File I/O in C++
File Input/Output (I/O) in C++ allows you to read from and write to files, enabling persistent data storage beyond the runtime of a program. C++ provides an easy-to-use file stream library, allowing you to work with files in both text and binary formats. Mastering file I/O is essential for creating applications that can save and load data efficiently.
Lets Go!
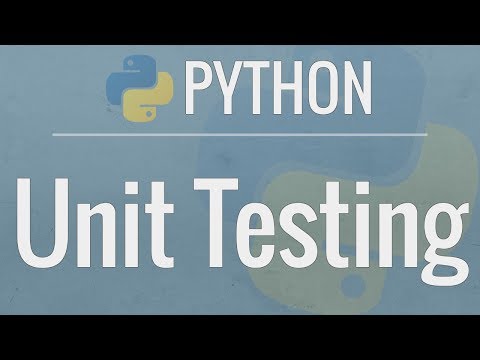
File I/O in C++
Level 9
File Input/Output (I/O) in C++ allows you to read from and write to files, enabling persistent data storage beyond the runtime of a program. C++ provides an easy-to-use file stream library, allowing you to work with files in both text and binary formats. Mastering file I/O is essential for creating applications that can save and load data efficiently.
Get Started 🍁File I/O in C++
File Input/Output (I/O) in C++ allows you to read from and write to files, enabling persistent data storage beyond the runtime of a program. C++ provides an easy-to-use file stream library, allowing you to work with files in both text and binary formats. Mastering file I/O is essential for creating applications that can save and load data efficiently.
Introduction to C++ File Handling
Welcome to the course "Introduction to C++ File Handling"! In this course, you will learn how to read and write from text files using the C++ programming language. This essential skill will empower you to work with external files, store data, and retrieve information efficiently.
Before diving into the practical aspects of file handling, let's cover some background information. C++ Builder is a full-featured IDE that facilitates rapid app development for various platforms, connecting to numerous databases effortlessly. Leveraging powerful frameworks like the VCL framework for native Windows apps and the Fire Monkey framework for cross-platform UIs, C++ Builder simplifies the development process.
To begin working with text files in C++, we'll include the "fstream" library and utilize the "fstream" data type to interact with files. From opening and closing files to reading and writing data, you'll comprehensively explore the file handling functionalities.
Now, here's a question to spark your curiosity: How can you decipher a secret message stored in a text file using a C++ program? This course will equip you with the knowledge and skills to solve such tasks.
Get ready to embark on a journey of mastering file handling in C++! We look forward to having you on board as we explore the intricacies of reading and writing data from text files. Let's dive in and start coding!
Main Concepts of File Handling in C++
-
Include fstream Library: In order to read and write from text files using C++, you need to include the fstream library, which allows you to work with files similar to how you use
iostream
for input and output to the console. -
Declare and Use fstream Variable: After including the fstream library, you need to declare a variable of type
fstream
to work with files. This variable will be used to open, close, read, and write from files. -
Writing to a Text File: To write to a text file, first, you need to open the file using the
open
function with the filename and the mode (ios::out
) specified. Then, you can use the<<
operator with the fstream variable to write text to the file. Finally, close the file using theclose
function. -
Appending to a Text File: If you want to append new text to an existing file, you need to open the file in append mode (
ios::app
). This will add the new text to the end of the file without overwriting the existing content. -
Reading from a Text File: To read from a text file, open the file in read mode using the
open
function with the filename and read mode (ios::in
). Use a while loop with thegetline
function to read each line of text from the file and store it in a string variable. Output the text to the console usingcout
. -
Tasks for Practice:
-
Task 1: Text Encryption: Combine knowledge of ASCII table and file handling to cipher text and write it to a file. Write a program to read the file, decipher the text, and display it in the console.
-
Task 2: Quiz Application: Develop a quiz application using structures and read questions and answers from a file instead of hardcoding them in the application. Write a program to read the questions and answers from the file and display them for the quiz application.
-
By understanding these main concepts and practicing the provided tasks, learners can enhance their skills in file handling with C++ programming language.
Practical Applications of Reading and Writing from Text Files Using C++
Step-by-Step Guide:
-
Include the Fstream Library:
- Ensure you include the Fstream library in your C++ program to enable working with files. Use the following code snippet:
#include <fstream>
.
- Ensure you include the Fstream library in your C++ program to enable working with files. Use the following code snippet:
-
Declare a File Stream Variable:
- Declare a variable of type
fstream
to interact with files. Example:fstream myFile;
.
- Declare a variable of type
-
Writing to a Text File:
- Open the file using the
open
function with the file name and write mode.- Example:
myFile.open("filename.txt", ios::out)
.
- Example:
- Check if the file has been opened successfully using
if (myFile.is_open())
. - Write text to the file using the file stream variable with redirection.
- Example:
myFile << "Text to write";
.
- Example:
- Close the file after writing by using
myFile.close()
.
- Open the file using the
-
Appending to a Text File:
- To append data to an existing file, open it in append mode (
ios::app
).- Example:
myFile.open("filename.txt", ios::app)
.
- Example:
- Check if the file is open, append the text, and close the file.
- To append data to an existing file, open it in append mode (
-
Reading from a Text File:
- Open a file in read mode using
ios::in
in theopen
function. - Read each line from the file using a
while
loop andgetline
.- Example:
while (getline(myFile, line)) { // Read and process each line }
.
- Example:
- Output each line to the console using
cout
.
- Open a file in read mode using
Try it Out:
-
Task 1: Encrypt a Story Using the ASCII Table:
- Write a story in a text file, cipher it using ASCII values, store it in a new file.
- Write a program to decrypt the text back into letters and display on the console.
-
Task 2: Build a Quiz Application Using File Input:
- Create a quiz application where questions and answers are read from a file.
- Implement a program to read the quiz data from the file and present it to the user.
Practice and Share Your Code:
- Solve the tasks mentioned above to strengthen your skills.
- Share your code in the comments for feedback and learning from others.
Enjoy learning and exploring file operations in C++! If you have any questions or need further guidance, feel free to ask. Keep coding and never stop learning! 🚀
Test your Knowledge
Which class is used to write data to a file in C++?
Which class is used to write data to a file in C++?
Advanced Insights into Reading and Writing Text Files using C++
In the world of C++ programming, working with text files is a fundamental skill that opens up a realm of possibilities. Once you grasp the basics of including the fstream library, declaring fstream variables, and opening files in appropriate modes, you can delve into more advanced aspects of file manipulation.
Tips for Efficient File Handling:
-
Appending vs. Overriding: Understand the difference between writing to a file in "write" mode (overriding existing content) and "append" mode (adding new content at the end). Utilize append mode to prevent overwriting valuable data.
-
Error Handling: Always check if a file has been successfully opened before reading from or writing to it. This ensures that your program operates smoothly without unexpected errors.
-
Reading Line by Line: Utilize the
getline()
function to read text files line by line, allowing you to process each piece of information individually. This method is particularly useful for handling structured data within files.
Recommendations for Practice:
-
Challenge Task 1: Combine ASCII ciphering with file handling by creating a program that encodes text into numbers and stores it in a file. Then, develop a decoding program that retrieves the original text from the file. This task enhances your understanding of both concepts in a creative way.
-
Challenge Task 2: Extend your knowledge of structures by implementing a quiz application that retrieves questions and answers from a file. This task reinforces your understanding of reading structured data from files and integrating it into your C++ programs.
Expert Advice:
- Debugging Techniques: Embrace the learning process, as making mistakes is a natural part of growth. Use debugging tools to identify and resolve issues efficiently. Learning from errors enhances your problem-solving skills and deepens your understanding of file handling concepts.
Curiosity Question:
How can you optimize file reading and writing operations in C++ to enhance the efficiency and performance of your programs? Dive deeper into techniques like buffering, asynchronous file operations, and memory mapping to uncover advanced strategies for file handling.
Exploring these advanced insights will not only sharpen your skills in file manipulation but also broaden your capabilities as a proficient C++ programmer. Keep practicing, experimenting, and pushing the boundaries of your knowledge to excel in this dynamic field.
By incorporating these advanced insights and recommendations into your C++ journey, you can elevate your expertise in reading and writing text files while honing your programming skills to new heights. Happy coding!
Additional Resources for File Handling in C++
- C++ File Handling Documentation: A comprehensive guide on file handling in C++ from cplusplus.com.
- File I/O in C++: An article on file input/output operations in C++ from GeeksforGeeks.
- C++ Filestream Tutorial: A tutorial on file handling using filestreams in C++ from Tutorialspoint.
- Advanced File Handling in C++: Learn about advanced file handling techniques in C++ with this educational resource from educba.
Explore these resources to enhance your understanding of file handling in C++ and deepen your knowledge on the topic. Happy coding!
Practice
Task
Task: Create a program that reads a file and counts the number of words in it.
Task: Write a program that takes a list of integers and writes them to a binary file.
Task: Implement a simple logging system that appends logs to a file with timestamps.
Task: Develop a program that reads a CSV file and stores the data in an array of structures.
Task: Create a backup program that copies the contents of one file to another.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
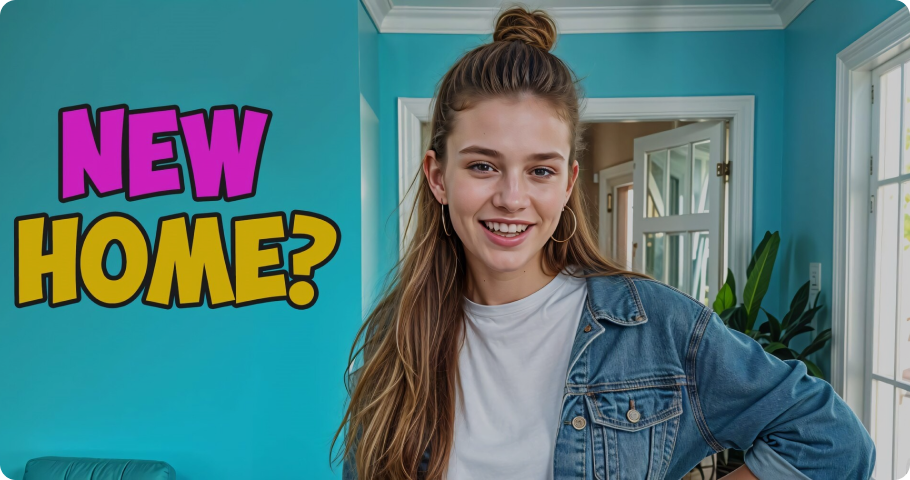
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.