Vue.js Advanced Application Design, Testing, and Deployment
In Level 3, we will focus on designing large-scale, performant Vue.js applications. This includes optimizing performance with techniques like code splitting and caching, writing unit and integration tests for Vue components, and deploying Vue.js applications with CI/CD pipelines. You'll also explore best practices for maintaining and scaling Vue apps in production.
Lets Go!
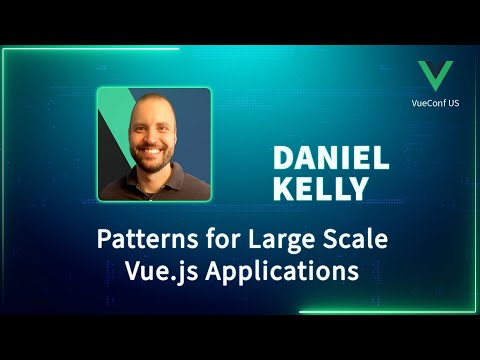
Vue.js Advanced Application Design, Testing, and Deployment
Lesson 3
Learn to optimize Vue.js applications for performance, write unit and integration tests for reliability, and automate deployment through CI/CD pipelines.
Get Started 🍁Vue.js Advanced Application Design, Testing, and Deployment
This level will take your Vue.js skills to the next level by focusing on advanced application design, performance optimization, testing, and deployment. You’ll learn how to optimize your Vue apps for scalability and speed, write tests to ensure reliability, and use CI/CD pipelines for smooth deployments.
Key Concepts of Advanced Vue.js Development
-
Code Splitting:
- Code splitting allows you to split your JavaScript bundle into smaller chunks, improving app load time by loading only necessary code.
- Vue supports dynamic imports and lazy loading for code splitting.
-
Performance Optimization:
- Use techniques like lazy loading, async components, and virtual scrolling to optimize large applications.
- Use Vue’s built-in performance tools such as
v-once
,v-memo
, andv-bind
to reduce unnecessary re-renders.
-
Unit Testing:
- Writing unit tests ensures that each component behaves as expected.
- Tools like Vue Test Utils and Jest can help test Vue components in isolation.
-
Integration Testing:
- Integration tests check how various parts of your application work together, ensuring that state management, routes, and API calls function correctly.
-
Continuous Integration / Continuous Deployment (CI/CD):
- Automate the testing, building, and deployment of Vue.js applications with CI/CD pipelines using tools like GitHub Actions, GitLab CI, or CircleCI.
-
Vue CLI and Vue.config:
- The Vue CLI provides tools for bundling and optimizing your application, and Vue.config allows for custom configuration to tailor your project to specific needs.
Practical Applications
- Implement Code Splitting: Refactor a large Vue app to implement dynamic imports for better performance.
- Optimize Performance: Use
v-once
andv-bind
to optimize rendering in components with heavy DOM updates. - Write Unit Tests: Write unit tests for a simple component using Vue Test Utils and Jest.
- Integration Testing: Write tests that verify interactions between components, like making an API call and updating the state.
- Set Up CI/CD: Create a CI/CD pipeline using GitHub Actions to automatically run tests and deploy your application to production.
Test your Knowledge
What is code splitting used for in Vue.js?
What is code splitting used for in Vue.js?
Advanced Insights
-
Server-Side Rendering (SSR): Vue can be used for server-side rendering to improve SEO and initial load time. Learn how to set up SSR with Vue and Nuxt.js.
-
Vue Composition API for Large-Scale Apps: While the Composition API offers flexibility, it's essential to structure your logic well in large applications to avoid messy code.
-
Error Boundaries: Learn how to handle errors globally in Vue apps using error boundaries to improve user experience.
-
State Management Best Practices: Learn how to organize your Vuex store with modules and avoid pitfalls of overusing state management in small apps.
-
Lazy Loading Routes and Components: Explore how to optimize the performance of routes and components using Vue Router’s lazy loading feature, ensuring that large apps only load the necessary code at runtime.
Additional Resources for Advanced Vue.js
- Vue.js Official Performance Guide: Vue Performance
- Vue Test Utils Documentation: Vue Test Utils
- Continuous Deployment with GitHub Actions: GitHub Actions
- Nuxt.js for SSR: Nuxt.js
- Vue.js SSR Tutorial: Vue SSR Guide
Practice
Task: Set up code splitting in a Vue.js project using dynamic imports for routes and components.
Task: Write a unit test for a component using Vue Test Utils and Jest to ensure it behaves correctly.
Task: Create an integration test to verify that components interact properly, such as an API call updating the component’s state.
Task: Set up a CI/CD pipeline using GitHub Actions to automate tests and deployments for your Vue app.
Task: Optimize a Vue app by using lazy loading and the v-once
directive to reduce unnecessary re-renders.