Ruby Syntax
Ruby syntax refers to the rules and arrangement of words in the Ruby programming language to form well-formed sentences or statements. This includes identifiers, variables, constants, methods, and commands that are essential for writing code in Ruby.
Lets Go!
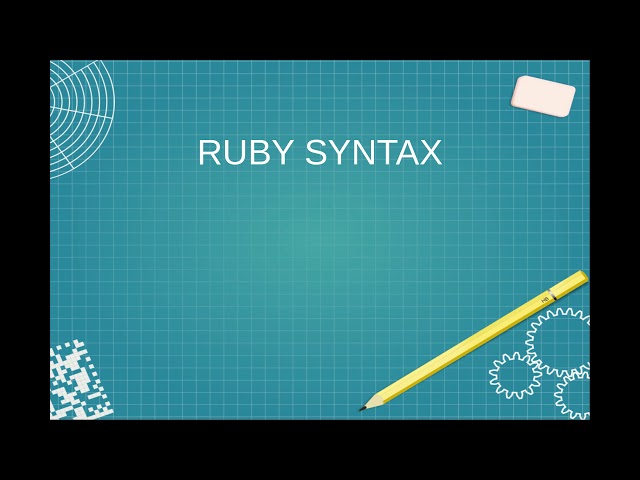
Ruby Syntax
Lesson 5
Understand the basic syntax rules in Ruby, including indentation, keywords, and operators.
Get Started 🍁Introduction to Ruby Syntax
Welcome to the "Introduction to Ruby Syntax" course! In this course, we will delve into the fundamental rules and conventions that govern the syntax of Ruby programming language. Understanding syntax is crucial as it forms the foundation for constructing well-formed sentences and statements in a programming language.
Have you ever wondered how variables, constants, methods, and identifiers are uniquely identified and used in code? Throughout this course, we will explore the rules of identifiers, the differences between "puts" and "print" statements, the significance of commands in explaining code, the utilization of variables to store and manipulate data, and the concept of interpolation to insert values into strings.
By the end of this course, you will have a solid understanding of Ruby syntax, enabling you to write clear and effective code. So, are you ready to embark on this exciting journey of exploring Ruby syntax and enhancing your programming skills? Let's get started!
Main Concepts of Ruby Syntax
-
Identifiers: Identifiers in Ruby refer to variable names, constants, and methods. These symbols uniquely identify elements in the code, conveying their meaning and usage. Ruby identifiers are case-sensitive, meaning "andromeda" and "Andromeda" would be considered different identifiers. Rules for identifiers include starting with an alphabet or underscore, allowing numbers but not starting with one, avoiding white spaces within, and not using keywords.
-
Difference between
puts
andprint
: Bothputs
andprint
are used to print strings. The key difference is thatputs
adds a newline automatically after execution, whileprint
does not. This distinction affects how the output is displayed when code is run. -
Commands: Commands in Ruby are executable code written by developers to explain their code, making it easier to understand. There are single-line commands, indicated by a hash symbol before the code, and multi-line commands, enclosed by
begin
andend
statements. -
Variables: Variables in Ruby are used to store and manipulate data. For example, to obtain a string input from a user, the
gets
method can be used along with theputs
statement to display a prompt. This interaction demonstrates how variables can enhance user input functionality in Ruby programs. -
Interpolation: Interpolation allows values to be inserted into strings. By placing variables within
#{}
in a string, their values can be dynamically included. This feature is especially useful for combining strings with variables to generate dynamic content within Ruby programs.
Practical Applications of Ruby Syntax
Step-by-Step Guide:
-
Identifiers:
- Start by creating variables, constants, and methods in Ruby.
- Follow these rules for identifiers:
- Should start with an alphabet or underscore.
- Can include numbers but not start with a number.
- Should not have white spaces.
- Should not be keywords.
Try this out! Create a variable following the identifier rules:
my_variable = "Hello, Ruby!"
-
Difference Between
puts
andprint
Statements:puts
adds a newline automatically after execution.print
does not add a newline.
Interactive demo: Try running these two statements to see the difference:
puts "Hello" print "World"
-
Comments:
- Use single-line comments with
#
. - Use multi-line comments with
=begin
and=end
.
Hands-on exercise: Add comments to your code:
# This is a single-line comment =begin This is a multi-line comment =end
- Use single-line comments with
-
Taking User Input:
- Use the
gets
method to get input from the user. - Display a prompt using
puts
.
Practice it now: Create a program to get user input:
puts "Enter your name:" user_input = gets.chomp puts "Hello, #{user_input}!"
- Use the
-
Interpolation:
- Insert values into strings using interpolation.
- Use
#{}
to include variables within a string.
Test it out: Try interpolating variables into a string:
a = 10 b = 20 puts "The sum of a and b is #{a + b}"
Now grab your favorite code editor and dive into Ruby syntax! Happy coding! 🚀
Test your Knowledge
What character is used to denote comments in Ruby?
What character is used to denote comments in Ruby?
Advanced Insights into Ruby Syntax
When diving deeper into Ruby syntax, it's essential to understand the significance of identifiers, which are crucial for variables, constants, and methods. Identifiers uniquely identify elements in the code and provide meaning and usage. Remember that Ruby identifiers are case-sensitive, distinguishing between identifiers like entropy
and Entropy
.
Rules of Identifiers:
- Should start with an alphabet or underscore.
- Can include numbers, but not start with a number.
- Cannot contain white space.
- Should not be keywords.
Differentiating between puts
and print
can enhance your Ruby skills. While both are used to print a string, puts
automatically adds a newline after execution, creating a separate line for each output. On the other hand, print
outputs text without spaces.
Understanding the use of commands in Ruby is another advanced aspect. Commands are essential executable codes that developers use to explain their code for better comprehension. Remember the distinction between single-line comments (#) and multi-line comments (begin-end) for organizing code readability.
To enhance user interaction, mastering variables and interpolation is key. Variables allow for storing and manipulating data, while interpolation enables inserting values into strings dynamically. These skills are fundamental for creating interactive and dynamic Ruby programs.
Keep exploring Ruby syntax to master its nuances and maximize your programming capabilities.
Happy learning!
Additional Resources for Ruby Syntax
- Ruby Documentation: Official documentation for Ruby programming language.
- Ruby Style Guide: A comprehensive guide on coding style and best practices in Ruby.
- Ruby Cookbook: A collection of practical recipes for Ruby programming.
- Ruby on Rails Guides: Official guides for Ruby on Rails framework, built on the Ruby language.
- Ruby Weekly: A weekly newsletter with updates, tutorials, and news related to Ruby programming.
Explore these resources to enhance your understanding of Ruby syntax and programming. Happy learning! 🚀
Practice
Task: Write a Ruby script that calculates the sum of two numbers provided by the user.
Task: Ensure the code follows Ruby syntax conventions (e.g., proper indentation).