Variables and Data Types
This lesson provides a detailed explanation of data types and variables in Ruby programming. It covers integers, strings, and booleans, along with practical examples and explanations of how to use variables in a Ruby program.
Lets Go!
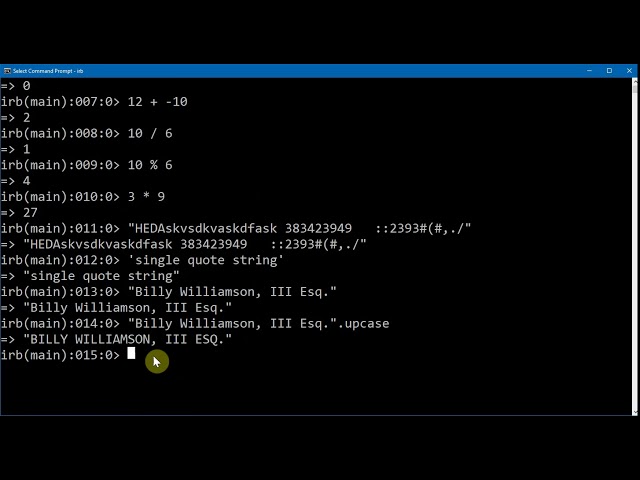
Variables and Data Types
Lesson 6
Learn about Ruby's variable declaration and commonly used data types like integers, floats, strings, arrays, and hashes.
Get Started 🍁Introduction to Ruby Programming: Creating Your Own Pokemon Game
Welcome to "Introduction to Ruby Programming: Creating Your Own Pokemon Game"! In this course, we will embark on a journey to learn the fundamentals of programming in Ruby by creating our very own Pokemon clone.
Have you ever wondered how the inner workings of a Pokemon game are coded? Join us as we dive into the world of Ruby programming to uncover the magic behind creating a digital universe filled with pocket monsters.
Throughout this course, we will cover essential topics such as data types, variables, control flow, and more, all while working towards the goal of developing our Pokemon game from scratch.
Are you ready to unleash your creativity and bring your favorite Pokemon to life through code? Let's embark on this exciting adventure together!
So, are you prepared to catch 'em all in the world of Ruby programming? Let's get started!
Main Concepts of Programming in Ruby
-
Integers:
- Integers are positive numbers, negative numbers, or zero.
- They can be used in different mathematical operations like addition, subtraction, multiplication, and division.
- Ruby handles integer division differently, where dividing two integers results in another integer.
- Remainders of integers can be obtained using the modulus operator.
-
Strings:
- Strings are sequences of characters enclosed in quotes.
- They can contain letters (uppercase and lowercase), numbers, spaces, and punctuation.
- Various methods can be applied to strings, like converting to uppercase using the
upcase
method. - Strings are manipulated and modified based on specific requirements in programming.
-
Booleans:
- Booleans are values that can either be true or false.
- They are used for logical comparisons and evaluations.
- Comparison operators, such as less than, greater than, equal to, and not equal to, return boolean values.
- Booleans are essential for decision-making and control flow in programming.
-
Variables:
- Variables are used to store and manipulate data in a program.
- They are defined by assigning a value to a variable name, following conventions like lowercase letters with underscores.
- Values stored in variables can be of various data types, including integers, strings, booleans, and more.
- Variables allow for dynamic and flexible data handling, enabling changes and updates throughout the program's execution.
Practical Applications of Data Types and Variables in Ruby
Let's apply the concepts of data types and variables discussed in the video to create a simple program related to capturing and managing Pokémon.
Step-by-Step Guide
-
Open the IRB Console:
- Open your command prompt and type
irb
to start the Ruby interpreter.
- Open your command prompt and type
-
Create Variables for Pokémon Data:
- To simulate capturing a Pokémon, create variables for the Pokémon's name, level, and status:
pikachu_name = "Pikachu" pikachu_level = 50 has_fainted = false
- To simulate capturing a Pokémon, create variables for the Pokémon's name, level, and status:
-
Modify Pokémon Data:
- Update variables to reflect changes in the Pokémon's status:
pikachu_name = "Billy Williamson the Third" pikachu_level += 1 has_fainted = true
- Update variables to reflect changes in the Pokémon's status:
-
Utilize Data Types:
- Explore different data types with the variables created:
- String: Represents the Pokémon's name.
- Integer: Stores the Pokémon's level.
- Boolean: Tracks if the Pokémon has fainted.
- Explore different data types with the variables created:
-
Manipulate Data Using Variables:
- Increase the Pokémon's level by one using the
+=
operator:pikachu_level += 1
- Increase the Pokémon's level by one using the
-
Adjust Pokémon Status:
- Change the
has_fainted
variable totrue
if the Pokémon faints during a battle:has_fainted = true
- Change the
-
Experiment Further:
- Feel free to explore additional operations and modifications to enhance the Pokémon experience in your program.
Hands-On Activity
- **Try modifying the Pokémon's level and status using variables in the IRB console.
- **Experiment with different string manipulations for the Pokémon's name.
- **Explore how boolean variables can be toggled based on in-game events.
- **Create new variables and data types to expand the Pokémon program further.
Give it a go and have fun creating your Pokémon world with Ruby! Feel free to share your experience and any challenges faced along the way.
Test your Knowledge
Which of the following is NOT a valid data type in Ruby?
Which of the following is NOT a valid data type in Ruby?
Advanced Insights into Programming with Ruby
In our journey to explore the fundamentals of Ruby programming, we have delved into data types and variables. Now, let's take a deeper look into some advanced aspects that will enrich your understanding and proficiency in Ruby programming.
Ruby Interpreter (IRB)
While many of us are familiar with writing Ruby code in files and executing them from the command line, there's another valuable tool at our disposal - the Interactive Ruby Shell (IRB). IRB allows you to interactively run Ruby code on-the-fly, making it ideal for quick testing or experimentation. By utilizing IRB, you can swiftly test snippets of code, perform calculations, or validate logic, gaining immediate feedback on your code.
Curiosity Question: How can you leverage IRB to explore new Ruby methods or functionalities in real-time?
Integer Math & Operations
When working with integers in Ruby, it's crucial to understand not just basic arithmetic operations but also some fascinating nuances. For instance, Ruby's behavior with integer division might surprise you, as it returns an integer result instead of a decimal fraction. Explore the intricacies of integer division and modulo operator to manipulate numbers creatively in your Ruby programs.
Expert Tip: Utilize integer operations creatively to enhance the logic and efficiency of your Ruby programs. Experiment with different mathematical operations to develop a deeper understanding of integer handling in Ruby.
Manipulating Strings
Strings are a fundamental data type in Ruby, offering a wealth of methods for manipulation and transformation. By exploring methods like upcase
, concat
, reverse
, and more, you can dynamically modify and work with strings to suit your programming needs. Understanding the power of string manipulation opens up a plethora of possibilities for creating dynamic and responsive programs.
Recommendation: Dive deeper into string manipulation methods in Ruby to uncover diverse ways of transforming and utilizing strings in your programs.
Harnessing Variables
Variables play a crucial role in storing and managing data in your Ruby programs. By creating and manipulating variables efficiently, you can maintain state, track values, and facilitate dynamic interactions within your code. Experiment with updating variables, incrementing values, and changing data types to witness the impact on your program's behavior.
By delving into these advanced insights and exploring the nuances of data types, operations, strings, and variables in Ruby, you'll elevate your programming skills and unlock new possibilities for building robust and dynamic applications.
Stay tuned for our upcoming sessions where we will delve into control flow mechanisms and demonstrate how to leverage variables effectively in building complex programs. Let's continue our journey of mastering Ruby programming together!
Additional Resources for Ruby Programming
Interested in expanding your knowledge of Ruby programming after watching this video? Check out these resources to enhance your understanding:
-
Ruby Official Documentation: Dive deeper into the official Ruby documentation to explore more about data types, variables, and other fundamental concepts. Visit Ruby Documentation.
-
Ruby Guides: Explore in-depth tutorials and guides on Ruby programming to further your learning journey. Check out the Ruby Guides website.
-
RubyMonk: Sharpen your Ruby skills with interactive lessons and challenges on RubyMonk. Practice coding and advance your proficiency in Ruby. Visit RubyMonk.
-
Ruby Programming Language Forum: Engage with a community of Ruby programmers, ask questions, and participate in discussions related to Ruby programming on the official Ruby forum.
-
"Learn Ruby the Hard Way" by Zed A. Shaw: Consider grabbing a copy of this popular book to deepen your understanding of Ruby programming concepts through hands-on exercises and projects. Check out Learn Ruby the Hard Way.
Explore these resources to further your exploration of Ruby programming and take your skills to the next level! Happy coding!
Practice
Task: Create a Ruby script to declare and print variables of each data type.
Task: Write a program that combines string interpolation and variables to generate a greeting message.