Basic Operators (Arithmetic, Comparison, Logical, etc.)
Ruby operators are essential for performing mathematical operations, comparisons, assignments, logical operations, ternary operations, range operations, definition checks, scope resolution, and bitwise operations in Ruby programming. This comprehensive guide covers the different types of Ruby operators and provides real examples for each type.
Lets Go!
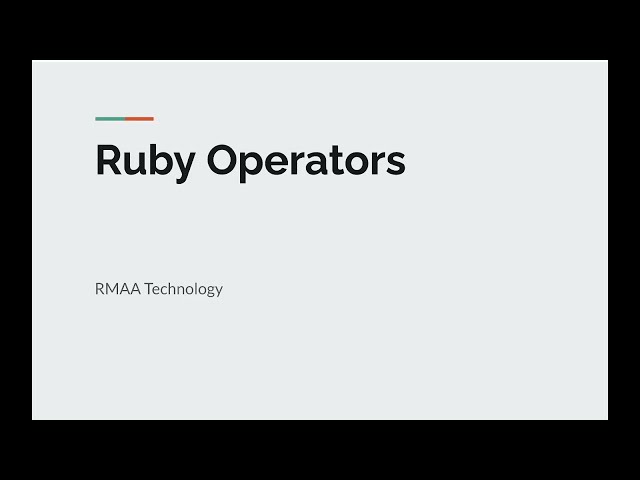
Basic Operators (Arithmetic, Comparison, Logical, etc.)
Lesson 8
Explore arithmetic, comparison, and logical operators in Ruby and their use in expressions.
Get Started 🍁Introduction to Ruby Operators
Welcome to the course "Introduction to Ruby Operators"! In this comprehensive course, we will delve into the world of Ruby operators, exploring different types of operators, their functionalities, and how to use them in a Ruby program with real examples.
Background Information:
Ruby operators are essential elements in performing various tasks within a Ruby program. From arithmetic operations to logical comparisons, assignment, ternary operations, range creation, defined checks, and bitwise operations, operators play a crucial role in manipulating data and controlling program flow.
Course Overview:
- Arithmetic Operators: Perform mathematical operations like addition, subtraction, multiplication, division, modulus, and exponentiation.
- Comparison Operators: Compare values to determine equality, inequality, greater than, less than, and other relational operations.
- Assignment Operators: Assign values to variables and perform compound operations.
- Logical Operators: Combine conditions using AND, OR, and NOT operators to make logical decisions.
- Ternary Operator: Evaluate conditions to choose between two expressions.
- Range Operator: Create sequences of elements within a specific range.
- Defined Operator: Check if a variable or function is defined in a Ruby program.
- Scope Resolution Operator: Resolve scope within classes, objects, and modules.
- Bitwise Operators: Perform operations on binary representations of integers.
Prerequisites:
This course assumes basic knowledge of programming concepts and familiarity with Ruby syntax. It is recommended for beginners and intermediate Ruby programmers looking to enhance their understanding of operators in Ruby.
Curiosity Question:
Have you ever wondered how operators like "+", "-", "*", and "/" work behind the scenes in a Ruby program? Let's explore and unravel the magic of Ruby operators together!
Join us on this exciting journey to master Ruby operators and unlock the full potential of your Ruby programming skills. Let's dive in and start exploring the fascinating world of Ruby operators! 💻🚀🔍
Are you ready to embark on this learning adventure? Let's get started! 🎉
Main Concepts of Ruby Operators
-
Arithmetic Operators: These operators allow us to perform mathematical operations in a Ruby program. They include addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**).
-
Comparison Operators: Used to compare values, such as checking for equality (==), inequality (!=), less than (<), greater than (>), less than or equal to (<=), and greater than or equal to (>=).
-
Assignment Operators: Used to assign values to variables, such as equal (=), incremental (+=), decremental (-=), multiplication (*=), division (/=), and modulus (%=).
-
Logical Operators: Used to combine two or more conditions. Include AND (&&), OR (||), and NOT (!).
-
Ternary Operator: Represented by a question mark (?) and a colon (:). It has an evaluation expression followed by two statements; the result determines which statement is executed.
-
Range Operator: Helps create a range of elements. Can be inclusive (..) or exclusive (...), defining a sequence of values.
-
Defined Operator: Checks if a variable or function is defined in a Ruby program.
-
Scope Resolution Operator: Represents by a double colon (::), used to specify the scope of class or module.
-
Bitwise Operators: Work directly on binary representation of integers. Include AND (&), OR (|), XOR (^), left shift (<<), and right shift (>>).
By understanding these concepts and practicing with real examples, learners can effectively use Ruby operators in their programs. Feel free to experiment with different numbers and combinations to deepen your understanding.
Practical Applications of Ruby Operators
Example: Arithmetic Operators
-
Addition: To perform addition in Ruby, use the
+
symbol.
Try it out:4 + 2
-
Subtraction: For subtraction, use the
-
symbol.
Try it out:4 - 2
-
Multiplication: Use the
*
symbol for multiplication.
Try it out:4 * 2
-
Division: Perform division using the
/
symbol.
Try it out:4 / 2
-
Modulus: Get the remainder of a division with the
%
symbol.
Try it out:4 % 2
-
Exponent: Calculate the power with
**
symbol.
Try it out:4 ** 2
Try the above examples interactively using IRB!
Example: Comparison Operators
-
Equality Check: Use
==
to check if two values are equal.
Try it out:4 == 2
-
Inequality Check: Verify if two values are not equal with
!=
.
Try it out:4 != 2
-
Less Than: Use
<
to check if one number is less than another.
Try it out:4 < 2
-
Greater Than: Determine if one number is greater than another with
>
.
Try it out:4 > 2
Experiment with different values to see the results in action!
Interactive Practice:
- Open your IRB (Interactive Ruby) console.
- Copy and paste the provided examples for various operators.
- Modify the numbers in the examples to create your custom exercises.
- Play around with different operators and values to become familiar with Ruby's operator functionalities.
Test your Knowledge
Which of the following is NOT an arithmetic operator in Ruby?
Which of the following is NOT an arithmetic operator in Ruby?
Advanced Insights into Ruby Operators
In Ruby programming, operators play a crucial role in performing various operations. Let's delve into some advanced insights that will enhance your understanding of operators in Ruby.
Arithmetic Operators
Arithmetic operators are fundamental in performing mathematical operations in Ruby. Beyond the basic addition, subtraction, multiplication, and division, you can leverage modulus (%) to obtain the remainder of a division. For example, what would be the result of 4 % 3? Think about it!
Comparison Operators
Comparison operators are used to compare values in Ruby. Understanding the nuances of equality (==), inequality (!=), greater than (>), and less than (<) can help you create intricate conditional statements. Could you craft a complex condition involving multiple comparison operators to test your skills?
Logical Operators
Logical operators are employed for combining conditions in Ruby. By mastering logical AND (&&), OR (||), and NOT (!) operations, you can build sophisticated logic structures in your code. Can you create a scenario where a complex logical operation determines the outcome?
Bitwise Operators
Bitwise operators work directly at the binary level in Ruby, making them powerful tools for handling bitwise operations. Exploring AND (&), OR (|), XOR (^), and left/right shift operators (<<, >>) can open up possibilities for optimizing code. How would you use bitwise operators to efficiently manipulate binary data?
Ternary Operator
The ternary operator (?:) offers a concise way to make decisions based on conditions. By understanding its structure and application, you can streamline your code logic. Can you devise a scenario where the ternary operator simplifies a decision-making process in your program?
Range Operator
Range operators are essential for creating sequences of elements in Ruby. Aside from generating numerical ranges, you can experiment with character ranges and custom data types. How would you utilize range operators to iterate over a specific range of data effectively?
Defined Operator and Scope Resolution Operator
The defined operator helps ensure the existence of variables or functions in your Ruby program, offering a safeguard against undefined entities. On the other hand, the scope resolution operator (::) aids in accessing class constants and methods across modules.
By exploring these advanced insights into Ruby operators, you'll not only deepen your proficiency in using them but also unlock creative ways to solve programming challenges. Embrace the diversity and flexibility of Ruby operators to empower your coding journey further!
Additional Resources for Ruby Operators
Explore these resources to enhance your understanding of Ruby operators and take your programming skills to the next level:
- Ruby Operator Precedence Table - A comprehensive guide to understand the precedence of different operators in Ruby.
- Ruby Operators Cheat Sheet - A quick reference guide that summarizes the various operators in Ruby with examples.
- Ruby Bitwise Operators - Dive deep into how bitwise operators work in Ruby and explore advanced use cases.
- Ruby Range Operators - Learn more about how range operators are used to create sequences of values in Ruby.
- Ruby Ternary Operator Explained - Understand how ternary operators work in Ruby and how they can be utilized effectively in your code.
By exploring these resources, you can gain a deeper insight into Ruby operators and improve your proficiency in programming with Ruby. Happy learning!
Practice
Task: Write a script that calculates the area of a rectangle using arithmetic operators.
Create a program that compares two user inputs and prints whether they are equal, greater, or lesser.