Advanced Vue.js: Performance, SSR, Testing, and API Integration
In Level 4, you will dive into advanced Vue.js topics, including performance optimization techniques, server-side rendering (SSR) with Nuxt.js, testing with Vue Test Utils, and integrating external APIs. These skills will help you build high-performance, scalable applications, test them effectively, and integrate data from third-party services.
Lets Go!
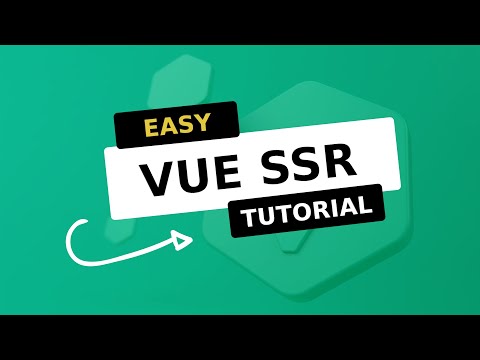
Advanced Vue.js: Performance, SSR, Testing, and API Integration
Lesson 4
Master performance optimization techniques, SSR with Nuxt.js, testing Vue components and applications, and integrating external APIs to build highly performant, scalable, and testable Vue.js applications.
Get Started 🍁Advanced Vue.js: Performance, SSR, Testing, and API Integration
In this level, you'll explore advanced Vue.js features, including performance optimization, server-side rendering with Nuxt.js, testing components with Vue Test Utils, and integrating with external APIs. By the end of this level, you'll be equipped with the skills to build robust, scalable applications with improved performance, advanced SEO capabilities, and reliable testing workflows.
Key Concepts of Advanced Vue.js
-
Performance Optimization:
- Learn how to optimize your Vue.js application for better performance by reducing unnecessary re-renders, lazy-loading components, optimizing the event loop, and using caching strategies.
- Techniques like
v-show
vsv-if
, lazy loading, code splitting, and proper use of computed properties will be covered.
-
Server-Side Rendering (SSR) with Nuxt.js:
- Nuxt.js is a powerful framework for building SSR applications with Vue.js. It provides automatic routing, static site generation, and server-side rendering capabilities out of the box.
- You'll learn how to set up a Nuxt.js project, work with async data fetching, and take advantage of SSR for faster page loads and better SEO.
-
Vue Test Utils and Testing:
- Vue Test Utils is the official unit testing library for Vue.js applications. You'll learn how to write unit tests for your components, simulate user interactions, and test Vuex actions and mutations.
- We'll also explore end-to-end testing with tools like Cypress.
-
API Integration:
- Learn how to integrate external APIs into your Vue.js application using Axios or Fetch. You will also work with third-party services such as authentication, payment systems, and data fetching.
Practical Applications
- Performance Optimization: Optimize an existing Vue.js app by implementing code splitting, lazy loading of components, and memoization.
- Nuxt.js Setup: Create a Nuxt.js application with SSR, implement async data fetching, and deploy it as a static site.
- Testing with Vue Test Utils: Write unit tests for a Vue component, mock API calls, and test Vuex stores.
- API Integration: Integrate a third-party API (e.g., Google Maps, Stripe) into your Vue.js application and display the data dynamically.
Test your Knowledge
What is the primary advantage of Server-Side Rendering (SSR) in Vue.js?
What is the primary advantage of Server-Side Rendering (SSR) in Vue.js?
Advanced Insights
-
Caching Strategies: Learn about different caching techniques like memoization, localStorage, and sessionStorage to improve performance.
-
Static Site Generation (SSG): Explore how Nuxt.js can generate static pages at build time, which improves load times and SEO.
-
Code Splitting: Break your app into smaller chunks to load only the necessary JavaScript for each page, reducing the initial loading time.
-
End-to-End Testing: Explore end-to-end testing with Cypress to ensure your application works as expected from the user’s perspective.
-
Error Handling in APIs: Learn how to handle errors when making API calls, including retries, error boundaries, and user notifications.
Additional Resources for Advanced Vue.js
- Nuxt.js Official Documentation: Nuxt.js Docs
- Vue Performance Optimization: Vue Performance Best Practices
- Vue Test Utils: Vue Test Utils Docs
- Cypress for End-to-End Testing: Cypress Documentation
- Axios Documentation: Axios Docs
Practice
Task: Optimize a Vue.js application by implementing lazy loading for its components and using memoization for computed properties.
Task: Set up a Nuxt.js project with SSR and implement dynamic data fetching using the asyncData
method.
Task: Write unit tests for a Vue component using Vue Test Utils and mock an API call.
Task: Integrate a third-party API (like a weather API) into a Vue component and display the data dynamically.
Task: Set up Cypress for end-to-end testing of your Vue.js app and write tests for user interactions.