Working with Strings and String Methods
JavaScript string methods are built-in functions that allow users to manipulate and work with text, also known as strings. These methods provide various functionalities like getting the first character, finding the index of a character, getting the length of a string, trimming white spaces, converting strings to uppercase or lowercase, repeating a string, checking if a string starts or ends with a certain character, and more.
Lets Go!
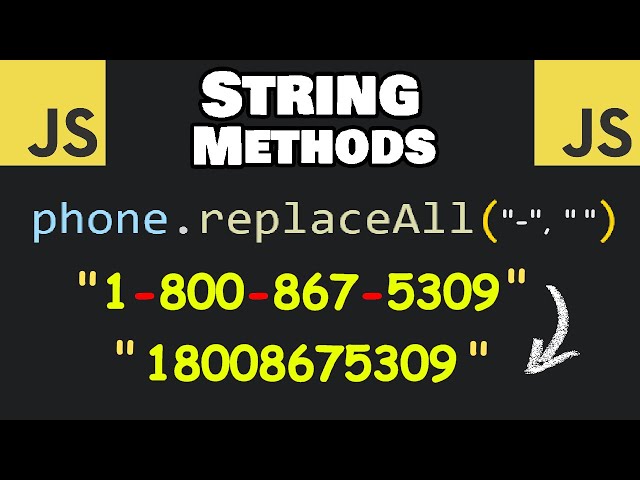
Working with Strings and String Methods
Lesson 15
Understand how to manipulate and format strings using built-in JavaScript methods
Get Started 🍁Introduction to String Methods in JavaScript
Welcome to our course on "Introduction to String Methods in JavaScript"!
Strings are essential components in programming, representing text data that can be manipulated in various ways. In this course, we will explore how to work with strings using different built-in methods in JavaScript.
Are you curious about how you can extract specific characters from a string or check if it starts with a certain character? Or maybe you want to transform a string to all uppercase or lowercase letters?
Throughout this course, we will cover key concepts such as extracting characters, finding indices, getting string length, trimming whitespace, changing case, repeating strings, and more. These methods will enable you to efficiently manipulate text data and enhance your skills as a JavaScript developer.
Join us on this journey as we delve into the world of string methods and discover the endless possibilities of working with text in JavaScript. Let's start exploring the fascinating world of string manipulation together!
Are you ready to dive in and unleash the power of string methods in JavaScript? Let's get started!
Main Concepts of String Methods in JavaScript
-
CharAt Method
- Used to get the character at a specific index in a string.
- Indexing in strings starts at 0.
- Example: Username.charAt(0) would return the first character.
-
IndexOf Method
- Returns the index of the first occurrence of a specified character in a string.
- Example: Username.indexOf('o') would return the index of the first 'o'.
-
LastIndexOf Method
- Returns the index of the last occurrence of a specified character in a string.
- Example: Username.lastIndexOf('e') would return the last index of 'e'.
-
Length Property
- Returns the number of characters in a string.
- Example: Username.length would return the length of the string.
-
Trim Method
- Removes any leading or trailing white spaces from a string.
- Example: Username.trim() would remove any white spaces from the username.
-
ToUpperCase and ToLowerCase Methods
- Changes all characters in a string to either uppercase or lowercase.
- Example: Username.toUpperCase() would convert the string to uppercase.
-
Repeat Method
- Repeats a string a specified number of times.
- Example: Username.repeat(3) would repeat the username three times.
-
StartsWith Method
- Checks if a string starts with a specified character(s) and returns a boolean.
- Example: Username.startsWith(' ') would check if the username starts with a space.
-
EndsWith Method
- Checks if a string ends with a specified character(s) and returns a boolean.
- Example: Username.endsWith('0') would check if the username ends with '0'.
-
Includes Method
- Checks if a string contains a specified substring and returns a boolean.
- Example: Username.includes(' ') would check if the username contains a space.
-
ReplaceAll Method
- Replaces all occurrences of a specified character in a string.
- Example: PhoneNumber.replaceAll('-', '') would remove all dashes from the phone number.
-
PadStart and PadEnd Methods
- Pads a string with specified characters until it reaches a desired length.
- Example: PhoneNumber.padStart(15, '0') would add zeros at the start of the phone number to make it 15 characters long.
Practical Applications of String Methods
Step 1: Getting the First Character of a String
- Create a variable called
username
and set it equal to your username or full name. - Use the
charAt
method to get the first character of the string:console.log(username.charAt(0));
Step 2: Finding the First Occurrence of a Character
- Find the index of the first occurrence of a character (e.g., 'o'):
console.log(username.indexOf('o'));
Step 3: Getting the Length of a String
- Determine the length of the string:
console.log(username.length);
Step 4: Trimming Whitespace
- Trim any whitespace before or after the string:
username = username.trim(); console.log(username);
Step 5: Changing Case
- Make the string all uppercase:
console.log(username.toUpperCase());
- Make the string all lowercase:
console.log(username.toLowerCase());
Step 6: Repeating a String
- Repeat the string a certain number of times (e.g., 3):
console.log(username.repeat(3));
Step 7: Checking if a String Starts or Ends with a Character
- Check if the string starts with a certain character (e.g., a space):
console.log(username.startsWith(' '));
- Check if the string ends with a certain character (e.g., a space):
console.log(username.endsWith(' '));
Step 8: Checking if a String Includes a Character
- Check if the string includes a certain character (e.g., a space):
console.log(username.includes(' '));
Step 9: Eliminating Dashes in a Phone Number
- Eliminate all dashes from a phone number:
phoneNumber = phoneNumber.replaceAll('-', ''); console.log(phoneNumber);
Step 10: Padding a String
- Pad the start of the string with zeros until it's 15 characters long:
console.log(phoneNumber.padStart(15, '0'));
- Pad the end of the string with zeros until it's 15 characters long:
console.log(phoneNumber.padEnd(15, '0'));
Feel free to try these practical applications with your own strings and names!
Test your Knowledge
What does the trim() method do?
What does the trim() method do?
Advanced Insights into String Methods in JavaScript
In this section, we will delve deeper into the advanced aspects of manipulating text using string methods in JavaScript. Let's explore some tips, recommendations, and expert insights to enhance your understanding of this topic.
Tips for Advanced String Manipulation:
-
Using Replace All Method: When you need to eliminate or replace specific characters in a string, the
replace all
method comes in handy. It allows you to efficiently replace all instances of a character with another one, giving you full control over string modifications. -
Padding Strings with Pad Start and Pad End: The
pad start
andpad end
methods enable you to add characters at the beginning or end of a string until it reaches a specified length. This can be useful for formatting data, such as phone numbers, with leading or trailing characters. -
Exploring Case Transformation: Experiment with
toUpperCase
andtoLowerCase
methods to convert strings entirely to uppercase or lowercase, respectively. This can be beneficial for standardizing text or implementing formatting requirements.
Recommendations for Effective String Handling:
-
Efficient Trimming with
trim
Method: Use thetrim
method to remove leading and trailing whitespace from a string, ensuring clean and concise data representation. This can be particularly useful when dealing with user inputs or external data sources. -
Enhancing Readability with Index Methods: Dive into the
charAt
,indexOf
, andlastIndexOf
methods to pinpoint specific characters or positions within a string. These methods offer valuable insights into string composition and manipulation. -
Conditional String Checking with
startsWith
andendsWith
: LeveragestartsWith
andendsWith
methods to validate string beginnings or endings based on predefined criteria. This can help streamline data validation processes and enhance program logic.
Expert Advice for Effective String Handling:
-
Optimizing String Iteration: Experiment with string iteration techniques to efficiently process text data and extract relevant information. By combining string methods creatively, you can enhance the functionality and performance of your JavaScript code.
-
Exploring String Matching and Searching: Dive deeper into advanced string searching and matching techniques to identify patterns or substrings within larger text data. This can open up new possibilities for data analysis and text processing in your projects.
Curiosity question for exploration:
- How can you leverage a combination of string methods in JavaScript to implement complex text processing tasks efficiently?
By delving into these advanced insights and recommendations, you can expand your expertise in handling text data effectively using string methods in JavaScript. Keep exploring and experimenting with different techniques to enhance your programming skills further!
Additional Resources for JavaScript String Methods
-
MDN Web Docs: String - Official documentation from Mozilla on JavaScript strings, methods, and properties.
-
JavaScript.info: Strings - Comprehensive guide on handling strings in JavaScript, including methods, examples, and best practices.
-
W3Schools: JavaScript String Methods - Interactive tutorial with examples of various string methods in JavaScript.
-
Codecademy: Learn JavaScript - Interactive course to deepen your understanding of JavaScript, including string manipulation techniques.
Explore these resources to enhance your knowledge and skills in working with strings in JavaScript. Happy coding!
Practice
Task: Create a string variable with your full name.
Task: Use methods like toUpperCase(), toLowerCase(), trim(), and slice() to manipulate the string.
Task: Replace part of the string using replace() and log the results.