Writing and Running Your First JavaScript Program
JavaScript is a popular programming language used to create interactive websites and web applications. This tutorial covers setting up the development environment, writing JavaScript programs, and running them in a web browser.
Lets Go!
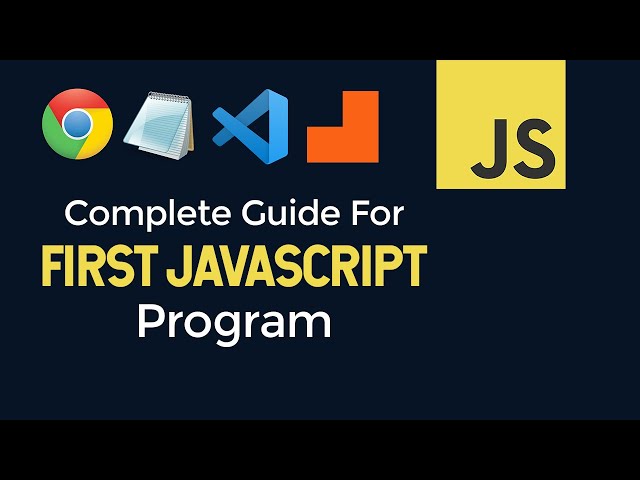
Writing and Running Your First JavaScript Program
Lesson 3
Learn how to write your first JavaScript program using tools like VS Code, run JavaScript in a web browser, and execute JavaScript within an HTML page.
Get Started 🍁Introduction to JavaScript Programming
Welcome to the "Introduction to JavaScript Programming" course by CodeAffection! In this comprehensive tutorial series, we will dive into the world of JavaScript, a powerful programming language that is widely used for creating dynamic and interactive web applications.
Before we begin writing our first JavaScript program, let's take a moment to understand the essential tools and setup required for JavaScript development. We will explore the various text editors available, such as VS Code, Sublime, Atom, and more, to write our JavaScript code efficiently.
Unlike other programming languages, JavaScript does not require a separate runtime environment software installation. Instead, we will be using a web browser, such as Google Chrome or Mozilla Firefox, which already comes equipped with a JavaScript engine to execute our programs.
Throughout this course, we will explore different ways to run JavaScript code, including using the browser console and integrating JavaScript with HTML pages. We will also discuss best practices, such as organizing JavaScript code in separate files and utilizing VS Code extensions like KoaKa for a seamless development experience.
Are you ready to embark on your JavaScript programming journey and unleash the full potential of this versatile language? Let's dive in and start writing our first JavaScript program together! 🚀
Curiosity question: Did you know you can write and run JavaScript code directly within your web browser? Let's explore this exciting feature further in the upcoming lessons!
Main Concepts of JavaScript Development
-
Text Editors for Writing JavaScript Code
- Text editors like Notepad, VS Code, Sublime, Atom, etc., can be used to write JavaScript code.
- VS Code is recommended due to the features it offers, but any text editor can be used.
-
Runtime Environment for JavaScript
- JavaScript can be executed in web browsers like Google Chrome or Mozilla Firefox.
- No separate runtime environment software installation is needed as web browsers come with a JavaScript engine.
-
IDE Extensions for JavaScript Development
- IDE extensions can be added to enhance the development process.
- Specific extensions can be mentioned when needed during development.
-
Running JavaScript Code in Web Browsers
- JavaScript code can be written and executed directly in the browser's developer console.
- Examples of performing math operations and printing messages in the console are provided.
-
Executing JavaScript in HTML Pages
- JavaScript code can be included in an HTML file using
<script>
tags within the<head>
or<body>
sections. - Separate JavaScript files can be created and linked to HTML files for better organization.
- JavaScript code can be included in an HTML file using
-
Visual Studio Code (VS Code) for JavaScript Development
- VS Code offers features like proper code formatting, syntax highlighting, and ease of use.
- Installation of VS Code is recommended for a better coding experience.
-
Using Koaka Extension for Running JavaScript
- The Koaka extension in VS Code allows running JavaScript without the need for an HTML file.
- This extension is useful for quickly testing and running JavaScript code.
-
Accessing JavaScript Notes on GitHub
- The video's instructor has shared JavaScript notes and project files on GitHub.
- Learners can access and refer to the GitHub repository for additional learning resources.
-
Upcoming Topics
- Comments in JavaScript will be discussed in the next session.
- Learners are encouraged to subscribe to the channel and share the video with others.
Practical Applications of JavaScript
Setting Up Development Environment
-
Choose a Text Editor: Select a text editor to write JavaScript code. Options include VS Code, Sublime Text, Atom, etc. Download and install VS Code if you haven't already.
-
Runtime Environment: Use a web browser like Google Chrome or Mozilla Firefox to execute JavaScript code. No separate software installation is needed.
-
IDE Extensions: Install IDE extensions to enhance development experience when required.
Writing Your First JavaScript Program
-
Running JavaScript Directly in the Browser
- Open Developer Console: Right-click on the webpage, click on "Inspect," and navigate to the Console tab.
- Execute JavaScript Operations:
5 + 3 // Outputs 8 console.log("Hello, World!"); // Outputs "Hello, World!"
-
Running JavaScript Within an HTML Page
- Create an HTML file (e.g.,
index.html
) and add JavaScript code using<script>
tags. - Execute JavaScript Code:
<script> console.log("Hello, World!"); console.log(5 + 3); // Outputs 8 </script>
- Create an HTML file (e.g.,
-
Separating JavaScript Code Into External Files
- Create a separate JavaScript file (e.g.,
script.js
) and move JavaScript code into it. - Link the script file in the HTML file using the
<script src="script.js"></script>
tag.
- Create a separate JavaScript file (e.g.,
-
Utilizing Visual Studio Code (VS Code)
- Open the project in VS Code for enhanced coding features.
- Benefit from better code formatting, indentation, and syntax highlighting.
-
Using Koaka VS Code Extension
- Install the Koaka extension for VS Code.
- Run JavaScript code directly from a file without the need for an HTML page using shortcuts provided by the extension.
GitHub Repository: Find the complete code and notes for JavaScript sessions in the GitHub project linked in the video description.
Hands-On Practice:
- Open your chosen text editor (e.g., VS Code).
- Create an HTML file with a simple structure.
- Write JavaScript code within
<script>
tags to print messages or perform mathematical operations. - Separate the JavaScript code into an external file for organization.
- Experiment with the Koaka VS Code extension to run JavaScript code without an HTML file.
Experience the immediate results and familiarize yourself with the development environment for JavaScript. Share your progress with friends to enhance learning!
Test your Knowledge
How do you display output in the browser console using JavaScript?
How do you display output in the browser console using JavaScript?
Advanced Insights into JavaScript Programming
In learning JavaScript, it is crucial to understand the nuances of setting up your development environment and the different approaches to executing JavaScript code. Let's explore some advanced insights into JavaScript programming:
Setting up Development Environment:
- Text Editors: While various text editors can be used to write JavaScript code, consider utilizing more sophisticated IDEs like Visual Studio Code (VS Code) for enhanced features.
- Runtime Environment: JavaScript can be executed in web browsers like Google Chrome or Mozilla Firefox, eliminating the need for separate runtime environment software installations.
Writing JavaScript Programs:
- IDE Extensions: Incorporating extensions can streamline development processes, improving efficiency and code quality.
Curiosity Question:
Have you explored all the features and extensions available in your preferred IDE for JavaScript development?
By understanding the intricacies of your development environment and effectively writing JavaScript programs, you'll enhance your coding experience and productivity.
Enhancing JavaScript Execution:
- Executing Code in Browser Console: Directly running JavaScript code in the browser console for quick testing and development.
- Running JavaScript in HTML: Incorporating JavaScript code within HTML files using script tags to build interactive web applications.
Curiosity Question:
How can utilizing script tags in HTML pages impact the organization and functionality of your JavaScript code?
By mastering the execution of JavaScript within different environments, you'll be equipped to create dynamic and engaging web applications effectively.
Leveraging IDE Tools:
- VS Code Integration: Utilizing Visual Studio Code for a user-friendly coding experience with features like code highlighting and proper code formatting.
- Koaka Extension: Exploring the Qoca extension in VS Code for a seamless JavaScript execution process without the need for an HTML file.
By optimizing your development environment with advanced tools and techniques, you'll elevate your JavaScript programming skills and efficiency.
Dive deeper into JavaScript programming by experimenting with different development environments, execution methods, and IDE features to gain a comprehensive understanding of this versatile language. Happy coding! 🚀
Additional Resources for JavaScript Development
-
Text Editors
-
Web Browsers
-
IDE Extensions
- JavaScript IDE extensions to make development easier.
-
JavaScript Learning
Explore these resources to enhance your understanding of JavaScript development and take your skills to the next level. Happy coding! 🚀
Practice
Task: Create a JavaScript file (hello.js) and write a script that prints 'Hello, JavaScript!' to the console.
Task: Open your browser's developer tools and run the script.
Task: Execute the same script using Node.js in