Understanding Operators in JavaScript
Operators in JavaScript are symbols or keywords used to perform operations on variables or values. They allow you to perform arithmetic calculations, compare values, and manipulate data.
Lets Go!
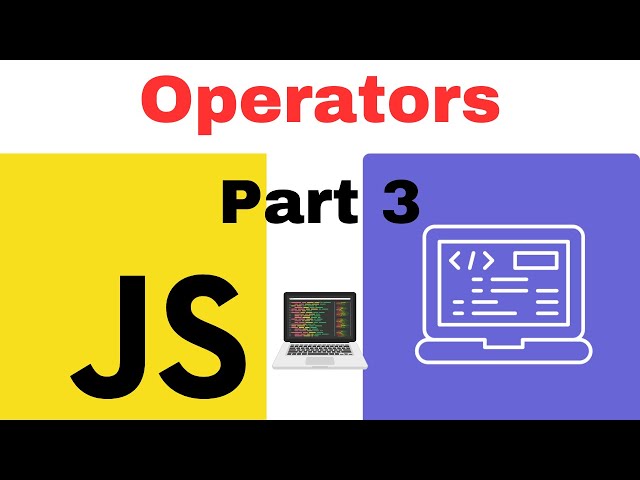
Understanding Operators in JavaScript
Lesson 6
Understand the use of arithmetic, comparison, logical, and assignment operators to perform operations and make decisions in JavaScript.
Get Started 🍁Introduction to Operators in JavaScript
Welcome to "Introduction to Operators in JavaScript", a course designed to provide you with a comprehensive overview of the essential symbols and keywords used to perform operations on variables and values in JavaScript.
In this course, we will cover various types of operators such as arithmetic operators for basic mathematical operations, comparison operators for comparing values and returning Boolean results, logical operators for logical operations on Boolean values, and assignment operators for assigning values to variables.
Have you ever wondered how JavaScript allows you to perform arithmetic calculations, compare values, and manipulate data seamlessly? Join us on this journey to delve into the world of operators in JavaScript and unlock the power of this versatile programming language.
Let's get started! 🚀
Main Concepts of JavaScript Operators
-
Arithmetic Operators: These are symbols or keywords in JavaScript that are used to perform basic mathematical operations like addition, subtraction, multiplication, and division on variables or values. For example, the
+
symbol is used for addition,-
for subtraction,*
for multiplication, and/
for division. -
Comparison Operators: In JavaScript, comparison operators are used to compare values and return a Boolean result of either true or false. This allows us to check if two values are equal (
==
or===
), if one value is greater than another (>
), if one value is less than another (<
), and so on. -
Logical Operators: These operators are used to perform logical operations on Boolean values in JavaScript. Examples of logical operators include
&&
for AND,||
for OR, and!
for NOT. These operators help us make decisions in our code based on certain conditions. -
Assignment Operators: Assignment operators in JavaScript are used to assign values to variables. The basic assignment operator is
=
, which assigns the value on the right-hand side to the variable on the left-hand side. Other assignment operators include+=
,-=
,*=
, and/=
, which perform arithmetic operations while assigning values to variables.
Practical Applications of JavaScript Operators
JavaScript operators are essential for performing various operations on variables and values. Here's how you can use different types of operators in your code:
Arithmetic Operators
- Addition (+):
let sum = 3 + 5; console.log(sum); // Output: 8
- Subtraction (-):
let difference = 10 - 3; console.log(difference); // Output: 7
- Multiplication (*):
let product = 4 * 6; console.log(product); // Output: 24
- Division (/):
let quotient = 15 / 3; console.log(quotient); // Output: 5
Comparison Operators
- Equal to (==):
let num1 = 5; let num2 = 5; console.log(num1 == num2); // Output: true
- Not equal to (!=):
let val1 = 10; let val2 = 7; console.log(val1 != val2); // Output: true
Logical Operators
- AND (&&):
let x = true; let y = false; console.log(x && y); // Output: false
- OR (||):
let a = true; let b = true; console.log(a || b); // Output: true
Assignment Operators
- Assignment (=):
let message = "Hello!"; console.log(message); // Output: Hello!
- Increment (+=):
let num = 3; num += 2; console.log(num); // Output: 5
Try out these examples in your code editor to see JavaScript operators in action! Feel free to modify the values and experiment with different combinations. Happy coding!
Test your Knowledge
What is the output of 5 + '5' in JavaScript?
What is the output of 5 + '5' in JavaScript?
Advanced Insights into JavaScript Operators
In JavaScript, operators play a crucial role in performing various operations on variables and values. Let's delve into some advanced aspects and deeper insights into how operators work in JavaScript.
Arithmetic Operators
Arithmetic operators are used for basic mathematical operations like addition, subtraction, multiplication, and division. However, they can also be used for more complex operations such as exponentiation, modulus, and increment/decrement operations.
Comparison Operators
Comparison operators are essential for evaluating conditions and comparing values. They return a Boolean true or false result based on whether the comparison is true or false. Understanding the nuances of strict equality (===
) versus loose equality (==
) can prevent unexpected outcomes in your code.
Logical Operators
Logical operators are used to perform logical operations on Boolean values. By mastering logical AND (&&
), OR (||
), and NOT (!
) operators, you can create complex conditions and make your code more efficient and readable.
Assignment Operators
Assignment operators are not only used to assign values to variables but also offer shortcuts for common operations like concatenation (+=
) and division (/=
). Understanding the subtle differences between =
and other assignment operators can prevent errors in your code.
Additional Resources for JavaScript Operators
-
MDN Web Docs: Expressions and Operators - A comprehensive guide to JavaScript operators with detailed explanations and examples.
-
W3Schools: JavaScript Operators - Provides a list of JavaScript operators with explanations and interactive examples for practice.
-
JavaScript.info: Operators - Explore different types of operators in JavaScript, along with practical examples and use cases.
-
YouTube: JavaScript Operators Explained - Watch a video tutorial explaining JavaScript operators in a simple and easy-to-understand manner.
Practice
Task: Write a script to calculate the area of a rectangle using arithmetic operators.
Task: Use comparison and logical operators to check if a number is both positive and even.