhance your skill in utilizing them effectively.
Multi-line Strings and Formatting
In earlier versions of JavaScript, creating multi-line strings involved concatenating individual lines. This process was cumbersome and prone to errors in maintaining formatting. Template literals simplify this by allowing you to span multiple lines without the need for concatenation.
Tip: To preserve formatting within template literals, avoid adding extra spaces or indents. Doing so can affect the output and may lead to unexpected results. Keeping your templates neat and concise ensures the desired presentation.
Curiosity Question: How can you efficiently manage indentation and line breaks within template literals to maintain clean and visually appealing output?
Ternary Operators within Template Literals
Template literals can seamlessly integrate ternary operators, enabling conditionals within strings. By leveraging string interpolation, you can dynamically include expressions based on specific conditions, enhancing the versatility of your templates.
Recommendation: When embedding ternary operators, ensure clarity in your conditions and outputs to avoid confusion. Clear and concise statements within your template literals enhance readability and comprehension.
Curiosity Question: How can you optimize the usage of ternary operators within template literals to streamline conditional logic and improve the clarity of your code?
Function Embedding and Expressions
Utilizing template literals extends beyond simple string interpolation, allowing for the inclusion of functions and expressions directly within your templates. This advanced feature enables dynamic computation and personalized content generation within your strings.
Expert Advice: Embrace the arrow function notation to define functions within template literals efficiently. Leveraging this notation enhances code readability and simplifies the integration of complex logic into your templates.
Curiosity Question: How can you leverage function embedding in template literals to create customized and dynamic outputs tailored to specific use cases?
By exploring these advanced aspects of template literals, you can elevate your proficiency in JavaScript string manipulation and unlock new possibilities for efficient and expressive coding practices.
Additional Resources for Template Literals
Here are some resources to further enhance your understanding of template literals and string interpolation:
-
Video Tutorial: Mastering JavaScript Template Literals
-
GitHub Repository: Exploring Advanced JavaScript Concepts
These resources will provide you with a deeper insight into template literals and how they can be used effectively in JavaScript. Happy learning!
Practice
Task: Create variables for your first name, last name, and age.
Task: Use a template literal to construct a sentence like: 'Hello, my name is [First Name] [Last Name], and I am [Age] years old.'
Task: Embed a mathematical expression inside a template literal, such as calculating your birth year.