Basic Syntax and Data Types
C++ is a versatile programming language, combining features of both high-level and low-level languages. Initially created by Bjarne Stroustrup in 1979, it has become one of the most widely used programming languages. C++ is particularly well-suited for developing software that requires high performance, such as operating systems, game engines, and real-time systems. The language supports object-oriented, procedural, and functional programming paradigms, offering developers a powerful toolset for various types of applications.
Lets Go!
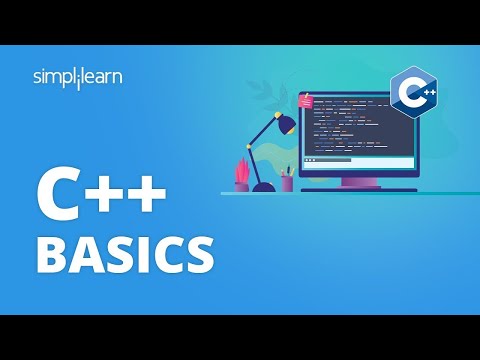
Basic Syntax and Data Types
Lesson 2
Understand the concept of variables, data types, and how they are used in C++ programming.
Get Started 🍁Welcome to Introduction to C++ Programming
Overview:
Welcome to this course on Introduction to C++ Programming! In this course, we will cover the basics of C++, starting from writing your first program in C++, understanding different types and variables, exploring concepts like arrays, strings, if-else statements, loops, and functions. Whether you are a beginner or looking to refresh your C++ skills, this course will provide you with a solid foundation in C++ programming.
Background Information:
C++ is a popular programming language introduced by John Stroustrup in 1979. Initially named 'C with Classes,' it later evolved into C++. This versatile language combines features of both high-level and low-level languages, making it suitable for various programming needs. C++ supports object-oriented, procedural, and functional programming paradigms, offering flexibility and efficiency in software development.
Setup and Prerequisites:
Before we dive into the course, ensure you have a code editor like VS Code set up for practice sessions. Familiarity with basic programming concepts and syntax will be beneficial, but this course is designed to be beginner-friendly, so no prior experience with C++ is required.
Curiosity Question:
Are you ready to write your first C++ program and unlock the power of this dynamic and efficient programming language?
Let’s embark on this learning journey together and explore the world of C++ programming! 🚀
Main Concepts of C++ Basics
-
Introduction to C++ Programming:
- C++ is a programming language introduced in 1979 by Bjarne Stroustrup.
- Originally called C with classes, later renamed C++.
- General-purpose, case-sensitive language.
- Features of object-oriented, procedural, and functional programming.
-
Writing Your First C++ Program:
- Begin with a simple 'Hello World' program.
- Includes header files, main function, and output using
cout
. - Syntax:
include <iostream>
,using namespace std
,int main()
,cout << 'Hello, World!'
,return 0
.
-
Types and Variables:
- Boolean, character, integer, and float data types.
- Variables are used to store values.
- Syntax:
data_type variable_name
.
-
Arrays:
- Collections of similar elements stored in contiguous memory.
- Allows storing multiple values of the same data type.
- Syntax:
data_type array_name[number_of_elements]
.
-
Strings:
- Collection of characters used to represent text.
- Two ways to create strings: C-style strings and string objects.
- Syntax:
string str = 'text'
.
-
If-Else Statements:
- Conditional statements used to run code based on conditions.
- Executes block of code inside
if
statement only if the condition is true. - Syntax:
if (condition) { code_block } else { code_block }
.
-
Loops:
- For Loop:
- Repeats a block of code for a fixed number of times.
- Initialization, condition, and updation parts.
- Syntax:
for (initialization; condition; updation) { code_block }
.
- While Loop:
- Repeats statements till a given condition is true.
- Syntax:
while (condition) { code_block }
.
- For Loop:
-
Functions:
- Group of statements designed to perform a specific task.
- Allows writing reusable code by defining and calling functions.
- Syntax:
return_type function_name(parameters) { code_block }
.
Understanding these main concepts will lay a strong foundation for learning C++ programming. Practice examples and experiment with different scenarios to reinforce your understanding.
Practical Applications of C++ Basics
Step-by-Step Guide:
-
Creating Your First Program: Hello World
- Open your preferred code editor (e.g., VS Code).
- Name the file
hello.cpp
. - Include the necessary header files:
#include <iostream>
. - Use
using namespace std
to access elements from the standard library. - Write the
main
function:int main() { cout << 'Hello World' << endl; return 0; }
- Compile and run the program to see 'Hello World' printed.
-
Understanding Types and Variables
- Learn about different data types in C++, such as boolean, character, integer, and float.
- Define variables using the syntax:
<data type> <variable name>
. - Practice creating variables to store various types of data.
-
Working with Arrays
- Declare and initialize arrays to store multiple elements of the same data type.
- Access elements in the array using index values.
- Experiment with array operations like finding the sum or average of elements.
-
Manipulating Strings
- Define strings in C++ using either C-style strings or string objects.
- Perform string operations such as concatenation, finding length, and manipulating characters.
- Try creating and modifying strings in your programs.
-
Implementing Control Structures: If-Else and Loops
- Use if-else statements to execute code based on conditions.
- Practice implementing for loops to iterate over a range of values.
- Explore while loops for scenarios where the number of iterations is not predefined.
-
Utilizing Functions
- Define functions to encapsulate code for specific tasks.
- Pass arguments to functions to make them more versatile.
- Call functions from the
main
function to execute the enclosed code.
-
Hands-On Examples:
- Example 1: Find the number of even and odd elements in an array.
- Example 2: Print numbers from 1 to 20 using a while loop and a function.
- Example 3: Demonstrate pushback and popback operations on a string.
Interactive Task:
- Choose one of the examples provided (e.g., counting even/odd elements in an array).
- Write the code in your code editor following the steps outlined.
- Compile and run the program to observe the output.
- Experiment with modifying the code to test different scenarios.
- Share your experience or any challenges faced in the process.
Test your Knowledge
What is the primary purpose of C++ variables?
What is the primary purpose of C++ variables?
Advanced Insights into C++ Programming
In this advanced section, we will delve deeper into the concepts covered in the video transcript and provide additional insights into C++ programming.
Advanced Aspects:
- Memory Management: Understanding how memory is allocated and deallocated in C++ is crucial for efficient programming. Explore dynamic memory allocation, pointers, and memory leaks.
- Advanced Data Structures: Dive into complex data structures like linked lists, trees, and graphs. Learn how to implement and manipulate these data structures efficiently in C++.
- Templates and Generic Programming: Discover the power of templates in C++, allowing you to write generic algorithms that work with different data types. Understand template metaprogramming for advanced functionality.
- Exception Handling: Explore how exception handling works in C++ to handle runtime errors gracefully. Learn about try-catch blocks, throwing exceptions, and handling different types of exceptions.
Expert Advice:
- Practice Regularly: To master C++ programming, consistent practice is key. Work on coding challenges, projects, and exercises to strengthen your skills.
- Understand Performance Optimization: Learn about optimizing your code for faster execution by understanding algorithms, data structures, and compiler optimizations.
- Read Advanced C++ Books: Explore books on advanced C++ topics such as 'Effective Modern C++' by Scott Meyers and 'C++ Concurrency in Action' by Anthony Williams for in-depth knowledge.
Curiosity Question:
How can you utilize C++ template specialization to create customized behavior for specific data types? Explore the possibilities and advantages of template specialization in C++ programming.
Additional Resources for C++ Basics
- C++ Tutorial for Beginners: A comprehensive video tutorial covering the basics of C++ programming for beginners.
- C++ Programming Language: An in-depth guide to the C++ programming language on GeeksforGeeks.
- C++ Data Types: Learn about different data types in C++ and how to use them effectively.
- Arrays in C++: Understand the concept of arrays in C++ and how to work with them.
- Strings in C++: Explore the manipulation and operations of strings in C++ programming.
- For Loop in C++: Learn how to use the for loop in C++ for iterative tasks.
- While Loop in C++: Understand the while loop and its implementation in C++ programming.
- Functions in C++: Dive into the concept of functions in C++ and how to create and use them effectively.
Practice
Task
Task: Write a C++ program to find the sum of integers from 1 to 100 using a loop.
Task: Create a C++ program that checks if a number is even or odd.
Task: Write a C++ program to reverse a string.
Task: Implement a C++ program that counts the number of elements in an array.
Task: Write a C++ function that calculates the factorial of a number.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Learn Ruby Programming Online with Step-by-Step Video Tutorials
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Learn Java Programming Online with Step-by-Step Video Tutorials
Explore our Java Programming course online with engaging video tutorials and practical guides to boost your skills and knowledge.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Python Programming
Learn how to use Python for general programming, automation, and problem-solving.