Mastering Vue.js:Performance Optimization, and Modular Architecture
In this advanced course, you will dive deep into Vue.js testing, performance optimization, and modular application architecture. Learn how to write effective unit and integration tests, optimize your Vue applications for speed, and structure your app in a modular way to improve maintainability and scalability.
Lets Go!
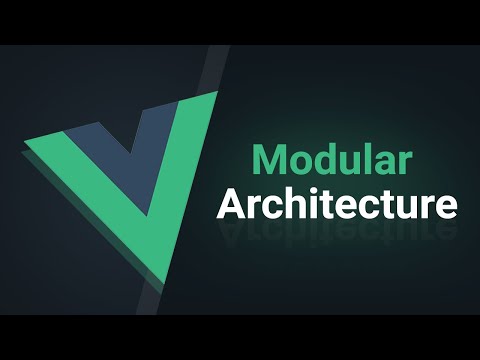
Mastering Vue.js:Performance Optimization, and Modular Architecture
Lesson 5
Master advanced techniques in Vue.js, including testing, performance optimization, and creating modular architectures for large-scale applications.
Get Started 🍁Mastering Vue.js:Performance Optimization, and Modular Architecture
This course focuses on advanced concepts in Vue.js to help you create high-performance applications that are easier to maintain and scale. You will master testing techniques, including unit and integration testing with tools like Jest and Vue Test Utils, optimize performance with advanced techniques, and build modular architecture with Vue 3 features like Composition API, Vuex, and Vue Router.
Key Concepts in Mastering Vue.js
-
Unit and Integration Testing:
- Learn how to write unit and integration tests for Vue components using Jest and Vue Test Utils.
- Understand the difference between unit tests (isolated testing) and integration tests (testing interactions between components).
-
Performance Optimization:
- Explore strategies for improving the performance of Vue applications, such as lazy loading components, optimizing reactivity, and caching.
- Learn how to use tools like Vue DevTools and Lighthouse to analyze performance and optimize your app.
-
Modular Architecture:
- Learn how to create a modular Vue application using Vue Router, Vuex, and Composition API to organize your app into smaller, reusable pieces.
- Understand how to use dynamic imports and code-splitting to optimize loading times and improve app scalability.
-
State Management with Vuex:
- Dive deeper into Vuex for managing state in larger applications. Learn advanced techniques for state mutation, actions, and getters.
Practical Applications
- Write Unit Tests: Create unit tests for your Vue components to ensure they behave as expected and handle edge cases.
- Performance Tuning: Use Vue DevTools and Chrome DevTools to analyze your app’s performance and make improvements.
- Modular Vue App: Build a modular Vue application using Composition API and Vue Router, and split the app into smaller, reusable components.
- State Management: Set up a Vuex store with namespaced modules for managing different aspects of your app's state, such as authentication and user settings.
Test your Knowledge
Which testing library is commonly used with Vue.js for unit and integration testing?
Which testing library is commonly used with Vue.js for unit and integration testing?
Advanced Insights for Vue.js Development
- Code Splitting and Lazy Loading: Implement lazy loading for routes and components to reduce the initial bundle size and improve load times.
- Advanced Vuex: Explore Vuex plugins and modules for advanced state management in large applications.
- Reactivity System: Understand Vue 3's reactivity system at a deeper level, and optimize performance by minimizing unnecessary re-renders.
- Server-Side Rendering (SSR): Learn the basics of Vue SSR for rendering applications on the server to improve SEO and load times.
Additional Resources for Advanced Vue.js
- Vue Test Utils Documentation: Vue Test Utils
- Jest Testing Framework: Jest Docs
- Vue 3 Performance Optimization Guide: Vue Performance
- Vue DevTools: Vue DevTools
Practice
Task: Write a unit test for a Vue component using Vue Test Utils and Jest.
Task: Use Vue DevTools to analyze your app’s performance and apply optimizations based on the results.
Task: Refactor your app to implement lazy loading for routes using Vue Router.
Task: Create a modular Vue app using Vuex and the Composition API, and organize your app's state into namespaced modules.
Task: Implement code splitting in your Vue app and measure the performance improvement.