Introduction to Node.js
Learn how Node.js, a runtime environment for JavaScript, allows developers to build servers outside of a browser, utilizing the V8 engine. Discover how Node.js simplifies server-side application development for front-end developers by enabling easy integration of NPM packages.
Lets Go!
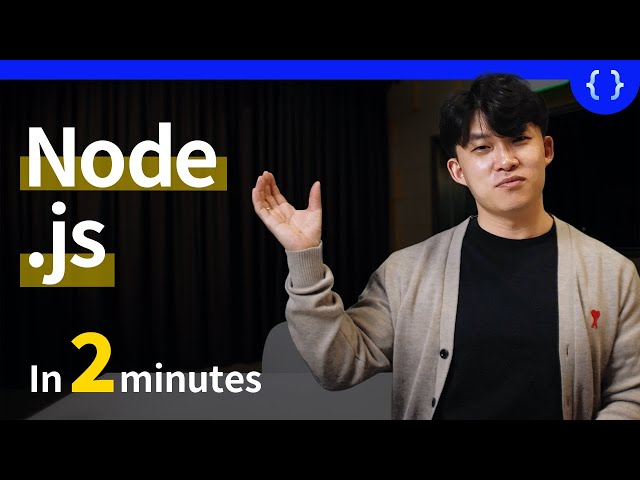
Introduction to Node.js
Lesson 1
Understand the basics of Node.js, including its event-driven, non-blocking I/O model. Learn about its use cases and ecosystem.
Get Started 🍁Introduction to Node.js for Server-Side Development
Welcome to "Introduction to Node.js for Server-Side Development"! My name is Jason K, and I'm a full stack developer working in South Korea. In this course, we will delve into the world of Node.js - a runtime environment that allows you to run JavaScript programs outside of web browsers like Firefox or Chrome.
Have you ever wondered how to build a server using JavaScript? Well, Node.js is the solution! It has gained popularity among front-end developers for enabling them to create server-side applications using a programming language they are already familiar with.
Throughout this course, we will explore the fundamentals of Node.js, including its use of the V8 engine and integration of various NPM packages. These packages are essentially blocks of code that can enhance your projects, such as calendars or charts, without the need to write everything from scratch.
By the end of this course, you will not only understand how to run a server with Node.js but also be familiar with popular frameworks like Express.js and Nest.js. Whether you are looking to enhance your web development skills or broaden your understanding of server-side technologies, this course is tailored for you.
Join me in this exciting journey into the world of Node.js, where we will build servers, handle requests, and store data in databases. Get ready to explore the limitless possibilities of server-side development with Node.js. Let's embark on this learning adventure together!
Are you ready to unleash the power of Node.js in your projects? Let's dive in and discover the endless possibilities that await us!
Main Concepts of Node.js
-
Node.js as a Runtime Environment: Node.js allows you to run JavaScript programs outside of a browser by using the V8 engine, which is the core of Google Chrome. This allows front-end developers to build server-side applications using their familiar programming language.
-
NPM Packages: Node.js allows you to use various NPM packages, which are the easiest and fastest way to use JavaScript modules. Modules are blocks of code that perform specific functions, such as building a calendar, chart, or table.
-
Integration of Modules: Once a module is registered in NPM (Node Package Manager), you can easily bring them into your project and integrate them. This allows developers to add functionalities like a calendar to a blog without having to write every line of code from scratch.
-
Popular Frameworks: Frameworks like Express.js and Nest.js have become popular choices for building applications using Node.js. Many companies in Korea use Node.js as the backbone of their server due to its ability to handle thousands of requests in a single server process.
-
Building a Server with Node.js: When writing a blog post, Node.js can handle requests and save the post to a database. When visitors access the blog, Node.js fetches the data from the server, allowing them to read the post.
Practical Applications of Node.js
Setting Up a Server with Node.js
- Install Node.js: Make sure you have Node.js installed on your computer. You can download it from the official Node.js website.
- Initialize a Node.js Project: Open your terminal and navigate to the directory where you want to create your project. Run
npm init
and follow the prompts to create apackage.json
file. - Install Express.js: To use Express.js, run
npm install express
in your terminal. Express.js is a popular framework for building web applications. - Create a Server: Write a simple Express.js server code in a file (e.g.
server.js
). Useapp.get()
to handle GET requests andapp.listen()
to start the server on a specific port. - Run the Server: In your terminal, run
node server.js
to start your Node.js server. You should see a message indicating that the server is running. - Test the Server: Open your browser and go to
http://localhost:<port>
(replace<port>
with the port number you specified). You should see your server response.
Adding Modules with NPM
- Search for Modules: Use the Node Package Manager (NPM) to search for modules that you want to add to your project. For example, you can search for a calendar module by running
npm search calendar
. - Install Modules: Once you find the module you want, install it using
npm install <module_name>
. For example, if you want to install a calendar module, runnpm install calendar
. - Integrate Modules: In your project code, require the module using
require('<module_name>')
and use its functionality as needed. For example, if you installed a calendar module, you can use its functions to display a calendar in your project.
Handling Requests and Data Persistence
- Create Routes: Define routes in your Express.js server to handle different types of requests (e.g. GET, POST).
- Handle Requests: Write code in your routes to handle incoming requests. For example, to save a blog post to a database, you can create a route that accepts POST requests and saves the data.
- Fetch Data: Implement routes to fetch data from your database when users access your blog. Use database queries to retrieve the necessary information and send it back to the client.
Stay Curious and Keep Learning
If you have any questions or specific topics you want to learn more about, feel free to leave a comment below. Let's continue exploring the world of Node.js together!
Test your Knowledge
What is Node.js primarily used for?
What is Node.js primarily used for?
Advanced Insights into Building Servers with Node.js
Now that we understand the basics of building a server using Node.js, let's delve into some advanced insights that can enhance your development skills:
-
Runtime Environment: Node.js serves as a runtime environment, enabling JavaScript programs to run outside of traditional web browsers like Firefox or Chrome. This flexibility empowers developers to leverage their JavaScript skills in server-side application development.
-
NPM Packages: Node Package Manager (NPM) is a vast repository of JavaScript modules that can be easily integrated into your projects. Modules, such as calendars, charts, or tables, can be quickly added to your application by installing the corresponding NPM packages. This streamlines the development process and saves time by utilizing existing code modules.
-
Popular Frameworks: Explore popular frameworks like Express.js and Nest.js, which have gained traction in the development community. These frameworks provide handy tools and features to streamline server-side development in Node.js applications.
-
Scalability: Node.js excels in handling multiple requests simultaneously within a single process. This scalability feature makes it ideal for applications that require handling a high volume of requests efficiently.
Curiosity Question: Have you explored any unique NPM packages for enhancing your Node.js projects?
By harnessing these advanced insights, you can elevate your Node.js server development skills and create robust applications with ease. Keep exploring new packages and frameworks to stay updated with the evolving landscape of JavaScript development.
Additional Resources for Node.js Server Development
-
Node.js Official Website
Explore the official website of Node.js to learn more about its capabilities, features, and documentation: Node.js -
Express.js Documentation
Dive into the documentation of Express.js, a popular web application framework for Node.js: Express.js -
Nest.js Framework
Discover Nest.js, a progressive Node.js framework for building efficient, reliable, and scalable server-side applications: Nest.js -
npm (Node Package Manager)
Learn more about npm, the package manager for JavaScript and the world's largest software registry: npm -
Node.js Tutorials on YouTube
Watch tutorials on Node.js server development on YouTube to enhance your understanding and practical skills: Node.js Tutorials
By exploring these additional resources, you can expand your knowledge and proficiency in building server-side applications with Node.js. Feel free to ask any specific questions or share your insights in the comments below. Happy learning!
Practice
Task: Write a brief explanation of Node.js in your own words.
Task: Research and list three real-world applications built with Node.js.