Functions in C++
Functions in C++ allow you to group code into reusable blocks, which can improve code readability, maintainability, and modularity. Functions are an essential part of structured programming, allowing you to divide tasks into smaller, manageable units.
Lets Go!
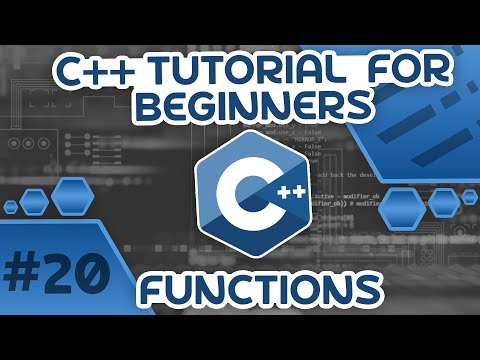
Functions in C++
Level 5
Functions in C++ allow you to group code into reusable blocks, which can improve code readability, maintainability, and modularity. Functions are an essential part of structured programming, allowing you to divide tasks into smaller, manageable units.
Get Started 🍁Functions in C++
Functions in C++ allow you to group code into reusable blocks, which can improve code readability, maintainability, and modularity. Functions are an essential part of structured programming, allowing you to divide tasks into smaller, manageable units.
Introduction to Functions in C++
Welcome to the course "Introduction to Functions in C++"! Functions are an essential building block in programming, allowing us to create reusable blocks of code that perform specific tasks. In this course, we will dive deep into the world of functions and explore how they can streamline our code, enhance readability, and improve efficiency.
Functions in C++ can take in parameters, perform operations, and return values. They can be simple, like adding two numbers, or complex, like solving advanced equations. By mastering functions, you'll be able to create modular, organized, and efficient code that can be reused in various parts of your program.
Throughout this course, we will cover topics such as defining functions, passing parameters, using default parameters, and understanding pass by value versus pass by reference. We will also explore advanced concepts like pointers and object-oriented programming.
Are you ready to unlock the power of functions in C++ and enhance your programming skills? Let's dive in and discover the endless possibilities of functions!
Main Concepts of Functions in C++
-
Functions: Functions are reusable blocks of code that can take in parameters or arguments, perform operations, and return values. They help in organizing and separating logical operations in a program.
-
Parameters and Arguments: Parameters are the variables in the function definition, while arguments are the actual values passed to the function when it is called. Parameters must match the type and number of arguments provided.
-
Return Types: Functions can have return types specifying the type of value they will return. This helps in indicating what the function will output when executed.
-
Default Parameters: Functions can have default parameters, allowing certain parameters to be optional. If a value is not provided, the default value is used.
-
Pass by Value vs. Pass by Reference:
- Pass by Value: When passing arguments by value, copies of the original values are passed to the function. Any changes made to the parameters within the function do not affect the original values.
- Pass by Reference: When passing arguments by reference using
&
, the function receives the memory address of the original values. Changes made to the parameters within the function directly impact the original values.
-
Pointers in Functions: Functions can also accept pointers, allowing flexibility in manipulating data using memory addresses.
-
Function Naming and Organization: Functions should be named descriptively to indicate their purpose and should ideally perform a single task efficiently. Properly naming and organizing functions can improve code readability and maintainability.
-
Multiple Return Statements: Functions can have multiple return statements, but the first encountered return statement will terminate the function execution.
-
Flexibility in Return Types: Functions are flexible in terms of return types and can return various data types such as pairs, vectors, arrays, etc.
By understanding these main concepts of functions in C++, learners can effectively utilize functions to structure their code, enhance reusability, and optimize program logic. Functions play a crucial role in programming and are essential for building efficient and organized applications.
Practical Applications of Functions
Functions in C++ are a powerful tool that allows for the creation of reusable blocks of code to perform specific tasks. Here are some practical applications of functions that you can try out:
Step-by-Step Guide
1. Create a Basic Function
- Define a function with a return type and parameters.
- Write the body of the function to perform a task, such as adding two numbers.
- Call the function in the
main
function and pass the required arguments. - Run the code to see the function in action.
#include <iostream> int add(int x, int y) { return x + y; } int main() { int result = add(2, 3); std::cout << result << std::endl; return 0; }
2. Use Default Parameters
- Implement a function with default parameters to make certain inputs optional.
- Call the function with and without providing the optional parameter.
- Observe the behavior of the function based on the arguments passed.
3. Pass by Reference vs. Pass by Value
- Create a function to swap the values of two variables using pass by reference.
- Compare the results of passing variables by value, reference, and pointers.
- Understand the difference between modifying variables within a function using references or pointers.
Example Code for Swapping Variables Using Pass by Reference
#include <iostream> void swap(int& x, int& y) { int temp = x; x = y; y = temp; } int main() { int a = 2, b = 4; std::cout << "Before Swap: a = " << a << ", b = " << b << std::endl; swap(a, b); std::cout << "After Swap: a = " << a << ", b = " << b << std::endl; return 0; }
By following these steps and examples, you can gain a better understanding of how functions work and how they can be implemented in practical scenarios within your C++ programs. Have fun experimenting with different function implementations and exploring the capabilities of functions in your coding projects!
Test your Knowledge
Which of the following is a correct way to define a function in C++?
Which of the following is a correct way to define a function in C++?
Advanced Insights into Functions
Functions are a fundamental concept in programming as they allow for reusable blocks of code that can perform specific tasks efficiently. In this section, we will explore some advanced aspects and deeper insights into functions to enhance your understanding.
Default Parameters
When defining a function, you can set default values for parameters. This means that the function can be called without passing specific arguments, as it will use the default values instead. Default parameters provide flexibility and make functions more versatile. You can utilize default parameters to handle different scenarios and simplify function calls.
Pass by Reference vs. Pass by Value
Understanding the difference between pass by reference and pass by value is crucial when working with functions. When passing arguments by value, a copy of the value is created, ensuring that the original value remains unchanged. On the other hand, passing arguments by reference allows the function to modify the original value directly. By using references or pointers, you can alter the original values passed into the function, enabling you to perform operations directly on them.
Best Practices for Functions
- Functions should ideally do one thing very well, focusing on a specific task or operation.
- Organize your code by creating functions that encapsulate related logic, making your code more readable and maintainable.
- Consider utilizing functions within other functions to modularize your code and improve code reuse.
- Strive to use meaningful function names that describe their purpose for clarity and understanding.
Curiosity Question: Why is it important to distinguish between pass by reference and pass by value when working with functions? How can understanding this distinction improve your programming practices?
Exploring the advanced aspects of functions can significantly enhance your programming skills and efficiency. By mastering the intricacies of functions, you can write more robust and scalable code, ultimately becoming a proficient programmer.
Additional Resources for Functions in C++
References:
Articles:
Video Tutorials:
- C++ Functions Explained - C++ Programming Tutorial for Beginners
- Pointers and Passing by Reference in C++
Explore these resources to deepen your understanding of functions in C++ and enhance your programming skills!
Practice
Task
Task: Write a function to find the maximum of two numbers using a function template.
Task: Implement a function that reverses a string using a recursive approach.
Task: Create a function that swaps two numbers using pointers.
Task: Write a program that calculates the factorial of a number using recursion.
Task: Define a function that takes a number as input and returns whether it's prime or not.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
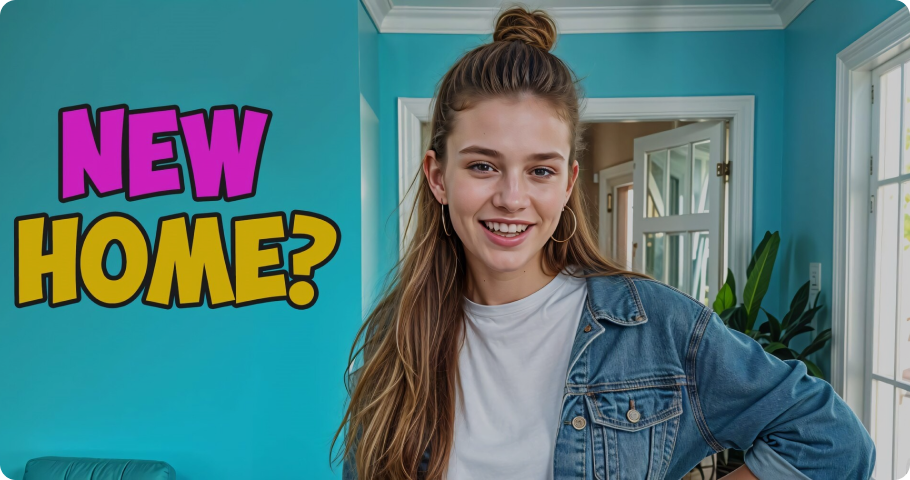
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.