Understanding JavaScript Data Types and Variables
JavaScript data types and variables are integral parts of programming, serving as placeholders for different values. There are five primitive data types in JavaScript, including strings, numbers, and booleans.
Lets Go!
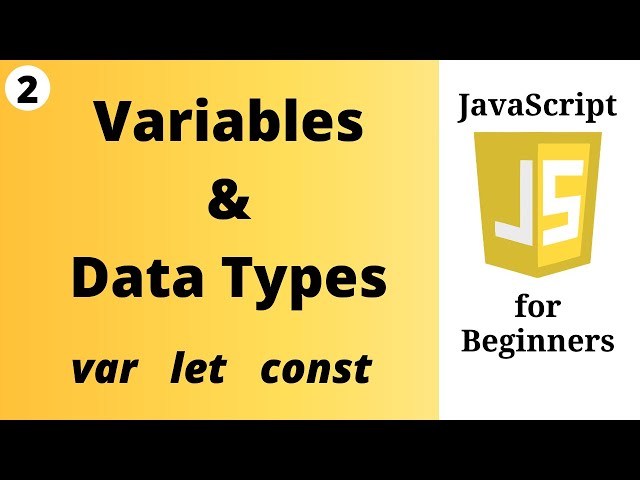
Understanding JavaScript Data Types and Variables
Lesson 5
Learn how to declare variables using var, let, and const and understand JavaScript data types such as strings, numbers, booleans, objects, and arrays.
Get Started 🍁Introduction to JavaScript Data Types and Variables
Welcome to the "Introduction to JavaScript Data Types and Variables" course! JavaScript is a powerful programming language used for creating dynamic and interactive web applications. In this course, we will explore the fundamental concepts of data types and variables in JavaScript.
Have you ever wondered how programming languages manage different types of data? In JavaScript, variables act as placeholders for various types of values, such as strings, numbers, and booleans. These data types play a crucial role in programming and allow developers to work with different kinds of information effectively.
Throughout this course, we will delve into the five primitive data types in JavaScript, including strings, numbers, and booleans. We will learn how to declare variables using the let
keyword and assign values to them using the assignment operator. Additionally, we will explore the concept of constant variables using the const
keyword and discuss the pitfalls of using the var
keyword.
By the end of this course, you will have a solid understanding of JavaScript data types and variables, enabling you to write more efficient and structured code. Get ready to dive into the world of JavaScript and unlock the potential of data manipulation and variable management. Stay tuned for an engaging learning experience ahead!
Main Concepts of JavaScript Data Types and Variables
- Variables:
- Variables act as placeholders for different types of values in programming. In JavaScript, variables are declared using keywords like
let
,var
, or `const.
- Variables act as placeholders for different types of values in programming. In JavaScript, variables are declared using keywords like
- Data Types:
- There are five primitive data types in JavaScript: strings, numbers, booleans, undefined, and null. Strings are used for characters, words, or sentences, and always need to be declared within quotation marks. Numbers are for numerical values, while booleans represent true or false values. Undefined and null are used for error handling or special cases.
- Variable Declaration:
- Use the
let
keyword to declare a variable. Make sure to assign a value using the equal sign (=
) to the variable.
- Use the
- String Variables:
- When declaring string variables, always enclose text within single or double quotation marks to differentiate them from other data types like numbers.
- Boolean Variables:
- Booleans in JavaScript use
true
orfalse
values for conditional statements or verifying the truthfulness of a statement.
- Booleans in JavaScript use
- Reserved Keywords:
- Keywords like
let
,true
,false
are reserved in JavaScript and do not require quotation marks when used as values.
- Keywords like
- Variable Scope:
- Avoid using the
var
keyword for variable declaration as it can cause problems like redeclaring the same variable, which is not allowed.
- Avoid using the
- Constant Variables:
- Use the
const
keyword to declare variables that should not be reassigned later. Attempting to change a constant variable will result in an error.
- Use the
- Best Practices:
- Use
let
for variables that may change andconst
for variables that should remain constant. Avoid usingvar
due to its potential issues with redeclaration.
- Use
Understanding these main concepts forms the basis of working with data types and variables in JavaScript, essential for effective programming practices.
Practical Applications of JavaScript Data Types and Variables
To apply the concepts discussed in the video, you can follow these steps to practice declaring variables, assigning values, and understanding different data types in JavaScript:
-
Declare and Assign a Number Variable:
- Use the
let
keyword to declare a variable. For example:let number;
- Assign a numerical value to the variable using the equal sign. For example:
number = 10;
- Save the code and log the variable to the console to see the assigned value. Verify that
10
is displayed.
- Use the
-
Declare and Assign a String Variable:
- Use the
let
keyword to declare a variable. For example:let text;
- Assign a text value within single or double quotation marks. For example:
text = "Hello, World";
- Save the code and log the variable to the console. Verify that
"Hello, World"
is displayed.
- Use the
-
Declare and Assign a Boolean Variable:
- Use the
let
keyword to declare a variable. For example:let isCorrect;
- Assign a boolean value (true or false) to the variable. For example:
isCorrect = true;
- Save the code and log the variable to the console. Verify that
true
is displayed.
- Use the
-
Understand
undefined
andnull
Data Types:- If a variable is declared but not assigned, it will have an
undefined
value. - Explicitly assign
undefined
to a variable by typingundefined
. - Assign
null
to a variable for an empty value. - Save the code and observe the values of
undefined
andnull
in the console.
- If a variable is declared but not assigned, it will have an
-
Use
var
,let
, andconst
Keywords for Variable Declaration:- Experiment with declaring variables using
var
,let
, andconst
keywords. - Note the differences in behavior, especially reassigning values.
- Understand that
var
allows redeclaration,let
allows value change, andconst
enforces immutability.
- Experiment with declaring variables using
-
Practice Mathematical Operations and String Manipulation:
- Experiment with performing calculations using numeric variables.
- Try concatenating strings or manipulating them in various ways.
- Explore how data types affect the results of operations.
By following these steps and experimenting with different variable declarations and data types, you can gain a hands-on understanding of JavaScript fundamentals. Don't hesitate to try out the code examples and see the outcomes directly in your console. Have fun exploring JavaScript data types and variables!
Test your Knowledge
Which keyword is block-scoped in JavaScript?
Which keyword is block-scoped in JavaScript?
Advanced Insights into JavaScript Data Types and Variables
In JavaScript, understanding data types and variables is crucial for programming. Let's delve deeper into some advanced aspects and insights:
Tip:
- While primitive data types like strings, numbers, and booleans are commonly used, understanding when to utilize 'undefined' and 'null' is essential for error handling and problem caching.
Recommendation:
- Avoid confusion between data types - ensure numbers are assigned without quotation marks, while strings require single or double quotes.
Expert Advice:
- When working with variables, be mindful of the assignment operator (=) - it signifies the value being assigned to a variable.
Explore further into JavaScript data types and variables - the foundation of coding mastery. What insights will you uncover next?
Additional Resources for JavaScript Data Types and Variables
For further learning on JavaScript data types and variables, consider exploring the following resources:
- MDN Web Docs on JavaScript Data Types: Link
- W3Schools JavaScript Variables Tutorial: Link
- JavaScript.info on Data Types: Link
These resources provide in-depth explanations, examples, and exercises to enhance your understanding of JavaScript data types and variables. Happy learning! 🚀
Practice
Task: Declare three variables using var, let, and const. Assign values of different data types (string, number, and boolean).
Task: Create an object representing a car with properties such as brand, model, and year. Log the object to the console.