Ruby Constants
Constants in Ruby are used to store static data that should not be modified during the program's execution. They are indicated by capital letters in the variable name.
Lets Go!
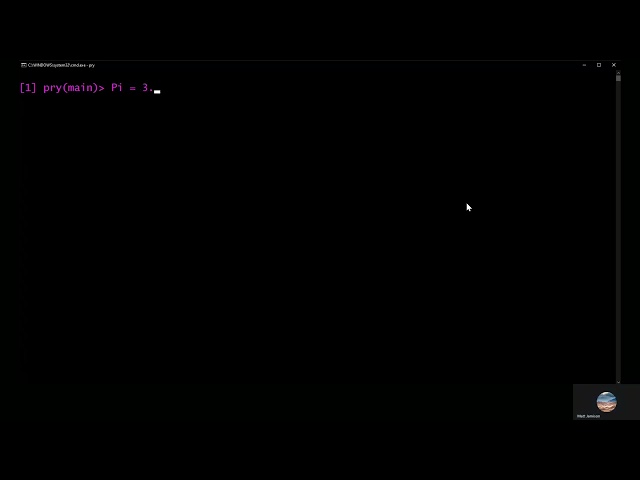
Ruby Constants
Lesson 7
Understand the purpose and usage of constants in Ruby, including naming conventions and immutability.
Get Started 🍁Introduction to Constants in Ruby
Welcome to "Introduction to Constants in Ruby"! Have you ever wondered how to incorporate static data into your programs effectively? In this course, we will explore the essential concept of constants in Ruby programming.
Constants are valuable for storing values that remain unchanged throughout the program's execution, such as the mathematical constant Pi. By using constants, we can ensure consistency and readability in our codebase.
Throughout this course, we will cover the fundamentals of constants in Ruby, including how to declare and utilize them in your programs. We will also delve into the importance of maintaining the integrity of constants and the potential consequences of reassigning their values.
By the end of this course, you will have a solid understanding of how constants work in Ruby and how they contribute to the overall structure of your programs. Get ready to enhance your Ruby programming skills and discover the power of constants in your coding journey!
Main Concepts of Constants in Ruby
-
Constants in Ruby: Constants are used to store values that do not change throughout the program's execution, such as the value of pi. They are indicated by using all capital letters in the variable name.
-
Usage of Constants: While it is not common to put static data into a program, there are instances where constants are useful. For example, the value of pi is a constant because it never changes.
-
Defining Constants: To define a constant in Ruby, you can assign a value to it using the
=
operator. For example,PI = 3.14
would define a constant namedPI
with a value of 3.14. -
Overwriting Constants: In Ruby, it is possible to overwrite the value of a constant during the program's execution. However, this practice is not advisable because constants are meant to be immutable.
-
Class Names as Constants: In Ruby, class names also start with a capital letter because they are treated as constants within the program. This is why it is important to follow naming conventions when defining classes in Ruby.
Practical Applications of Constants in Ruby
Constants in Ruby are useful for storing data that should not be changed throughout the program. One common example is using a constant for the value of pi. Let's create a constant for pi and practice using it in Ruby.
Step 1: Define a Constant for Pi
PI = 3.14
In this step, we set the constant PI
equal to the value of pi, which is 3.14.
Step 2: Try to Override the Constant (Not Recommended)
PI = 4
Even though it's not advisable, in this step, we attempt to overwrite the value of PI
with 4. Remember, Ruby allows you to do this, but it will issue a warning.
Step 3: Check the Value of Pi
puts PI
After attempting to overwrite the constant, check the value of PI
using puts PI
. If overridden successfully, it will display the new value; otherwise, it will show the original value.
By following these steps, you can understand the concept of constants in Ruby and how to use them in your programs effectively. Make sure to experiment and try changing the constant value to see how Ruby behaves. Have fun coding!
Test your Knowledge
How are constants identified in Ruby?
How are constants identified in Ruby?
Advanced Insights into Ruby Constants
In programming, constants are used to store values that do not change during the execution of a program. While it is best practice to avoid putting static data into your code whenever possible, there are cases where constants are necessary. For example, the value of pi (π) is a commonly used constant in mathematical calculations.
In Ruby, constants are indicated by using capital letters in the variable name. For instance, you can define a constant for pi like so: PI = 3.14
. However, it is important to note that Ruby does allow you to overwrite the value of a constant in your program. This could lead to unexpected behavior, so it is not advisable to do so.
If a constant is redefined in your program, Ruby will issue a warning indicating that the constant has already been initialized. Despite this warning, the new value will be used if referenced in the code. It's essential to be cautious when working with constants to avoid unintentional changes to your program's logic.
Furthermore, in Ruby, class names are also considered constants within the program. This is why class names in Ruby typically start with a capital letter. Understanding the relationship between constants and class names can help you structure your code more effectively and maintain consistency in your programming practices.
Tip:
Avoid redefining constants in your Ruby programs to prevent unexpected behavior and maintain code integrity.
Additional Resources for Constants in Ruby Programming
-
Article: Understanding Constants in Ruby - A detailed explanation of how constants work in Ruby and best practices for utilizing them in your code.
-
Documentation: Ruby Core Docs - Constants - The official Ruby documentation on constants, providing in-depth information and examples.
-
Tutorial: Ruby Constants and Variables - A step-by-step guide on using constants in Ruby programming, including practical examples and exercises.
-
Video: Understanding Constants in Ruby - A video tutorial explaining the concept of constants in Ruby and how to effectively use them in your projects.
Explore these resources to deepen your understanding of constants in Ruby programming and enhance your coding skills. Happy learning! 🚀
Practice
Task: Define constants to store application settings like version and company name.
Task: Write a script that outputs these constants.