Writing your first Node.js program
Node.js is an open-source, cross-platform JavaScript runtime environment that allows you to run JavaScript code outside of a web browser. In this tutorial, you will learn how to create your first Node.js application and read inputs from the terminal using the readline module.
Lets Go!
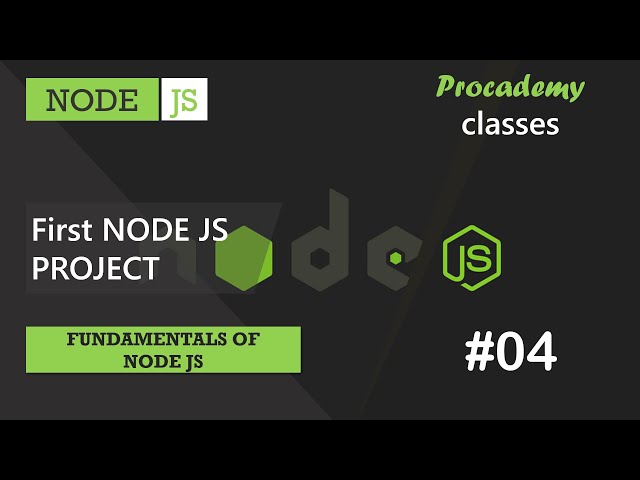
Writing your first Node.js program
Lesson 3
Write and execute a simple "Hello, World!" program using Node.js.
Get Started 🍁Introduction to Node.js Basics
Welcome to the "Introduction to Node.js Basics" course! In this course, we will delve into the fundamentals of Node.js, a powerful tool that allows you to execute JavaScript code outside of the browser.
Are you ready to explore how to create your first Node.js application and harness the capabilities of this versatile environment? Have you ever wondered how to run JavaScript code in a server-side environment with ease? If so, this course is the perfect starting point for you!
Course Overview
Throughout this course, you will learn how to create Node.js applications, interact with the terminal, read user input, and work with files. We will start by setting up our Node.js environment and creating our first Node.js application. As we progress, we will cover essential concepts like using console.log
to output messages to the terminal and leveraging modules like readline
for user input.
By the end of this course, you will have a solid foundation in Node.js basics, equipping you with the knowledge and skills to build robust applications and dive deeper into advanced Node.js topics.
Get ready to embark on a journey into the world of Node.js! Are you excited to get started? Let's begin our exploration together.
Main Concepts of Node.js Basics
-
Creating a Node.js Application:
- Node.js allows developers to execute JavaScript code outside of the browser environment.
- To create a Node.js application, you need to set up a folder and create a
.js
file (e.g.,app.js
) for writing Node.js code.
-
Printing Output to Terminal:
- Use
console.log()
to display messages in the terminal. - Ensure to save the file before executing it to see the updated output.
- Use
-
Reading User Input from Terminal:
- Node.js does not natively support reading terminal input, but you can import the
readline
module. - Create an interface using
createInterface()
method and specify input (process.stdin
) and output (process.stdout
). - Use
question()
method to prompt the user for input and handle the input using a callback function.
- Node.js does not natively support reading terminal input, but you can import the
-
Closing the User Interface:
- After capturing user input, close the interface using the
close()
method. - Listen for the
close
event withon()
method and perform actions when the interface is closed, e.g., logging a message and exiting the process usingprocess.exit()
.
- After capturing user input, close the interface using the
By understanding these core concepts, you can begin to explore the capabilities of Node.js in handling input and output operations effectively.
Practical Applications of Node.js Basics
Step-by-Step Guide
- Setting Up the Environment: Ensure you have Node.js installed and configured on your system.
- Creating a Node.js Application:
- Open Visual Studio Code and create a new folder for your Node.js files.
- Create a new file inside this folder and name it
app.js
. - Write your Node.js code in this file, starting with printing messages to the console using
console.log()
.
console.log("Hello from Node.js");
-
Executing the Node.js Application:
- Open the built-in terminal in Visual Studio Code.
- Type the command
node app.js
and press enter to execute your Node.js file. - Remember to save your file before running it to see the desired output.
-
Reading User Input:
- Remove the existing code in
app.js
. - Import the
readline
module using therequire
function. - Create an interface to read user input by setting up the input and output properties.
- Remove the existing code in
const readline = require('readline'); const rl = readline.createInterface({ input: process.stdin, output: process.stdout });
- Prompting User Input:
- Use the
rl.question()
method to prompt the user to enter a value. - Define a callback function to handle the input provided by the user.
- Use the
rl.question('Please enter your name: ', (name) => { console.log('You entered: ' + name); });
- Closing the Interface:
- Close the interface once the input is received and processed to prevent it from lingering.
- Listen for the
close
event on the interface and define logic to execute when it occurs.
rl.on('close', () => { console.log('Interface closed'); process.exit(0); });
-
Running the Updated Node.js Application:
- Save your changes in
app.js
and run the file usingnode app.js
. - Enter your name when prompted and observe the output, including the closure of the interface.
- Save your changes in
-
Experiment and Explore:
- Modify the code to interact with users in different ways or handle additional input scenarios.
- Explore further capabilities of Node.js by working with files, as mentioned in the video transcript.
Hands-on Practice
- Create a new Node.js file in Visual Studio Code.
- Follow the steps above to print messages to the console and read user input.
- Experiment with different prompts and explore additional functionalities of the
readline
module. - Share your experience and any challenges faced while working on this practical application. Have fun coding with Node.js!
Test your Knowledge
Which command is used to execute a Node.js program?
Which command is used to execute a Node.js program?
Advanced Insights into Node.js Basics
After successfully setting up Node.js and executing JavaScript code outside the browser, it's essential to delve deeper into creating Node.js applications. One crucial aspect to remember is the importance of saving your files before executing them. Node.js files must be saved to ensure that the changes you make are reflected when you run the program. Always remember to save your files to avoid unwanted results.
Pro Tip: Before executing a Node.js file, make it a habit to save your changes first to ensure that all modifications are applied.
Curiosity: Why is it crucial to save a Node.js file before executing it, and what potential issues can arise if this step is overlooked?
One fascinating feature of Node.js is the ability to read input from users through the terminal. While Node.js doesn't have a built-in function for this, you can utilize modules provided by Node.js for such tasks. The "readline" module in Node.js allows you to create an interface to read inputs from the terminal efficiently.
Expert Advice: Utilize the "readline" module in Node.js to interact with users via the terminal, offering a dynamic and interactive experience within your applications.
Curiosity: How can the "readline" module in Node.js enhance the user experience in your applications, and what are the benefits of interactive input capabilities?
In addition to reading user input, you can further enhance your Node.js applications by optimizing the closing of interfaces. When users have interacted with your program and the interface needs to be closed, listening to the "close" event and executing specific actions can streamline the user experience and application flow.
Recommendation: Handle interface closing events efficiently to provide a seamless user experience and ensure proper termination of processes within your Node.js applications.
Curiosity: What are the advantages of listening to and handling "close" events in Node.js applications, and how can this improve the overall functionality and user satisfaction of your programs?
Explore these advanced insights in Node.js to elevate your skills and create more robust and interactive applications. Stay curious, experiment with different functionalities, and continue expanding your knowledge in Node.js development.
Additional Resources for Node.js Basics
- Node.js Official Documentation
- Node.js Tutorial for Beginners
- Introduction to Node.js on FreeCodeCamp
- Node.js Crash Course on Traversy Media
- Node.js Learning Path on Udemy
Explore these resources to enhance your understanding of Node.js basics and take your skills to the next level! Happy learning! 🚀
Practice
Task: Create a JavaScript file named app.js with the following content:
console.log('Hello, World!');
- Run the file using node app.js in the terminal.