Vue.js State Management, Routing, and Form Handling
In Level 2, we will dive deeper into more advanced concepts of Vue.js, such as managing state with Vuex, handling routing with Vue Router, and working with forms. You'll learn how to manage complex state in your applications, implement dynamic routing, and validate forms efficiently. By the end of this level, you will have the skills needed to build more interactive and dynamic Vue.js applications.
Lets Go!
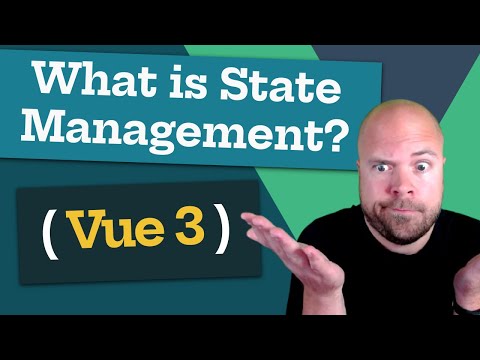
Vue.js State Management, Routing, and Form Handling
Lesson 2
Learn to manage state in complex Vue.js applications with Vuex, handle routing with Vue Router, and build forms with validation to improve user interaction and experience.
Get Started 🍁Vue.js State Management, Routing, and Form Handling
In this level, you will learn how to use Vuex for state management in larger applications, set up routing with Vue Router, and handle forms including validation. These skills are essential when building interactive, scalable applications with Vue.js.
Key Concepts of State Management, Routing, and Forms
-
Vuex (State Management):
- Vuex is a state management library for Vue.js applications. It provides a centralized store for all the components in an app, making it easier to manage state and avoid prop-drilling.
- Key concepts of Vuex include
state
,mutations
,actions
, andgetters
.
-
Vue Router (Routing):
- Vue Router is the official router for Vue.js, enabling navigation between different views or pages in single-page applications.
- You’ll learn about dynamic routing, nested routes, route guards, and lazy loading of components.
-
Form Handling:
- Handling forms in Vue.js involves working with
v-model
for two-way data binding, form validation, and submitting data to an API. - You’ll also explore form validation techniques using custom validators or third-party libraries like VeeValidate.
- Handling forms in Vue.js involves working with
Practical Applications
- Vuex Store Setup: Create a Vuex store to manage application-wide state and implement actions to fetch and update data.
- Vue Router Setup: Set up Vue Router with multiple routes, including dynamic routes for user profiles or product details.
- Form Handling: Build a form with validation to collect user information and submit it to an API.
- Nested Routes: Set up nested routes for a complex layout, such as an admin dashboard with different sections for users and settings.
Test your Knowledge
What is the main purpose of Vuex in a Vue.js application?
What is the main purpose of Vuex in a Vue.js application?
Advanced Insights
-
Modular Vuex: Learn how to break the Vuex store into modules to organize the state better, especially for larger applications.
-
Vue Router Navigation Guards: Explore route guards to prevent unauthorized users from accessing certain routes or pages.
-
Form Validation Libraries: While Vue offers basic form validation, explore third-party libraries like VeeValidate or Vuelidate for more advanced validation needs.
-
Lazy Loading with Vue Router: Optimize app performance by lazily loading components when they are needed rather than loading all components at once.
Additional Resources for State Management, Routing, and Forms
- Vuex Official Documentation: Vuex Docs
- Vue Router Official Documentation: Vue Router Docs
- VeeValidate for Form Validation: VeeValidate
- Vuex and Vue Router Tutorial: Vuex and Vue Router Guide
Practice
Task: Create a Vuex store to manage a list of products in your app and implement actions to add and remove items from the cart.
Task: Set up Vue Router with nested routes for a user profile and account settings page.
Task: Build a login form with validation that submits the data to a mock API.
Task: Use v-model
to bind input fields in a form and display the entered data dynamically.
Task: Add a route guard in Vue Router to ensure only authenticated users can access the dashboard route.