Pointers and Memory Management
Pointers and memory management are critical concepts in C++ that enable developers to directly interact with memory. Mastery of pointers allows for more efficient programs, the use of dynamic memory, and precise control over data. Proper memory management ensures that applications do not have memory leaks and are optimized for performance.
Lets Go!
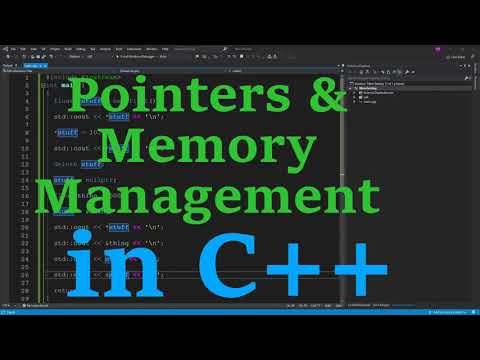
Pointers and Memory Management
Level 6
Pointers and memory management are critical concepts in C++ that enable developers to directly interact with memory. Mastery of pointers allows for more efficient programs, the use of dynamic memory, and precise control over data. Proper memory management ensures that applications do not have memory leaks and are optimized for performance.
Get Started 🍁Pointers and Memory Management
Pointers and memory management are critical concepts in C++ that enable developers to directly interact with memory. Mastery of pointers allows for more efficient programs, the use of dynamic memory, and precise control over data. Proper memory management ensures that applications do not have memory leaks and are optimized for performance.
Introduction to Pointers and Memory Management with C++
Welcome to the "Introduction to Pointers and Memory Management with C++" course! In this course, you will delve into the world of pointers and memory management in C++. We will explore how pointers function as a small bit of memory that points to the location of an object in memory, thus allowing for efficient manipulation and access to data.
Before we dive into advanced topics, we will cover the basics of declaring pointers, initializing memory, dereferencing pointers to access data, and the fundamental operations of new and delete in memory management. Throughout the course, you will gain a comprehensive understanding of how to effectively manage memory allocation, prevent memory leaks, and debug common errors related to memory management.
Are you ready to uncover the power of pointers and enhance your C++ programming skills? Let's embark on this learning journey together!
Curiosity Question: Have you ever wondered how pointers optimize memory usage and data access in C++ programming?
Don't miss out on the upcoming lessons where we will explore shared pointers, unique pointers, and further advanced topics in pointers and memory management. Get ready to elevate your coding proficiency with Code Tech Tutorials!
Let's begin our exploration of pointers and memory management with C++! 🚀🖥️
Main Concepts of Pointers and Memory Management with C++
-
Pointers in C++: Pointers are small bits of memory that indicate the start of an object in memory. They are more efficient than passing around actual objects because they only store the memory address of the object.
-
Initialization of Pointers: Initializing a pointer with
new
allocates memory for the object it points to and assigns it a default value. Without initialization, a pointer only holds a memory address. -
De-referencing Pointers: De-referencing a pointer using
*
allows access to the data it points to. This is essential for reading or modifying the value of the object pointed to. -
Memory Deallocation: Calling
delete
on a pointer releases the memory occupied by the object pointed to. Failing to delete memory can lead to memory leaks, where unused memory remains allocated. -
Scope and Pointers: Pointers must be managed within appropriate scopes. If a pointer goes out of scope without deallocating memory, it can result in dangling pointers or bad references.
-
Error Handling: Errors like
bad_alloc
can occur when memory allocation fails. Using try-catch blocks can help in catching and handling such exceptions gracefully. -
Overloading
new
anddelete
Operators: Redefining these operators allows programmers to customize memory allocation and deallocation. It enables the implementation of custom memory management strategies and error logging. -
Debugging Techniques: Implementing logging mechanisms for memory operations helps in tracking memory usage, identifying memory leaks, and optimizing memory management in complex programs.
-
Shared Pointers and Unique Pointers: Shared pointers and unique pointers are advanced concepts in C++ for managing memory by enabling shared ownership or exclusive ownership of dynamically allocated objects. These will be covered in the next episode for further exploration.
Practical Applications of Pointers and Memory Management
Welcome to the practical applications section! Let's dive into some hands-on coding with pointers and memory management in C++ based on the concepts discussed in the video. Follow these steps to experiment with pointers and memory management techniques:
Step 1: Initializing a Pointer
// Initialize a pointer to a float called 'stuff' float* stuff = new float;
In this step, you are creating a pointer to a float and assigning memory space for it using the new
keyword.
Step 2: Dereferencing the Pointer
// Assign a value to the pointer & dereference to view the value *stuff = 10.0; std::cout << "Value of 'stuff': " << *stuff << std::endl;
By assigning a value to the pointer and dereferencing it, you can access and manipulate the data pointed to by the pointer.
Step 3: Managing Memory Allocation
// Delete the memory allocated for the pointer delete stuff; stuff = nullptr;
When you no longer need the memory allocated for the pointer, remember to delete it and set the pointer to nullptr
to prevent memory leaks.
Step 4: Using References with Pointers
// Declare an object and create a reference using a pointer float thing = 500.0; float& stuff_ref = thing;
You can create references to objects using pointers, helping you manage memory and access values efficiently.
Step 5: Error Handling for Allocation
try { float* endless_leak = nullptr; while (true) { endless_leak = new float; // Delete the previous memory if the allocation was successful delete endless_leak; } } catch(std::bad_alloc& e) { std::cout << "Caught exception: " << e.what() << std::endl; }
Simulating an endless memory allocation loop to demonstrate catching a bad_alloc
exception for memory allocation failure.
Step 6: Custom Memory Management
// Redefine new and delete for custom memory management #define DEBUG 1 #ifdef DEBUG #define new DEBUG_NEW #endif void* operator new(size_t size) { // Custom memory allocation tracking } void operator delete(void* pointer) { // Custom memory deallocation with logging }
Overloading new
and delete
operators for custom memory management and logging purposes.
Explore and Experiment Further
Feel free to modify the provided code snippets and experiment with different scenarios to deepen your understanding of pointers and memory management in C++. Stay tuned for more advanced topics like shared and unique pointers in the upcoming episodes!
Now, it's your turn to try out these steps in your preferred C++ development environment. Have fun coding! 🚀
Stay tuned for more exciting coding tutorials from Matt at Code Tech Tutorials. Like the video, share it with fellow programmers, and consider supporting the content by becoming a patron. Happy coding! 🖥️✨🔧
Test your Knowledge
What does a pointer in C++ store?
What does a pointer in C++ store?
Advanced Insights into Pointers and Memory Management with C++
In the realm of C++, mastering pointers and memory management can unlock a world of efficiency and control. Let's delve into some advanced concepts to further enhance your understanding of this critical topic.
Dynamic Memory Allocation and De-allocation
When dealing with pointers, it's essential to understand memory allocation and de-allocation. Remember, new
allocates memory for an object, while delete
frees up that memory. However, failing to delete dynamically allocated memory can lead to memory leaks, causing unused memory to accumulate, potentially leading to system crashes.
Tip: Always delete dynamically allocated memory before a pointer goes out of scope or assign it to nullptr
to avoid memory leaks.
Managing References
References in C++ provide an alternative way to work with memory and objects. When using references, keep in mind that the referenced object must exist as long as the reference remains in use. Otherwise, accessing a dangling reference can lead to runtime errors and unpredictable behavior.
Question: How can you ensure the validity of references, especially when dealing with objects going out of scope?
Handling Errors in Memory Allocation
During memory allocation with new
, it's crucial to handle potential errors, such as bad_alloc
exceptions when running out of memory. Utilizing try-catch blocks can help gracefully manage such exceptions, preventing crashes and enabling smoother program execution.
Recommendation: Wrap memory allocation operations in try-catch blocks to handle potential bad_alloc
exceptions effectively.
Overloading new
and delete
Advanced memory management techniques involve overloading the new
and delete
operators to customize memory allocation behavior. By defining custom new
and delete
functions, you can implement logging, error checking, and other memory management strategies tailored to your specific needs.
Curiosity: How can you design a sophisticated memory manager using custom new
and delete
operators to optimize memory usage in a large-scale C++ application?
By mastering these advanced insights into pointers and memory management in C++, you can elevate your programming skills and create more robust and efficient applications. Stay tuned for upcoming tutorials on shared pointers and unique pointers to deepen your knowledge further. Happy coding!
This section offers a comprehensive overview of advanced concepts in pointers and memory management, providing practical tips and insights to enhance understanding and proficiency in C++. It aims to inspire learners to explore further and deepen their expertise in this fundamental aspect of programming.
Additional Resources for Pointers and Memory Management in C++
- Article: A Comprehensive Guide to Pointers in C++
- Book: "Effective Modern C++" by Scott Meyers
- Video Tutorial: Memory Management in C++
- Online Course: C++ Memory Management Fundamentals
Explore these resources to deepen your understanding of pointers and memory management in C++. Happy learning!
Practice
Task
Task: Write a program that uses pointers to swap two integers.
Task: Create a program that dynamically allocates an array, fills it with values, and frees the memory.
Task: Implement a linked list using pointers.
Task: Write a program to demonstrate the use of smart pointers in C++.
Task: Use pointers to implement a function that reverses a string.
Task: Handle memory allocation failures using exception handling techniques.
Task: Create a dynamic array of strings and display them using pointer arithmetic.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
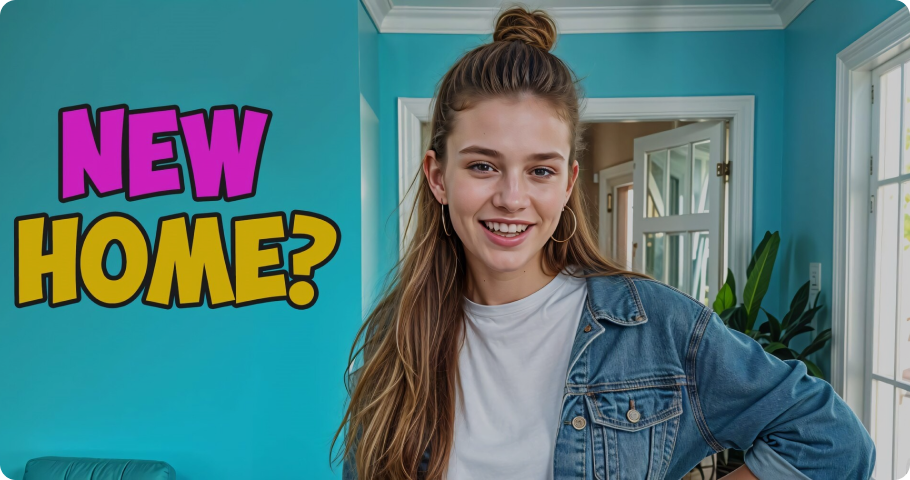
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.