Node.js Core Modules
Learn about the core modules in Node.js, including Path, OS, HTTP, and FS modules. Understand how to work with file paths, interact with the operating system, transmit data over HTTP, and manage the file system.
Lets Go!
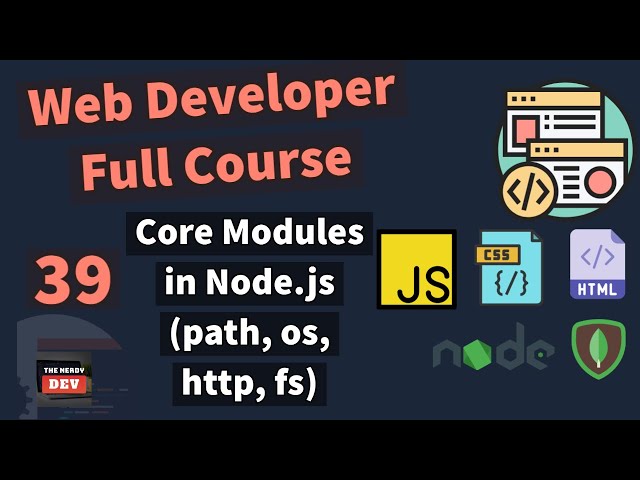
Node.js Core Modules
Lesson 4
Gain familiarity with Node.js core modules (fs, path, os, and events) and their key functionalities.
Get Started 🍁Introduction to Node.js Core Modules
Welcome to "Introduction to Node.js Core Modules"! In this course, we will delve into the fundamental modules of Node.js that serve as building blocks for developing robust applications.
Background:
In the previous video, we explored modules in Node.js, understanding how each file in a Node.js application is considered a module. We discussed how variables and functions within a module are scoped to that file and how they can be exported to other modules using the exports
object.
Essential Setup: Before diving into this course, it would be beneficial to have a basic understanding of JavaScript and Node.js setup on your machine.
Curiosity Question: Have you ever wondered how Node.js allows you to work with file paths and directories effortlessly?
In this course, we will explore key core modules like:
- The
path
module for working with file and directory paths. - The
os
module for operating system-related utility methods and properties. - The
http
module for networking in Node.js, enabling data transmission over the HTTP protocol. - The
fs
module for interacting with the file system, including reading, writing, renaming, and deleting files.
Get ready to unlock the power of Node.js core modules and elevate your development skills! Let's embark on this exciting journey together.
Main Concepts of Node.js Modules
-
Modules in Node.js: Every file defined in a Node.js application is considered a module. Variables and functions within each module are scoped to that file.
-
Exporting Functionality: Variables and functions of a module are not available outside the module unless explicitly exported. The
exports
object is used to export functionalities to other modules. -
Importing Functionality: To use a variable or function from another module, it needs to be explicitly exported first. Then, it can be imported using the
required
function. -
Core Modules: There are several important core modules in Node.js. Some of them include:
- Path Module: Provides utilities for working with file and directory paths. It exposes methods like
dirname
andextname
. - OS Module: Provides operating system-related utility methods and properties. It exposes methods like
eol
,arch
,freemem
,totalmem
, andversion
. - Events Module: Deals with the concept of event emitters. Covered in a separate video.
- HTTP Module: Used for networking in Node.js to transmit data over the HTTP protocol. It exposes methods like
methods
andstatuscodes
. - FS Module: Stands for File System, allows interaction with the file system. It exposes methods like
readFile
,writeFile
,appendFile
,unlink
,rename
,mkdir
, andrmdir
.
- Path Module: Provides utilities for working with file and directory paths. It exposes methods like
-
Creating a Simple HTTP Server: Demonstrated by using the
createServer
method and callbacks to handle incoming requests and serve responses. -
Working with the File System: Methods like
readFile
,writeFile
,appendFile
,unlink
, andrename
demonstrated for efficient file manipulation. -
Synchronous Versions: All the asynchronous file-related methods have synchronous versions for simpler control flow.
-
Creating and Deleting Directories: The
mkdir
method creates a directory, while thermdir
method deletes it.
By understanding these main concepts, learners can effectively work with modules, core modules, file system operations, and HTTP servers in Node.js.
Practical Applications of Node.js Core Modules
Let's dive into the practical applications of some of the core modules discussed in the video. Follow these steps to experiment with the functionalities provided by these modules:
1. Path Module
- Create a new file in the node demos folder and name it as
path-demo.js
.
const path = require('path'); console.log(path.dirname(__filename)); // Returns the directory name for the current file console.log(path.extname('path-demo.js')); // Returns the extension of the file
- Run the command
node path-demo.js
in the terminal to see the outputs.
2. OS Module
- Create a new file in the node demos folder and name it as
os-demo.js
.
const os = require('os'); console.log(os.arch()); // Returns the underlying CPU architecture console.log(os.freemem()); // Returns the amount of free system memory in bytes
- Run the command
node os-demo.js
in the terminal to see the outputs.
3. HTTP Module
- Create a new file in the node demos folder and name it as
http-demo.js
.
const http = require('http'); console.log(http.METHODS); // Lists all HTTP methods supported console.log(http.STATUS_CODES); // Lists all HTTP status codes and descriptions
- Run the command
node http-demo.js
in the terminal to see the outputs.
4. File System (FS) Module
- Create a new file in the node demos folder and name it as
fs-demo.js
.
const fs = require('fs'); fs.readFile('dummy.html', (error, data) => { if(error) throw error; console.log(data); });
- Create an
dummy.html
file in the same folder, add some content, and run the commandnode fs-demo.js
in the terminal to read the file content.
These steps will help you to interact with and utilize the functionalities offered by the core modules in Node.js. Don't forget to experiment with different methods and properties to enhance your understanding. Happy coding! 🚀
Test your Knowledge
Which module is used for working with the filesystem in Node.js?
Which module is used for working with the filesystem in Node.js?
Advanced Insights into Node.js Modules
In the previous video, we delved into the basics of modules in Node.js, understanding how variables and functions within each module are scoped to that file. We also learned how to export these functionalities for use in other modules. Now, let's explore some essential core modules in Node.js for advanced insights.
Path Core Module
The path
module provides utilities for working with file and directory paths in Node.js. By importing the path
module using require
, we can access various methods like dirname
and extname
to manipulate paths efficiently.
path.dirname()
: Returns the directory name for a given path.path.extname()
: Retrieves the extension of a file path.
Curiosity Question: Can you think of scenarios where manipulating directory names and file extensions can be beneficial in your Node.js applications?
OS Core Module
The os
core module in Node.js offers operating system-related utility methods and properties. By requiring the os
module, we can access information like CPU architecture, memory statistics, and the operating system version.
os.arch()
: Provides the CPU architecture information.os.freemem()
&os.totalmem()
: Yield the free and total system memory, respectively.os.version()
: Retrieves the operating system version.
Curiosity Question: How can understanding operating system-related information benefit your Node.js applications in terms of performance optimization and resource management?
HTTP Core Module
The http
core module allows Node.js to transmit data over the HTTP protocol, essential for networking tasks. By utilizing methods like methods
and statuses
, we can interact with HTTP methods and status codes in our applications.
http.methods
: Lists all supported HTTP methods.http.statusCodes
: Displays HTTP status codes with descriptions.
Recommendation: Experiment with creating an HTTP server using createServer
to handle incoming requests and serve responses, understanding the fundamentals of web communication in Node.js.
FS Core Module
The fs
core module, standing for the file system, enables interaction with files and directories in Node.js applications. Methods like readFile
, writeFile
, and unlink
provide functionality for reading, writing, and deleting files programmatically.
fs.readFile()
&fs.writeFile()
: Read and write file contents.fs.unlink()
: Delete a specified file.
Curiosity Question: How can using synchronous versions of file system methods improve or impact the performance of your Node.js application's file operations?
Additional Exploration
For further understanding and experimentation, explore synchronous versions of file system methods, methods for updating files, and creating and removing directories using the fs
core module.
By diving deeper into these core modules and their functionalities, you can enhance your Node.js skills and build robust applications. Remember to refer to the official Node.js documentation for comprehensive guides on each module.
Feel free to engage with the modules, experiment with different methods, and unleash the full potential of Node.js in your projects! 🚀
Additional Resources for Node.js Core Modules
- Node.js Official Documentation on Path Module
- Learn more about working with file and directory paths using the Path core module.
- Node.js Official Documentation on OS Module
- Explore operating system related utility methods and properties using the OS core module.
- Node.js Official Documentation on HTTP Module
- Understand how to transmit data over the HTTP protocol using the HTTP core module.
- Node.js Official Documentation on FS Module
- Interact with the file system and perform various file operations using the FS core module.
- Node.js Events Module
- Dive deeper into the concept of event emitters with Node.js' Events Module.
- Node.js Core Modules Guide
- Explore a comprehensive guide covering the Node.js core modules discussed in the video.
Feel free to explore these resources to enhance your understanding of Node.js core modules and take your knowledge to the next level! 🚀
Practice
Task: Create a script that:
- Reads a file using the
fs
module. - Prints the file's path using the
path
module. - Logs system information (e.g., OS type) using the
os
module. - Triggers a custom event using the
events
module.