Arrays and Strings in C++
Arrays and strings are fundamental data structures in C++ used to store collections of elements. Arrays store a fixed-size sequence of elements of the same type, while strings are a sequence of characters. Both are essential for handling and manipulating groups of data efficiently in C++.
Lets Go!
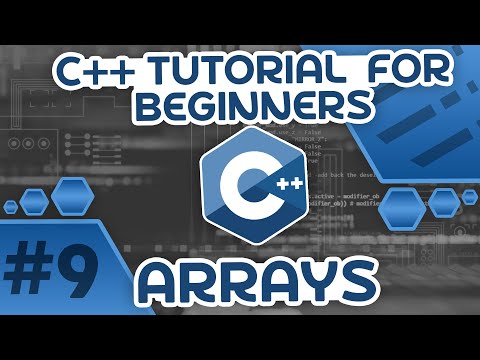
Arrays and Strings in C++
Level 4
Arrays and strings are fundamental data structures in C++ used to store collections of elements. Arrays store a fixed-size sequence of elements of the same type, while strings are a sequence of characters. Both are essential for handling and manipulating groups of data efficiently in C++.
Get Started 🍁Arrays and Strings in C++
Arrays and strings are fundamental data structures in C++ used to store collections of elements. Arrays store a fixed-size sequence of elements of the same type, while strings are a sequence of characters. Both are essential for handling and manipulating groups of data efficiently in C++.
Introduction to Arrays
Welcome to "Introduction to Arrays"! In this course, you will delve into the fundamentals of arrays, a crucial concept in programming.
An array is a data structure that allows you to store multiple values of the same data type in a single variable. Unlike lists, arrays require a predefined size and maintain elements of the same type.
Have you ever wondered how to efficiently store and access multiple elements in your programs? Arrays provide a solution to this problem by allowing you to store and manipulate data in a structured manner.
Throughout this course, you will learn the basics of arrays, how to declare and initialize them, access individual elements, and determine the size of an array. By the end of this course, you will have a solid understanding of how arrays work and how to leverage them in your own coding projects.
Are you ready to dive into the world of arrays and enhance your programming skills? Let's get started!
Main Concepts of Arrays
-
Definition of an Array: An array is a data structure that allows us to store multiple values of the same data type in the same variable or memory location.
-
Difference Between List and Array: It is important to note that a list is not the same as an array. While they are similar, arrays have some key differences, such as the fixed number of elements.
-
Array Structure: In C++, arrays are denoted by squiggly brackets. Elements within the array must all be the same data type, such as int, float, bool, or string.
-
Creating an Array: When declaring an array, it is essential to specify the data type of the elements it will store, followed by the array name and size in squared brackets.
-
Accessing Elements in an Array: Elements in an array are accessed using indices, which start at 0. For example, array[0] accesses the first element in the array.
-
Changing Elements in an Array: Elements in an array can be modified by assigning a new value to a specific index, allowing for dynamic changes to the array's content.
-
Initializing an Array: Array elements can be defined at the time of declaration or left empty, with values assigned later. Not initializing elements can lead to unpredictable values being stored.
-
Getting the Size of an Array: In C++, determining the size of an array involves using the
sizeof
function to calculate the number of bytes the array occupies and dividing it by the size of one element to find the total number of elements.
Practical Applications of Arrays
Arrays are essential data structures that allow you to store multiple values of the same type in a single variable. Here are some practical tips on working with arrays in C++:
Step 1: Defining an Array
To define an array in C++, follow these steps:
- Choose the data type the array will store (e.g., int, char, string).
- Declare the array with the desired data type and size using square brackets after the variable name.
int arr[5]; // Defines an integer array with 5 elements
Step 2: Initializing Array Elements
You can either provide initial values while defining the array or assign values later. Here's an example of initializing an array with elements:
int arr[] = {1, 2, 3, 4, 5}; // Initializes array with values 1, 2, 3, 4, 5
Step 3: Accessing Elements in the Array
Arrays in C++ start indexing at 0. To access elements in an array, use the index within square brackets. For example, to access the third element in the array arr
, use arr[2]
.
int thirdElement = arr[2]; // Access the third element (index 2) in the array
Step 4: Changing Array Elements
You can modify elements in an array by assigning new values to specific indices. For instance, to change the first element in the array arr
to 10:
arr[0] = 10; // Changes the first element to 10
Step 5: Getting the Size of the Array
While C++ does not have a straightforward method to determine the array's size, you can calculate it by dividing the total size of the array by the size of one element:
int size = sizeof(arr) / sizeof(arr[0]); // Calculates the number of elements in the array
Try out these steps in your own C++ program to practice working with arrays effectively! Let us know your experience in the comments below.
Test your Knowledge
Which of the following correctly declares an array in C++?
Which of the following correctly declares an array in C++?
Advanced Insights into Arrays
Arrays are essential data structures in programming, allowing for the storage of multiple values of the same data type in a structured manner. Here are some advanced insights into arrays to further enhance your understanding:
-
Understanding Array Initialization: When initializing an array, it's crucial to define the size of the array upfront. Remember, the size of an array cannot be changed after initialization. If you need to modify the size, you'll have to create a new array and copy the elements over.
-
Accessing Array Elements: Arrays in C++ are zero-indexed, meaning the first element is at index 0, the second element is at index 1, and so on. Ensure you grasp the concept of indices and how they relate to accessing specific elements within an array.
-
Changing Array Elements: It's possible to modify the values of individual elements within an array by accessing them through their respective indices. Understanding how to manipulate array elements is crucial for working efficiently with arrays.
-
Size of Arrays: Unlike some other programming languages, determining the size of an array in C++ isn't as straightforward. To find the number of elements in an array, you can utilize the
sizeof
operator to calculate the total bytes occupied by the array and then divide it by the size of a single element.
A question to ponder: How can you dynamically allocate memory for arrays in C++ to overcome the limitation of static array sizes?
Exploring these advanced insights will solidify your knowledge of arrays and empower you to leverage them effectively in your programming endeavors. Next time you work with arrays, be prepared to tackle more complex scenarios with confidence.
Additional Resources for Arrays
- Article: Arrays in C++
- Tutorial: Understanding Arrays in C++
- Video: Arrays Explained in C++
Explore these resources to enhance your understanding of arrays in C++. Happy learning!
Practice
Task
Task: Reverse an array of integers.
Task: Write a function that concatenates two strings.
Task: Find the largest number in an array of integers.
Task: Implement a function that checks if a string is a palindrome.
Task: Convert a string to uppercase.
Task: Implement a function to sort an array of strings in lexicographical order.
Task: Create a function to compare two strings.
Task: Write a program to count the number of occurrences of a character in a string.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
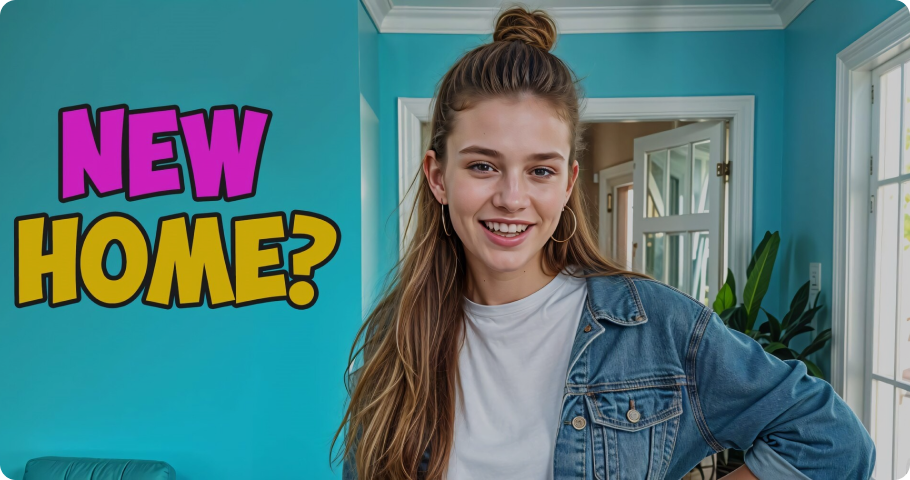
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.